There is a link embedded in a web element in the Main Tab, I want to open that link in a new tab in the same window using Selenium Webdriver and python.
Perform some tasks in the new tab and then close that tab and return back to the main tab.
Manually we will do this by right-clicking on the link and then select "open in new tab" to open that link in new tab.
I am new to Selenium. I am using Selenium and BeautifulSoup to web scrape. I only know how to click on a link. I have read through many posts but couldn't find a proper answer
url = "https://www.website.com"
path = r'path_to_chrome_driver'
drive = webdriver.Chrome(path)
drive.implicitly_wait(30)
drive.get(url)
source = drie.page_source
py_button = driver.find_element_by_css_selector("div[data-res-position = '1']")
py_button.click()
I expect the link in div[data-res-position = '1']
to open in a new tab
This is trick can be achieve if your locator return a correct href
.
Try this first:
href = driver.find_element_by_css_selector("div[data-res-position = '1']").get_attribute("href")
print(href)
If you get correct href
, you can do:
#handle current tab
first_tab = driver.window_handles[0]
#open new tab with specific url
driver.execute_script("window.open('" +href +"');")
#hadle new tab
second_tab = driver.window_handles[1]
#switch to second tab
driver.switch_to.window(second_tab)
#switch to first tab
driver.switch_to.window(first_tab)
Hope this helps.
Following import:
selenium.webdriver.common.keys import Keys
You have to send key:
py_button.send_keys(Keys.CONTROL + 't')
As there is a link embedded within in the webelement in the Parent Tab, to open the link in a New Tab in the same window using Selenium and Python you can use the following solution:
To demonstrate the workflow the url https://www.google.com/
was opened in the Parent Tab and then open in new tab
functionalty is implemented through ActionChains
methods key_down()
, click()
and key_up()
methods.
Code Block:
from selenium import webdriver
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.common.by import By
from selenium.webdriver.support import expected_conditions as EC
from selenium.webdriver.common.action_chains import ActionChains
from selenium.webdriver.common.keys import Keys
import time
options = webdriver.ChromeOptions()
options.add_argument("start-maximized")
driver = webdriver.Chrome(options=options, executable_path=r'C:\WebDrivers\chromedriver.exe')
driver.get("https://www.google.com/")
link = WebDriverWait(driver, 20).until(EC.element_to_be_clickable((By.LINK_TEXT, "Gmail")))
ActionChains(driver).key_down(Keys.CONTROL).click(link).key_up(Keys.CONTROL).perform()
Note: You need to replace (By.LINK_TEXT, "Gmail")
with your desired locator e.g. ("div[data-res-position = '1']")
Browser Snapshot:
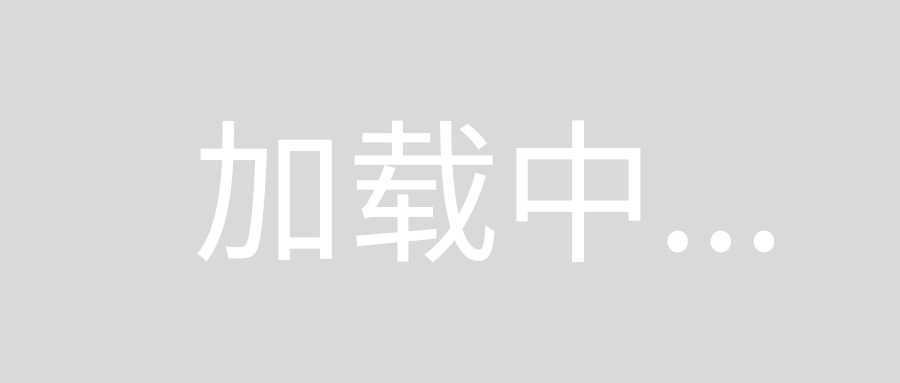
You can find a relevant Java based solution in Opening a new tab using Ctrl + click combination in Selenium Webdriver
Update
To shift Selenium's focus to the newly opened tab you can find a detailed discussion in Open web in new tab Selenium + Python