I develop a website with Visual Studio 2010. I want to run a Fortran DLL. I used Intel Visual Fortran to create a .dll and to test how to use it. My code is:
SUBROUTINE SIMPSON (N,H,I)
!DEC$ ATTRIBUTES DLLEXPORT, DECORATE, ALIAS : "SIMPSON" :: SIMPSON
!DEC$ ATTRIBUTES REFERENCE::N
!DEC$ ATTRIBUTES REFERENCE::H
!DEC$ ATTRIBUTES REFERENCE::I
INTEGER N,H,I
I=N+H
RETURN
END
which practically takes two integers, adds them and return the result. Now I have the .dll I don't know how to run it with Visual Studio. Can anyone who knows please give me steps to follow?
I do this all the time. What I do, is in the calling project (C#
, VB.NET
) I add the .dll
output to the project as an existing item, with Add as Link
option. Then I set it to copy if newer in the project tree.
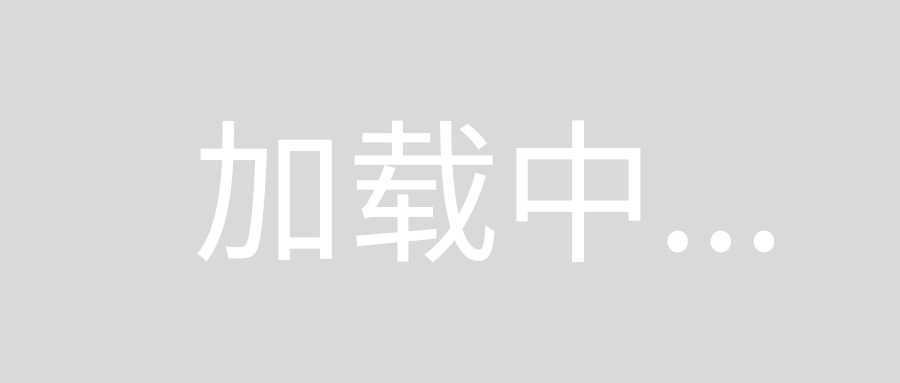
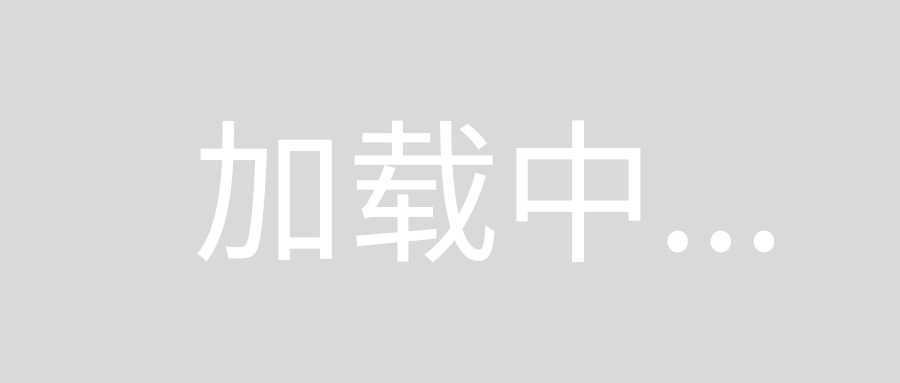
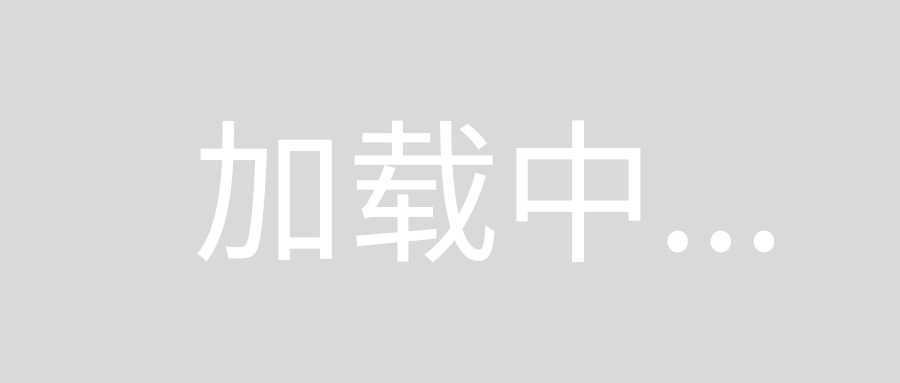
In the end it follows the binary when you compile it into the bin/Debug
or bin/Release
folders.
With C#
you then use the [DllImport()]
attrbiute like this:
[DllImport("trex_pc.dll")]
static extern Simpson(ref int N, ref int H, ref int I);
For more details look at this answer from me.
You could simply create a Console project in IVF and link in your DLL. That may require producing a .lib file containing references to your DLL. I'm not 100% sure how to do that, although perhaps it was automatically created for you.
After the library is linked in, you can simply call simpson
and it should work.
You can use the Post-Build Event in the dll Property Pages:
- Click right on DLL-project in Solution Explorer
- goto Build Events - post-Build Events
- Command Line
copy/y "$(OutDir)\$(ProjectName).dll" "$(SolutionDir)\bin\debug\"
The DLL will be copied each time you build the DLL or Solution.
The target location might be different.