I'm pretty sure this is not duplicate because I looked at other answers and they all outdated and do not provide exact answer.
I have Navigation Controller and several view controllers. I want to make Navigation Bar a bit taller so it would fit text size that I need. How can I do that ?
I tried this:
UINavigationBar.appearance().frame = CGRect(x: 0.0, y: 0.0, width: 320.0, height: 210.0)
but nothing happens...
Also, I wasn't able to add any constraints to the Nav Bar using Xcode layout buttons.
Hope somebody can help me to fix this issue. Thanks in advance!
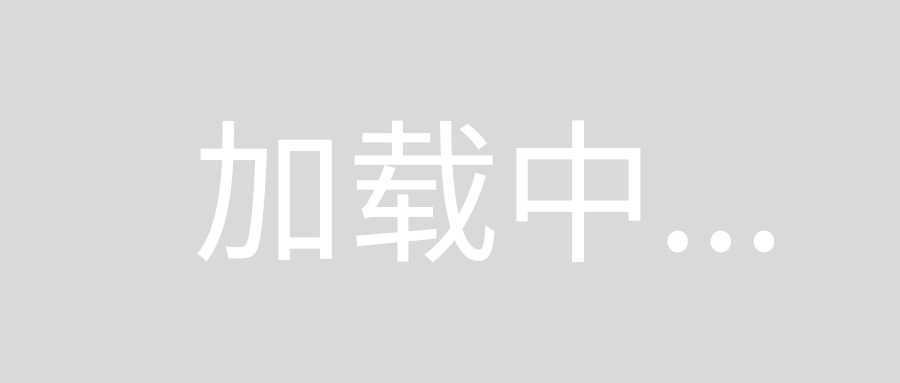
Here is one way to do it:
override func viewDidAppear(_ animated: Bool) {
super.viewDidAppear(animated)
let height: CGFloat = 50 //whatever height you want to add to the existing height
let bounds = self.navigationController!.navigationBar.bounds
self.navigationController?.navigationBar.frame = CGRect(x: 0, y: 0, width: bounds.width, height: bounds.height + height)
}
You can write this code in class that extends UINavigationController
override func viewDidLayoutSubviews() {
super.viewDidLayoutSubviews()
let height = CGFloat(72)
navigationBar.frame = CGRect(x: 0, y: 0, width: view.frame.width, height: height)
}
You can create a class based on UINavigationBar
like this
class TTNavigationBar: UINavigationBar {
override func sizeThatFits(_ size: CGSize) -> CGSize {
return CGSize(width: UIScreen.main.bounds.width, height: 55)
}
}
And add it to your NavigationController.
This might be better if your navigation bars in your app all have same adjusted height.
extension UINavigationBar {
open override func sizeThatFits(_ size: CGSize) -> CGSize {
return CGSize(width: UIScreen.main.bounds.width, height: 51)
}
}
you can draw your navigation bar from xib (with your height) and then:
self.navigationController?.navigationBar.addSubview(yourNavBarView)
in alternative, if you want create UIView from code,
let viewNavBar = UIView(frame: CGRect(
origin: CGPoint(x: 0, y:0),
size: CGSize(width: self.view.frame.size.width, height: 100)))
and then adding to nav bar.