可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
I've defined some values in my appsettings.json
for things like database connection strings, webapi locations and the like which are different for development, staging and live environments.
Is there a way to have multiple appsettings.json
files (like appsettings.live.json
, etc, etc) and have the asp.net app just 'know' which one to use based on the build configuration it's running?
回答1:
You may use conditional compilation:
public Startup(IHostingEnvironment env)
{
var builder = new ConfigurationBuilder()
.SetBasePath(env.ContentRootPath)
.AddJsonFile("appsettings.json", optional: true, reloadOnChange: true)
#if SOME_BUILD_FLAG_A
.AddJsonFile($"appsettings.flag_a.json", optional: true)
#else
.AddJsonFile($"appsettings.no_flag_a.json", optional: true)
#endif
.AddEnvironmentVariables();
this.configuration = builder.Build();
}
回答2:
I have added screenshots of a working environment, because it cost me several hours of R&D.
First, add a key to your launch.json
file.
See the below screenshot, I have added Development
as my environment.
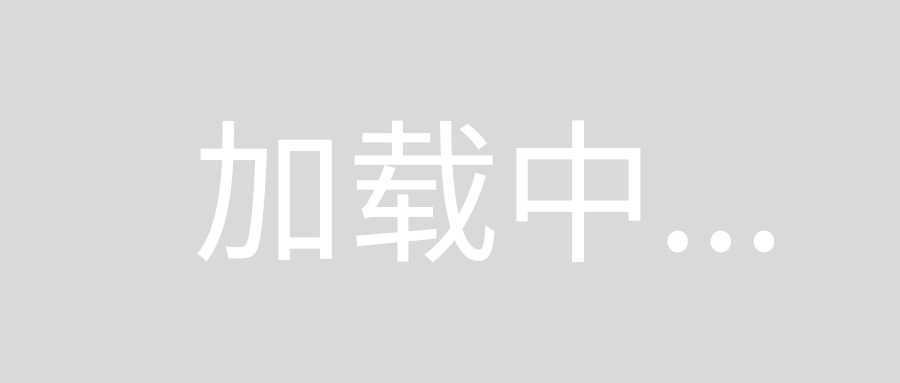
Then, in your project, create a new appsettings.{environment}.json
file that includes the name of the environment.
In the following screenshot, look for two different files with the names:
appsettings.Development.Json
appSetting.json
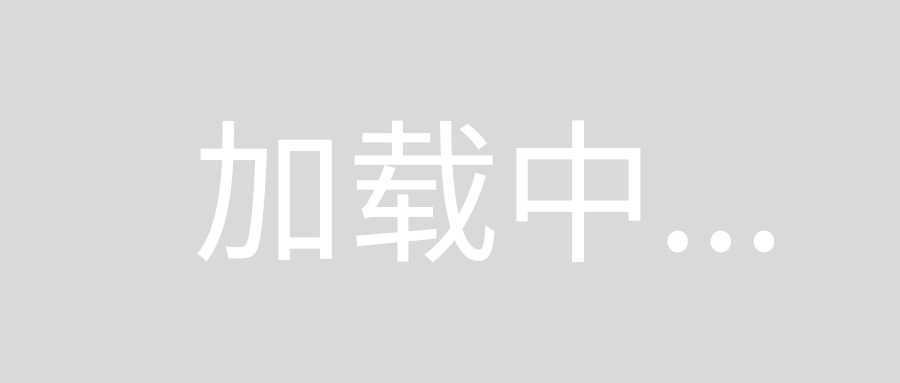
And finally, configure it to your StartUp
class, like below:
public Startup(IHostingEnvironment env)
{
var builder = new ConfigurationBuilder()
.SetBasePath(env.ContentRootPath)
.AddJsonFile("appsettings.json", optional: false, reloadOnChange: true)
.AddJsonFile($"appsettings.{env.EnvironmentName}.json", optional: true)
.AddEnvironmentVariables();
Configuration = builder.Build();
}
And at last I run it from the command line, like this:
dotnet run --environment "Development"
where "Development"
is the name of my environment.
回答3:
In ASP.NET Core you should rather use EnvironmentVariables instead of build configuration for proper appsettings.json
Right click on you project > Properties > Debug > Environment Variables
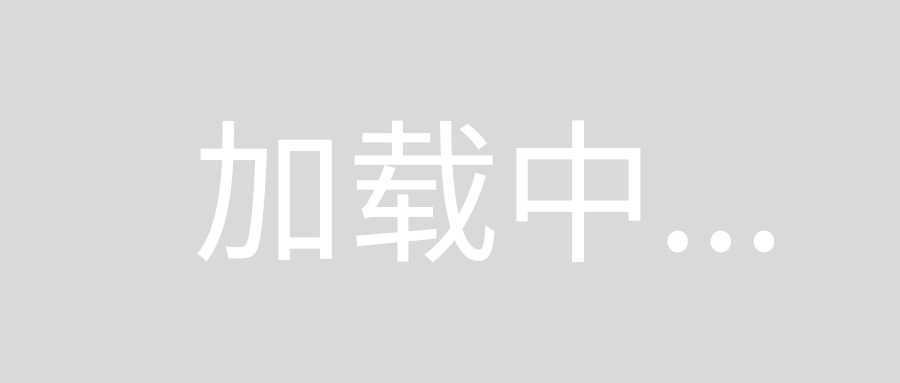
ASP.NET Core will take proper appsettings.json file.
Now you can use that Environment Variable like this:
public Startup(IHostingEnvironment env)
{
var builder = new ConfigurationBuilder()
.SetBasePath(env.ContentRootPath)
.AddJsonFile("appsettings.json", optional: true, reloadOnChange: true)
.AddJsonFile($"appsettings.{env.EnvironmentName}.json", optional: true)
.AddEnvironmentVariables();
Configuration = builder.Build();
}
If you do it @Dmitry 's way, you will have problems eg. when overriding appsettings.json values on Azure.
回答4:
Just an update for .NET core 2.0 users, you can specify application configuration after the call to CreateDefaultBuilder
:
public class Program
{
public static void Main(string[] args)
{
BuildWebHost(args).Run();
}
public static IWebHost BuildWebHost(string[] args) =>
WebHost.CreateDefaultBuilder(args)
.ConfigureAppConfiguration(ConfigConfiguration)
.UseStartup<Startup>()
.Build();
static void ConfigConfiguration(WebHostBuilderContext ctx, IConfigurationBuilder config)
{
config.SetBasePath(Directory.GetCurrentDirectory())
.AddJsonFile("config.json", optional: false, reloadOnChange: true)
.AddJsonFile($"config.{ctx.HostingEnvironment.EnvironmentName}.json", optional: true, reloadOnChange: true);
}
}
回答5:
You can make use of environment variables and the ConfigurationBuilder
class in your Startup
constructor like this:
public Startup(IHostingEnvironment env)
{
var builder = new ConfigurationBuilder()
.SetBasePath(env.ContentRootPath)
.AddJsonFile("appsettings.json", optional: true, reloadOnChange: true)
.AddJsonFile($"appsettings.{env.EnvironmentName}.json", optional: true)
.AddEnvironmentVariables();
this.configuration = builder.Build();
}
Then you create an appsettings.xxx.json
file for every environment you need, with "xxx" being the environment name. Note that you can put all global configuration values in your "normal" appsettings.json
file and only put the environment specific stuff into these new files.
Now you only need an environment variable called ASPNETCORE_ENVIRONMENT
with some specific environment value ("live", "staging", "production", whatever). You can specify this variable in your project settings for your development environment, and of course you need to set it in your staging and production environments also. The way you do it there depends on what kind of environment this is.
UPDATE: I just realized you want to choose the appsettings.xxx.json
based on your current build configuration. This cannot be achieved with my proposed solution and I don't know if there is a way to do this. The "environment variable" way, however, works and might as well be a good alternative to your approach.
回答6:
You can add the configuration name as the ASPNETCORE_ENVIRONMENT
in the launchSettings.json
as below
"iisSettings": {
"windowsAuthentication": false,
"anonymousAuthentication": true,
"iisExpress": {
"applicationUrl": "http://localhost:58446/",
"sslPort": 0
}
},
"profiles": {
"IIS Express": {
"commandName": "IISExpress",
"environmentVariables": {
ASPNETCORE_ENVIRONMENT": "$(Configuration)"
}
}
}
回答7:
1.Create multiple appSettings.$(Configuration).jsons like
appSettings.staging.json
appSettings.production.json
2.Create a pre-build event on the project which copies the respective file to appSettings.json like this
copy appSettings.$(Configuration).json appSettings.json
3.Use Only appSettings.json in your Config Builder
var builder = new ConfigurationBuilder()
.SetBasePath(env.ContentRootPath)
.AddJsonFile("appsettings.json", optional: false, reloadOnChange: true)
.AddEnvironmentVariables();
Configuration = builder.Build();