Here I want to set the already exist PDF document properties under Initial View tab in acrobat.
Document Options:
- Show = Bookmarks Panel and Page
- Page Layout = Continuous
- Magnification = Fit Width
- Open to Page number = 1
Window Options:
As show in below screen shot:
I am tried following code:
PdfStamper stamper = new PdfStamper(reader, new FileStream(dPDFFile, FileMode.Create));
stamper.AddViewerPreference(PdfName.DISPLAYDOCTITLE, new PdfBoolean(true));
the above code is used to set the document title show.
But following code are not working
For Page Layout:
stamper.AddViewerPreference(PdfName.PAGELAYOUT, new PdfName("OneColumn"));
For Bookmarks Panel and Page:
stamper.AddViewerPreference(PdfName. PageMode, new PdfName("UseOutlines"));
So please give guide me what is the correct way to meet my requirement.
I'm adding an extra answer in answer to the extra question in the comments of the previous answer:
When you have a PdfWriter
instance named writer
, you can set the Viewer preferences like this:
writer.ViewerPreferences = viewerpreference;
In this case, the viewerpreference
is a value that can have one of the following values:
PdfWriter.PageLayoutSinglePage
PdfWriter.PageLayoutOneColumn
PdfWriter.PageLayoutTwoColumnLeft
PdfWriter.PageLayoutTwoColumnRight
PdfWriter.PageLayoutTwoPageLeft
PdfWriter.PageLayoutTwoPageRight
See the PageLayoutExample for more info.
You can also change the page mode as is shown in the ViewerPreferencesExample. In which case the different values are "OR"-ed:
PdfWriter.PageModeFullScreen
PdfWriter.PageModeUseThumbs
PdfWriter.PageLayoutTwoColumnRight | PdfWriter.PageModeUseThumbs
PdfWriter.PageModeFullScreen | PdfWriter.NonFullScreenPageModeUseOutlines
PdfWriter.FitWindow | PdfWriter.HideToolbar
PdfWriter.HideWindowUI
Currently, you've only used the PrintPreferences example from the official documentation:
writer.AddViewerPreference(PdfName.PRINTSCALING, PdfName.NONE);
writer.AddViewerPreference(PdfName.NUMCOPIES, new PdfNumber(3));
writer.AddViewerPreference(PdfName.PICKTRAYBYPDFSIZE, PdfBoolean.PDFTRUE);
But in some cases, it's just easier to use:
writer.ViewerPreferences = viewerpreference;
Note that the official documentation is the book "iText in Action - Second Edition." The examples are written in Java, but you can find the C# version here. There is a new book in the works called "The ABC of PDF", but so far only 4 chapters were written. You'll find more info here: http://itextpdf.com/learn
The part about the different options to create a PdfDestination
is already present in "The ABC of PDF".
As for setting the language, this is done like this:
stamper.Writer.ExtraCatalog.Put(PdfName.LANG, new PdfString("EN"));
The result is shown in the following screen shot:
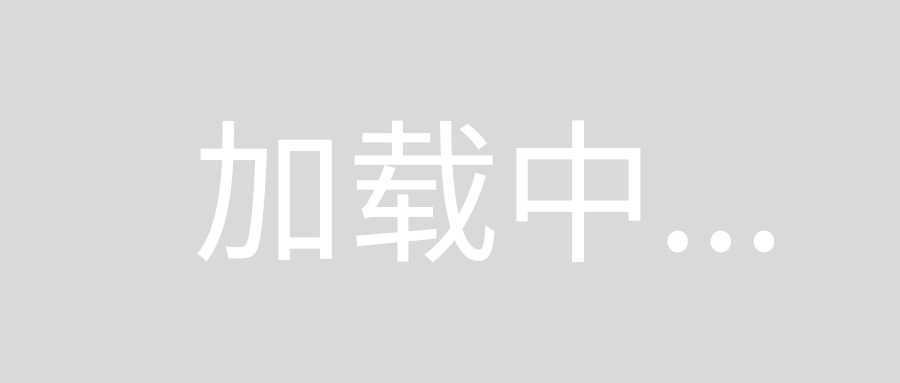
As you can see, there is now a Lang
entry with value EN
added to the catalog.
The two items Show = Bookmarks Panel and Page and Page Layout = Continuous are controlled one layer up from the ViewerPreferences
in the document's /Catalog
. You can get to this via:
stamper.Writer.ExtraCatalog
In your case you're looking for:
// Acrobat's Single Page
stamper.Writer.ExtraCatalog.Put(PdfName.PAGELAYOUT, PdfName.ONECOLUMN);
// Show bookmarks
stamper.Writer.ExtraCatalog.Put(PdfName.PAGEMODE, PdfName.USEOUTLINES);
The items Magnification = Fit Width and Open to Page number = 1 are also part of the /Catalog
but in a special key called /OpenAction
. You can set this using:
stamper.Writer.SetOpenAction();
In your case you're looking for:
//Create a destination that fit's width (fit horizontal)
var D = new PdfDestination(PdfDestination.FITH);
//Create an open action that points to a specific page using this destination
var OA = PdfAction.GotoLocalPage(1, D, stamper.Writer);
//Set the open action on the writer
stamper.Writer.SetOpenAction(OA);