可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
I am writing a simple guessing game program where the user will input a number to try and guess a randomly generated number.
If they get the number right I want to give them the option to play again.
Here is my code:
public class GuessingGame {
private Random num = new Random();
private int answer = num.nextInt(10);
private int guess;
private String playAgain;
public void inputGuess(){
System.out.println("Enter a number between 1 and 10 as your first guess: ");
Scanner input = new Scanner(System.in);
guess = input.nextInt();
do{
if (guess < 1 || guess > 10){
System.out.println("That is not a valid entry. Please try again: ");
guess = input.nextInt();
}else if (guess > answer){
System.out.println("Too high, Try Again: ");
guess = input.nextInt();
}else if (guess < answer){
System.out.println("Too low, Try Again: ");
guess = input.nextInt();
}
}while (guess != answer);
System.out.println("Congratulations, You guessed the number!");
System.out.println("Would you like to play again? Enter Y to play or any other key to quit: ");
playAgain = input.nextLine();
if(playAgain == "Y" || playAgain == "y"){
System.out.println("Enter a number between 1 and 10 as your first guess: ");
guess = input.nextInt();
}
}
}
The game plays through but when the user is prompted to play again nothing happens?
Any suggestions?
回答1:
Here is the completed code, fully working and tested... without using recursion.. and everything fixed.
public static void main(String[] args)
{
String playAgain = "";
Scanner scan = new Scanner(System.in);
do
{
ClassName.inputGuess();
System.out.println("Would you like to play again? Enter Y to play or any other key to quit: ");
playAgain = scan.nextLine();
}
while(playAgain.equalsIgnoreCase("Y"));
System.out.println("Thanks for playing!");
}
public void inputGuess()
{
Random num = new Random();
int answer = num.nextInt(10)+1;
Scanner input = new Scanner(System.in);
int guess;
System.out.println("Enter a number between 1 and 10 as your first guess: ");
guess = input.nextInt();
do
{
if (guess < 1 || guess > 10)
{
System.out.println("That is not a valid entry. Please try again: ");
guess = input.nextInt();
}
else
if (guess > answer)
{
System.out.println("Too high, Try Again: ");
guess = input.nextInt();
}
else
if (guess < answer)
{
System.out.println("Too low, Try Again: ");
guess = input.nextInt();
}
input.nextLine();
}
while (guess != answer);
System.out.println("Congratulations, You guessed the number!");
}
回答2:
Do the following and your code will work :
- Replace all
input.nextInt();
with Integer.parseInt(input.nextLine());
- Replace
(playAgain == "Y" || playAgain == "y")
with (playAgain.equalsIgnoreCase("Y"))
- Initialise
answer
inside inputGuess()
in starting instead.
- Replace the body of
if(playAgain.equalIgnoreCase("Y"))
with inputGuess();
When you enter integer value through console it also contain a \n
(next line) in it. But when you use nextInt()
, it doesn't read this \n
, but then when you tried to get next line with input.nextLine()
, it looks for \n
(next line) which is already there from integer entry and having nothing after that. Code look for "Y" or "y" and breaks because it doesn't found any of them.
That is why Integer.parseInt(input.nextLine());
works here
回答3:
Here is the code:
private Random num = new Random();
private int answer = num.nextInt(10) +1;
private int guess;
private String playAgain;
Scanner input = new Scanner(System.in);
public void inputGuess(){
System.out.println("Enter a number between 1 and 10 as your first guess: ");
guess = input.nextInt();
do{
if (guess < 1 || guess > 10){
System.out.println("That is not a valid entry. Please try again: ");
guess = input.nextInt();
}else if (guess > answer){
System.out.println("Too high, Try Again: ");
guess = input.nextInt();
}else if (guess < answer){
System.out.println("Too low, Try Again: ");
guess = input.nextInt();
}
if(guess == answer) {
System.out.println("Congratulations, You guessed the number!");
System.out.println("Would you like to play again? Enter Y to play or any other key to quit: ");
playAgain = input.nextLine();
}
}while (!playAgain.equals("Y") && !playAgain.equals("y"));
}
You just need to introduce the winning/losing logic inside the while, and the condition will be the ending/continue flag.
Another thing is always remember when comparing strings to use the equals method, since the == will compare the object reference and not the String value, in some cases == will return true for equal string since how JVM stores the Strings, but to be sure always use equals.
回答4:
Try something like this:
public void inputGuess(){
System.out.println("Enter a number between 1 and 10 as your first guess: ");
Scanner input = new Scanner(System.in);
guess = input.nextInt();
playAgain = "Y";
do{
if (guess < 1 || guess > 10){
System.out.println("That is not a valid entry. Please try again: ");
guess = input.nextInt();
}else if (guess > answer){
System.out.println("Too high, Try Again: ");
guess = input.nextInt();
}else if (guess < answer){
System.out.println("Too low, Try Again: ");
guess = input.nextInt();
}
if(guess == answer)
{
System.out.println("Congratulations, You guessed the number!");
System.out.println("Would you like to play again? Enter Y to play or N to quit: ");
input.nextLine();
playAgain = input.next();
answer = num.nextInt(10);
guess = -1;
if(!playAgain.equalsIgnoreCase("N"))
{
System.out.println("Enter a number between 1 and 10 as your first guess: ");
guess = input.nextInt();
}
}
}while (!playAgain.equalsIgnoreCase("N"));
}
You need your code for checking if they want to play again inside the loop. This way you wait until they have guessed the number correctly then ask if they want to play again. If they do you restart the process if they don't you exit the loop.
回答5:
Some of the solution I see above aren't correct. The random number, you need to add 1 to get between 1 and 10, also you need to compare with equals. I use case insensitive here.
The following code works as you need it.
import java.util.Random;
import java.util.Scanner;
public class Test2 {
private static Random num = new Random();
private static int answer = 0;
private static int guess;
private static String playAgain;
public static void main(String[] args) {
inputGuess();
}
// Guess Method.
public static void inputGuess(){
// create answer.
answer = 1+ num.nextInt(10);
System.out.println("Enter a number between 1 and 10 as your first guess: ");
Scanner input = new Scanner(System.in);
guess = input.nextInt();
do{
if (guess < 1 || guess > 10){
System.out.println("That is not a valid entry. Please try again: ");
guess = input.nextInt();
}else if (guess > answer){
System.out.println("Too high, Try Again: ");
guess = input.nextInt();
}else if (guess < answer){
System.out.println("Too low, Try Again: ");
guess = input.nextInt();
}
}while (guess != answer);
System.out.println("Congratulations, You guessed the number!");
System.out.println("Would you like to play again? Enter Y to play or any other key to quit: ");
playAgain = input.nextLine();
if( playAgain.equalsIgnoreCase("Y") ){
inputGuess();
}
}
}
回答6:
By now, you would have already guessed the right way to do it. Here is how I will approach it
public class Test {
public static void main (String...a) {
inputGuess();
}
public static void inputGuess() {
Scanner input = new Scanner(System.in);
String playAgain = "Y";
int guess;
Random ran = new Random();
int answer = ran.nextInt(10) + 1;
while (playAgain.equalsIgnoreCase("Y")) {
System.out.println("Enter a number between 1 and 10 as your first guess: " + answer);
guess = input.nextInt();
do {
if (guess < 1 || guess > 10) {
System.out.println("That is not a valid entry. Please try again: ");
guess = input.nextInt();
} else if (guess > answer) {
System.out.println("Too high, Try Again: ");
guess = input.nextInt();
} else if (guess < answer) {
System.out.println("Too low, Try Again: ");
guess = input.nextInt();
}
} while (guess != answer);
System.out.println("Congratulations, You guessed the number!");
System.out.println("Would you like to play again? Enter Y to play or any other key to quit: ");
input.nextLine();
playAgain = input.nextLine();
answer = ran.nextInt(10) + 1
}
}
}
回答7:
This code can serve your purpose...
import java.util.Random;
import java.util.Scanner;
public class GuessingGame
{
private Random num = new Random();
private int answer ;
private int guess;
private String playAgain;
public void inputGuess()
{
answer = num.nextInt(11);
System.out.println("Enter a number between 1 and 10 as your first guess: ");
Scanner input = new Scanner(System.in);
guess = input.nextInt();
do {
if (guess < 1 || guess > 10) {
System.out
.println("That is not a valid entry. Please try again: ");
guess = input.nextInt();
} else if (guess > answer) {
System.out.println("Too high, Try Again: ");
guess = input.nextInt();
} else if (guess < answer) {
System.out.println("Too low, Try Again: ");
guess = input.nextInt();
}
} while (guess != answer);
System.out.println("Congratulations, You guessed the number!");
System.out.println("Would you like to play again? Enter Y to play or any other key to quit: ");
do
{
playAgain = input.nextLine();
}while(playAgain.length()<1);
if (playAgain.trim().equalsIgnoreCase("y"))
{
inputGuess();
}
else
{
System.out.println("Good Bye!!!");
}
}
public static void main(String[] args) {
new GuessingGame().inputGuess();
}
}
回答8:
one issue :
Do not compare the content of two strings by ==
which just
you should use equals()
Imagine the right answer is one in this case
Follow this as your blue print sample
int answer = 0;
String yes = "";
Scanner input = new Scanner(System.in);
do{
do{
System.out.println("Enter your number");
answer = input.nextInt();
} while ( answer != 1);
System.out.println("Would you like to play again?");
yes = input.next();
} while ( yes.equalsIgnoreCase("yes"));
output:
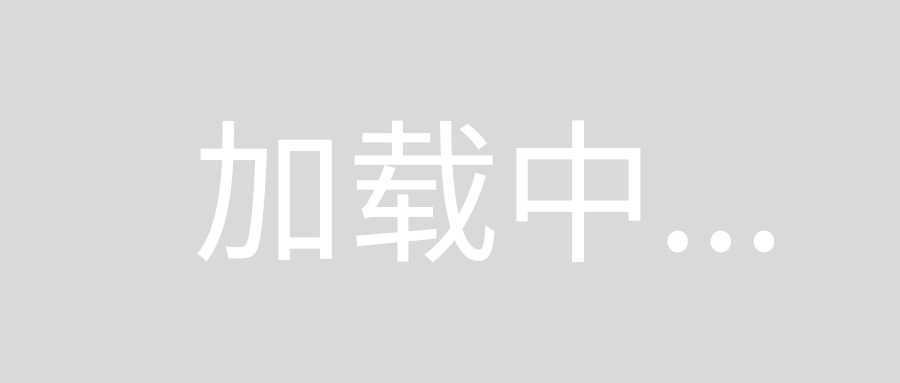