可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
Is it possible to access a BuildConfig value from AndroidManifest.xml?
In my build.gradle file, I have:
defaultConfig {
applicationId "com.compagny.product"
minSdkVersion 16
targetSdkVersion 21
versionCode 1
versionName "1.0"
// Facebook app id
buildConfigField "long", "FACEBOOK_APP_ID", FACEBOOK_APP_ID
}
FACEBOOK_APP_ID
is defined in my gradle.properties files:
# Facebook identifier (app ID)
FACEBOOK_APP_ID=XXXXXXXXXX
To use Facebook connect in my app, I must add this line to my AndroidManifest.xml:
<meta-data android:name="com.facebook.sdk.ApplicationId" android:value="@string/applicationId"/>
I want to replace @string/applicationId
by the BuildConfig field FACEBOOK_APP_ID
defined in gradle, like this:
<meta-data android:name="com.facebook.sdk.ApplicationId" android:value="FACEBOOK_APP_ID"/>
Is that possible using BuildConfig? If not, how can I achieve this?
回答1:
Replace
buildConfigField "long", "FACEBOOK_APP_ID", FACEBOOK_APP_ID
with
resValue "string", "FACEBOOK_APP_ID", FACEBOOK_APP_ID
then rebuild your project (Android Studio -> Build -> Rebuild Project).
The two commands both produce generated values - consisting of Java constants in the first case, and Android resources in the second - during project builds, but the second method will generate a string
resource value that can be accessed using the @string/FACEBOOK_APP_ID
syntax. This means it can be used in the manifest as well as in code.
回答2:
Another way to access Gradle Build Config values from your AndroidManifest.xml is through placeholders like this:
android {
defaultConfig {
manifestPlaceholders = [ facebookAppId:"someId..."]
}
productFlavors {
flavor1 {
}
flavor2 {
manifestPlaceholders = [ facebookAppId:"anotherId..." ]
}
}
}
and then in your manifest:
<meta-data android:name="com.facebook.sdk.ApplicationId" android:value="${facebookAppId}"/>
See more details here: https://developer.android.com/studio/build/manifest-build-variables.html
(Old link just for reference: http://tools.android.com/tech-docs/new-build-system/user-guide/manifest-merger)
回答3:
note: when you use resValue
the value can accidentally be overridden by the strings resource file (e.g. for another language)
To get a true constant value that you can use in the manifest and in java-code, use both manifestPlaceholders
and buildConfigField
: e.g.
android {
defaultConfig {
def addConstant = {constantName, constantValue ->
manifestPlaceholders += [ (constantName):constantValue]
buildConfigField "String", "${constantName}", "\"${constantValue}\""
}
addConstant("FACEBOOK_APP_ID", "xxxxx")
}
access in the manifest file:
<meta-data android:name="com.facebook.sdk.ApplicationId" android:value="${FACEBOOK_APP_ID}"/>
from java:
BuildConfig.FACEBOOK_APP_ID
If the constant value needs to be buildType-specific, the helper addConstant
needs to be tweaked (to work with groovy closure semantics), e.g.,
buildTypes {
def addConstantTo = {target, constantName, constantValue ->
target.manifestPlaceholders += [ (constantName):constantValue]
target.buildConfigField "String", "${constantName}", "\"${constantValue}\""
}
debug {
addConstantTo(owner,"FACEBOOK_APP_ID", "xxxxx-debug")
}
release {
addConstantTo(owner,"FACEBOOK_APP_ID", "xxxxx-release")
}
回答4:
Access build.gradle properties in your manifest as in following example:
For example you have a property "applicationId" in your build.gradle and you want to access that in your AndroidManifest:
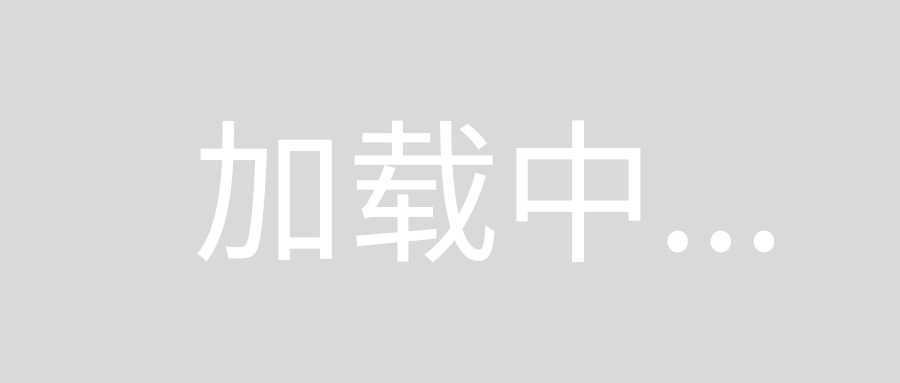
Access "applicationId" in AndroidManifest:
<receiver
android:name="com.google.android.gms.gcm.GcmReceiver"
android:exported="true"
android:permission="com.google.android.c2dm.permission.SEND">
<intent-filter>
<action android:name="com.google.android.c2dm.intent.RECEIVE" />
<category android:name="${applicationId}" />
</intent-filter>
</receiver>
Similarly, we can create string resources for other constants and access them in code files as simple as:
context.getString(R.string.GCM_SENDER_ID);
回答5:
Another option: use a different string resource file to replace all Flavor-dependent values:
Step 1:
Create a new folder in the "src" folder with the name of your flavor, im my case "stage"
Step 2:
Create resource files for all files that are dependent on the flavor
for example:
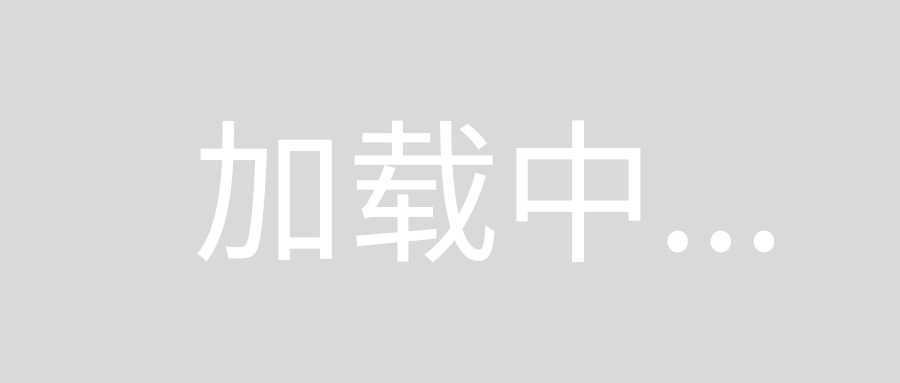
Step 3:
I am also using different icons, so you see the mipmap folders as well. For this quetion, only the "strings.xml" is important. Now you can overwrite all important string resources. You only need to include the ones you want to override, all others will be used from the main "strings.xml", it will show up in Android Studio like this:
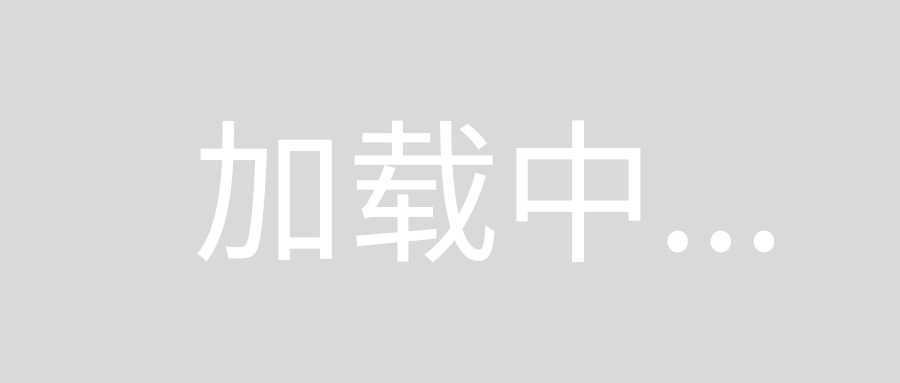
Step 4:
Use the string resources in your project and relax:
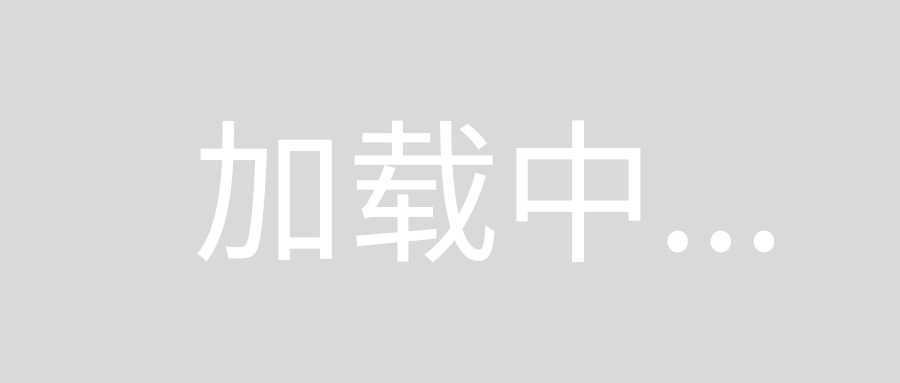
回答6:
@stkent is good but forgets to add that you need to rebuild your project afterwards
Replace
buildConfigField "long", "FACEBOOK_APP_ID", FACEBOOK_APP_ID
with
resValue "string", "FACEBOOK_APP_ID", FACEBOOK_APP_ID
then
Android Studio -> Build -> Rebuild Project
This will allow android generate the string resource accessible via
R.string.FACEBOOK_APP_ID