I'd like to build a navigation drawer where each item has a different selection color (the icon tint and text color) as the google play store has:
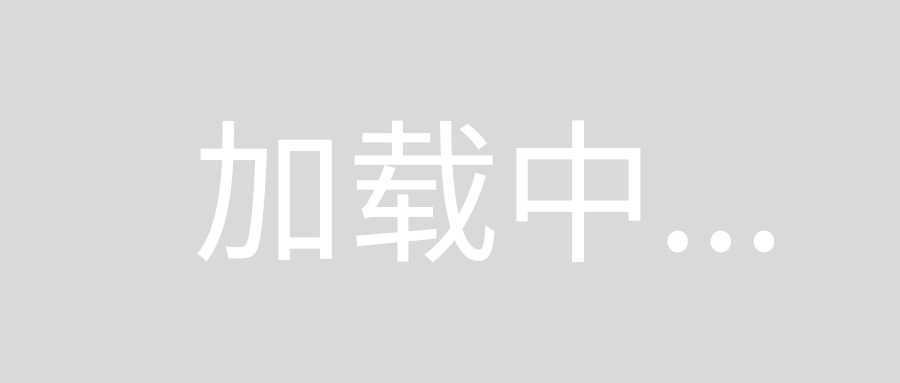
I'm not sure how they've solved this, I think they use different activities with different drawers. I want to use fragments and I want to change the icon tint and text color. Any ideas how I can do this? I'm using google's design support library and a drawer layout with a navigation view in there.
use app:itemIconTint
in your NavigationView
for icons and use app:itemTextColor
for textColors
Sample :
drawable/navigation_text_color
:
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<!-- This is used when the Navigation Item is checked -->
<item android:color="#009688" android:state_checked="true" />
<!-- This is the default text color -->
<item android:color="#E91E63" />
</selector>
and layout
:
<android.support.design.widget.NavigationView
.
.
app:itemTextColor="@drawable/navigation_text_color"/>
If by dynamically you mean programmatically you could try this:
// FOR NAVIGATION VIEW ITEM TEXT COLOR
int[][] states = new int[][]{
new int[]{-android.R.attr.state_checked}, // unchecked
new int[]{android.R.attr.state_checked}, // checked
new int[]{} // default
};
// Fill in color corresponding to state defined in state
int[] colors = new int[]{
Color.parseColor("#747474"),
Color.parseColor("#007f42"),
Color.parseColor("#747474"),
};
ColorStateList navigationViewColorStateList = new ColorStateList(states, colors);
// apply to text color
navigationView.setItemTextColor(navigationViewColorStateList);
// apply to icon color
navigationView.setItemIconTintList(navigationViewColorStateList);
So you could define multiple colors for different settings like Day or Night.
Finllay i got the answer,
You must change the colorAccent in the colors file to which ever color you want :
<color name="colorAccent">whichever color required</color>
This solution worked for me
<android.support.design.widget.NavigationView
.
.
app:itemIconTint="@color/your_selector_file_name" // for Icon
app:itemTextColor="@color/your_selector_file_name" // for text
/>
Adding to what @DAVOOD said. The below color selector worked for me.
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<!-- This is used when the Navigation Item is checked -->
<item android:color="@color/tenoBrandColor" android:state_pressed="true" />
<!-- This is the default text color -->
<item android:color="@color/textprimary" />
</selector>
There's a simpler option for the lazy ones out there.
TL;DR: Just create a new style
with the colorPrimary
being your desired color and then set the NavigationView
's theme
to be this new style
.
Just make a new style
as:
<style name="AppTheme.NoActionBar.NavigationView">
<item name="colorPrimary">@color/myDesiredColor</item>
</style>
This style
will automatically inherit from your base theme
(on my case it was AppTheme.NoActionBar
) and then you just have to set the colorPrimary
with your desired color.
Then you just have to set the NavigationView
's theme
as:
android:theme="@style/AppTheme.NoActionBar.NavigationView"
in:
<android.support.design.widget.NavigationView
android:id="@+id/nav_view"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_gravity="start"
android:fitsSystemWindows="true"
app:headerLayout="@layout/nav_header_menu_test"
app:menu="@menu/main_drawer"
android:theme="@style/AppTheme.NoActionBar.NavigationView" />