i'm managing a date that comes from an Alfresco Properties and is in the specified (Tue Jul 13 00:00:00 CEST 2010) and i need to convert it to a Java date...i've looked around and found millions of posts for various string to date conversion form and also this page and so i tried something like this:
private static final DateFormat alfrescoDateFormat = new SimpleDateFormat("EEE MMM dd HH:mm:ss zzz yyyy");
Date dataRispostaDate = alfrescoDateFormat.parse(dataRisposta);
But it throws an exception.(The exception is (SSollevata un'eccezione durante la gestione della data: java.text.ParseException: Unparseable date: "Tue Jul 13 00:00:00 CEST 2011").
I post the complete code:
try {
QName currDocTypeQName = (QName) nodeService.getType(doc);
log.error("QName:["+currDocTypeQName.toString()+"]");
if (currDocTypeQName != null) {
String codAtto = AlfrescoConstants.getCodAttoFromQName(currDocTypeQName.toString());
log.error("codAtto:["+codAtto+"]");
if (codAtto.equals(AlfrescoConstants.COD_IQT)){
List<ChildAssociationRef> risposteAssociate = nodeService.getChildAssocs(doc, AlfrescoConstants.QN_RISPOSTEASSOCIATE, RegexQNamePattern.MATCH_ALL);
for (ChildAssociationRef childAssocRef : risposteAssociate) {
// Vado a prendere il nodo
NodeRef risposta = childAssocRef.getChildRef();
String dataRisposta = (nodeService.getProperty(risposta, AlfrescoConstants.QN_DATA_RISPOSTA)).toString();
log.error("dataRisposta:["+dataRisposta+"]");
if (!dataRisposta.isEmpty()){
try {
Date dataDa = dmyFormat.parse(req.getParameter("dataDa"));
log.error("dataDa:["+dataDa.toString()+"]");
Date dataA = dmyFormat.parse(req.getParameter("dataA"));
log.error("dataA:["+dataA.toString()+"]");
Date dataRispostaDate = alfrescoDateFormat.parse(dataRisposta);
log.error("dataRispostaDate:["+dataRispostaDate.toString()+"]");
if (dataRispostaDate.after(dataDa) && dataRispostaDate.before(dataA)){
results.add(doc);
log.error("La data risposta è compresa tra le date specificate");
}else{
log.error("La data risposta non è compresa tra le date specificate");
}
} catch (ParseException e) {
log.error("Sollevata un'eccezione durante la gestione della data: " + e);
throw new RuntimeException("Formato data non valido");
}
}else{
log.error("La data risposta non è specificata");
}
}
}else{
results.add(doc);
}
}
} catch (Exception e) {
log.error("Sollevata un'eccezione durante la gestione del codice atto nel webscript nicola: " + e);
}
Anyone can help?
Basically your problem is that you are using a SimpleDateFormat(String pattern) constructor, where javadoc says:
Constructs a SimpleDateFormat using
the given pattern and the default date
format symbols for the default locale.
And if you try using this code:
DateFormat osLocalizedDateFormat = new SimpleDateFormat("MMMM EEEE");
System.out.println(osLocalizedDateFormat.format(new Date()))
you will notice that it prints you month and day of the week titles based on your locale.
Solution to your problem is to override default Date locale using SimpleDateFormat(String pattern, Locale locale) constructor:
DateFormat dateFormat = new SimpleDateFormat(
"EEE MMM dd HH:mm:ss zzz yyyy", Locale.US);
dateFormat.parse("Tue Jul 13 00:00:00 CEST 2011");
System.out.println(dateFormat.format(new Date()));
Based on your comments, I believe that your property is actually of type d:date or d:datetime. If so, the property will already be coming back from Alfresco as a java Date object. So, all you'd need to do is:
NodeRef risposta = childAssocRef.getChildRef();
Date dataRisposta = (Date)nodeService.getProperty(risposta, AlfrescoConstants.QN_DATA_RISPOSTA);
tl;dr
ZonedDateTime.parse( // Produce a `java.time.ZonedDateTime` object.
"Wed Jul 13 00:00:00 CEST 2011" , // Corrected `Tue` to `Wed`.
DateTimeFormatter.ofPattern( "EEE MMM d HH:mm:ss zzz uuuu" , Locale.US )
)
2011-07-13T00:00+02:00[Europe/Paris]
Bad data: Wed
vs Tue
You input string Tue Jul 13 00:00:00 CEST 2011
is invalid. July 13 of 2011 was a Wednesday, not a Tuesday.
String input = "Wed Jul 13 00:00:00 CEST 2011" ; // Corrected `Tue` to `Wed`.
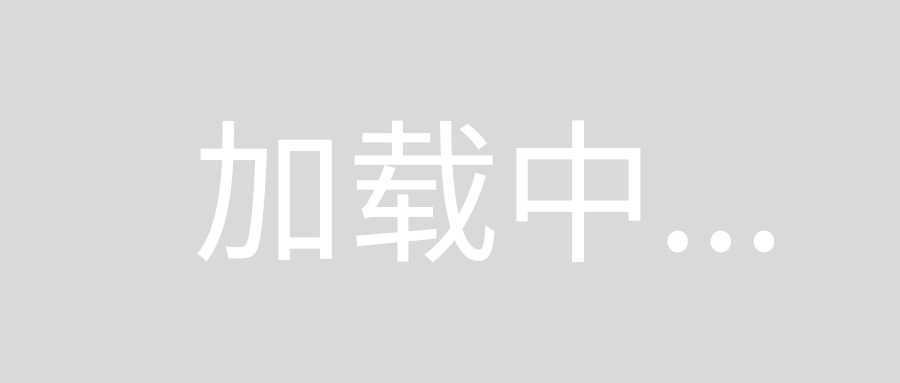
java.time
The modern approach uses the java.time classes rather than the troublesome old legacy date-time classes seen in other Answers.
Define a formatting pattern to match your input string. Notice the Locale
, which defines the human language to be used in parsing name of month and name of day-of-week.
DateTimeFormatter f = DateTimeFormatter.ofPattern( "EEE MMM d HH:mm:ss zzz uuuu" , Locale.US );
ZonedDateTime zdt = ZonedDateTime.parse( input , f );
zdt.toString(): 2011-07-13T00:00+02:00[Europe/Paris]
Time zone
Your CEST
is a pseudo-zone, not a true time zone. Never use these. They are not standardized, and are not even unique(!).
The ZonedDateTime
class will make a valiant effort at guessing the intention behind such a 3-4 character pseudo-zone. Your CEST
happened to work here, interpreted as Europe/Paris
time zone. But you cannot rely on the guess being 100% successful. Instead, avoid such pseudo-zones entirely.
Specify a proper time zone name in the format of continent/region
, such as America/Montreal
, Africa/Casablanca
, or Pacific/Auckland
.
ZoneId z = ZoneId.of( "Europe/Paris" ); // https://time.is/Paris
LocalDate today = LocalDate.now( z ); // Current date varies around the globe by zone.
ISO 8601
Your input string’s format is terrible. When serializing date-time values as text, use only the standard ISO 8601 formats.
The ZonedDateTime
class wisely extends the standard format by appending the name of the time zone in square brackets as seen in examples above.
About java.time
The java.time framework is built into Java 8 and later. These classes supplant the troublesome old legacy date-time classes such as java.util.Date
, Calendar
, & SimpleDateFormat
.
The Joda-Time project, now in maintenance mode, advises migration to the java.time classes.
To learn more, see the Oracle Tutorial. And search Stack Overflow for many examples and explanations. Specification is JSR 310.
Where to obtain the java.time classes?
- Java SE 8, Java SE 9, and later
- Built-in.
- Part of the standard Java API with a bundled implementation.
- Java 9 adds some minor features and fixes.
- Java SE 6 and Java SE 7
- Much of the java.time functionality is back-ported to Java 6 & 7 in ThreeTen-Backport.
- Android
- Later versions of Android bundle implementations of the java.time (JSR 310) classes.
- For earlier Android, the ThreeTenABP project adapts ThreeTen-Backport (mentioned above). See How to use ThreeTenABP….
The ThreeTen-Extra project extends java.time with additional classes. This project is a proving ground for possible future additions to java.time. You may find some useful classes here such as Interval
, YearWeek
, YearQuarter
, and more.
The problem is that CEST is not a timezone Java supports. You can use "CST".
The Javadoc for TimeZone notes:
Three-letter time zone IDs
For compatibility with JDK 1.1.x, some other three-letter time zone IDs (such as "PST", "CTT", "AST") are also supported. However, their use is deprecated because the same abbreviation is often used for multiple time zones (for example, "CST" could be U.S. "Central Standard Time" and "China Standard Time"), and the Java platform can then only recognize one of them.
For three/four letter timezone support I suggest you try JodaTime which may do a better job.
String dataRisposta = "Tue Jul 13 00:00:00 CST 2010";
Date dataRispostaDate = alfrescoDateFormat.parse(dataRisposta);
System.out.println(dataRispostaDate);
prints
Tue Jul 13 07:00:00 BST 2010
String[] ids = TimeZone.getAvailableIDs();
Arrays.sort(ids);
for (String id : ids) {
System.out.println(id);
}
prints
...
CAT
CET
CNT
CST
CST6CDT
CTT
...