This question already has answers here:
Closed 3 years ago.
I have the following code:
try
{
using (var myHttpWebResponse = (HttpWebResponse) httPrequestCreated.GetResponse())
{
var streamResponse = myHttpWebResponse.GetResponseStream();
if (streamResponse != null)
{
var streamRead = new StreamReader(streamResponse);
var readBuff = new Char[256];
var count = streamRead.Read(readBuff, 0, 256);
while (count > 0)
{
var outputData = new String(readBuff, 0, count);
finalResopnse += outputData;
count = streamRead.Read(readBuff, 0, 256);
}
streamRead.Close();
streamResponse.Close();
myHttpWebResponse.Close();
}
}
}
catch (WebException ex)
{
MessageBox.Show("something went wrong");
}
The error code is 404 Not Found
, but instead of a MessageBox I get the following error:
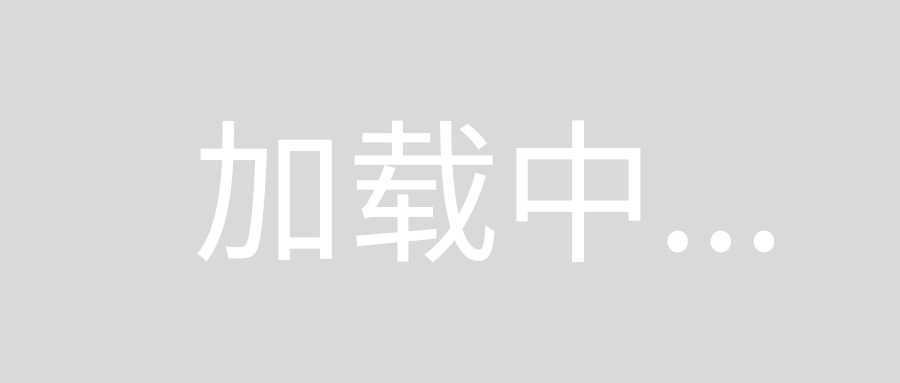
Why is the exception never caught?
You probably have first chance exception catching turned on in Visual Studio.
Try running the application without debugger (Ctrl+F5). Or, if you get this dialog, you can press Run (F5) to get your message box.
Are you sure you are 'catching' the same type of Exception? Instead of WebException
catch just the Exception
and see if you get the MessageBox
try
{
using (var myHttpWebResponse = (HttpWebResponse) httPrequestCreated.GetResponse())
{
var streamResponse = myHttpWebResponse.GetResponseStream();
if (streamResponse != null)
{
var streamRead = new StreamReader(streamResponse);
var readBuff = new Char[256];
var count = streamRead.Read(readBuff, 0, 256);
while (count > 0)
{
var outputData = new String(readBuff, 0, count);
finalResopnse += outputData;
count = streamRead.Read(readBuff, 0, 256);
}
streamRead.Close();
streamResponse.Close();
myHttpWebResponse.Close();
}
}
}
catch (Exception ex)
{
MessageBox.Show(string.format("this went wrong: {0}", ex.Message));
}
Edit: Watching you pic closely I think you are seeing the Exception before is being thrown to your catch block. On your VS hit Ctrl+Alt+E and make sure the Throw
check of Common Language Runtime Exceptions
is unchecked