可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
I am using Leaflet 1.0.0-rc.2+e02b5c9. I know the default is rendering all markers, polylines ... from longitude -180 to 180 as screen shot here:
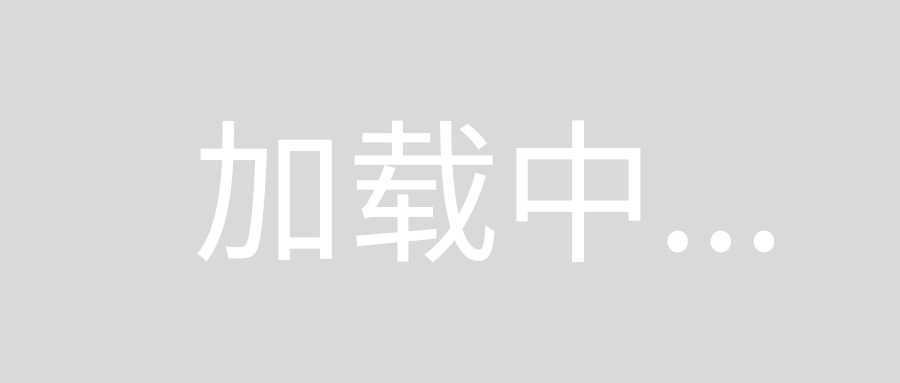
However, I want to show the map as this longitude I want to show at this point (It is middle sea between Japan and US):
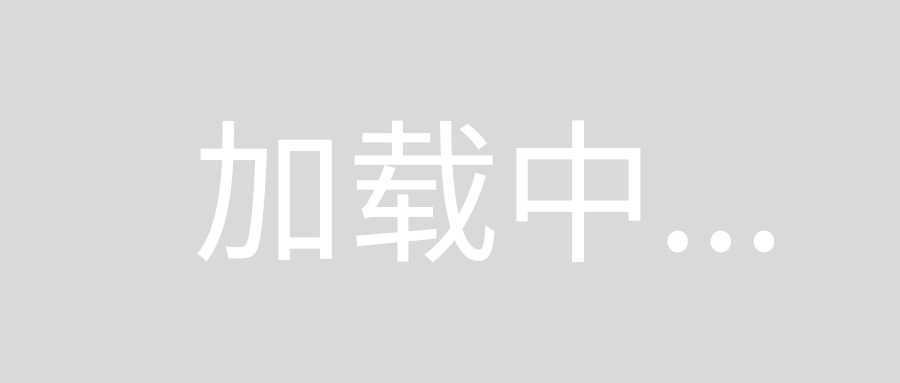
but you see all the markers are not rendered on the right side. Even though, If i set
worldCopyJump: true
when I drag to the right, all markers are appeared on the right but they are disappeared on the left and vice versa. Actually, I want they are appeared at the same time.
Any ideas to fix that??
回答1:
Just make sure that the longitudes of your markers are in the range 0..360
instead of in the range -180..180
. See a working example.
i.e. instead of
L.marker([0,170]).addTo(map);
L.marker([0,-180]).addTo(map);
L.marker([0,-170]).addTo(map);
Do something like
L.marker([0,170]).addTo(map);
L.marker([0,180]).addTo(map);
L.marker([0,190]).addTo(map);
In other words, if a longitude is smaller than zero, add 360 to it. You might want to use L.Util.wrapNum(lng, [0,360], true)
instead, if you plan to filter all your longitudes at once.
回答2:
A similar issue has been encountered and reported on the Leaflet Github
The solution is to increase the longitude of your markers if their initial longitude is below 0.
var totalMarkers = markerPositions.length;
for(var i = 0; i<totalMarkers; i++){
var mData = markerPositions[i];
if (mData.lon < 0) {
mData.lon += 360;
}
L.marker([mData.lat, mData.lon]).addTo(map);
}
回答3:
Thank you all for the help. Actually, I am not using your suggested codes but I got me the idea to fix it.
This is my solution, hope it helpful for others looking for same solution:
Screenshot that was solved
Always display the icon and its copy on the map (on longitude range you want, in my case, it is from 0 to 360)
makeMarkers: (item) ->
markers = []
markers.push(item.makeMarker())
copy_marker = item.makeMarker()
copy_marker.setLatLng( new L.LatLng(copy_marker._latlng.lat, copy_marker._latlng.lng + 360) )
markers.push(copy_marker)
markers
In Item class:
makeMarker: ->
LeafletIcon = L.Icon.extend(
options: {
iconSize: [25, 25],
iconAnchor: [10, 10],
popupAnchor: [0, 0]
},
)
icon = new LeafletIcon( {iconUrl: this.iconUrl} )
marker = L.marker([this.latitude, this.longitude], {icon: icon, zIndexOffset: 10})
marker.id = this.id
marker
Thank you again.
回答4:
Another approach is to leverage the Leaflet.RepeatedMarkers plugin, which will display a copy of each marker per 360 degrees of longitude:
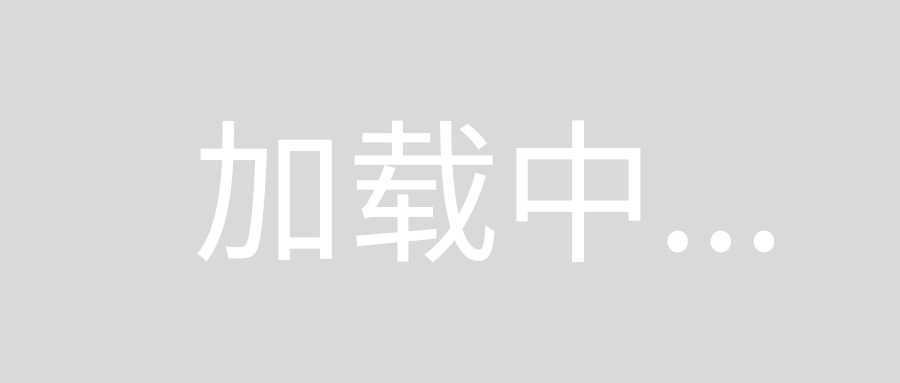
Applying this to markers near the antimeridian works as well, e.g.:
var myRepeatingMarkers = L.gridLayer.repeatedMarkers().addTo(map);
L.polyline([[-85,180],[85,180]]).addTo(map);
L.polyline([[-85,-180],[85,-180]]).addTo(map);
myRepeatingMarkers.addMarker(L.marker([0,140]));
myRepeatingMarkers.addMarker(L.marker([0,150]));
myRepeatingMarkers.addMarker(L.marker([0,160]));
myRepeatingMarkers.addMarker(L.marker([0,170]));
myRepeatingMarkers.addMarker(L.marker([0,180]));
myRepeatingMarkers.addMarker(L.marker([0,-170]));
myRepeatingMarkers.addMarker(L.marker([0,-160]));
myRepeatingMarkers.addMarker(L.marker([0,-150]));
myRepeatingMarkers.addMarker(L.marker([0,-140]));
will display something like:
Check out the live example for using Leaflet.repeatedMarkers with markers near the antimeridian.