In iPhone OS 3.0, Apple added the ability to share multiple pictures at once using the "Share" button and selecting multiple images (where a checkmark is used).
I'd love to have a UIImagePickerController which lets the user select multiple images at once, rather than having to go one by one. Is there a way to do this, or do I have to wait until they add this feature?
Try this wonderful API in swift: ImagePicker. As all other image APIs, it is simple to use and it is very well updated.
AssetLibrary + UICollectionView ^^
Basically, with StoryBoard, you import aUINavigationController
, you change the root controller to anUICollectionViewController
(will be your Album list), end add anotherUICollectionViewController
(will be your photos list).
Then with Assetlibrary you retrieve user albums and user album content.
I will make a such component as soon as i have some time.
1.install pod - pod "BSImagePicker", "~> 2.8"
- inside info plist add row Privacy - Photo Library Usage Description
3.paste below code inside a .swift file-
import UIKit
import BSImagePicker
import Photos
class MultipleImgViC: UIViewController {
@IBOutlet weak var imageView: UIImageView!
var SelectedAssets = [PHAsset]()
var photoArray = [UIImage]()
override func viewDidLoad() {
super.viewDidLoad()
}
@IBAction func selectImages(_ sender: Any) {
let vc = BSImagePickerViewController()
self.bs_presentImagePickerController(vc, animated: true, select: { (assest: PHAsset) -> Void in
},
deselect: { (assest: PHAsset) -> Void in
}, cancel: { (assest: [PHAsset]) -> Void in
}, finish: { (assest: [PHAsset]) -> Void in
for i in 0..<assest.count
{
self.SelectedAssets.append(assest[i])
}
self.convertAssetToImages()
}, completion: nil)
}
@IBAction func dismissview(_ sender: Any) {
dismiss(animated: true, completion: nil)
}
}
extension MultipleImgViC{
func convertAssetToImages() -> Void {
if SelectedAssets.count != 0{
for i in 0..<SelectedAssets.count{
let manager = PHImageManager.default()
let option = PHImageRequestOptions()
var thumbnail = UIImage()
option.isSynchronous = true
manager.requestImage(for: SelectedAssets[i], targetSize: CGSize(width: 200, height: 200), contentMode: .aspectFill, options: option, resultHandler: {(result,info) -> Void in
thumbnail = result!
})
let data = thumbnail.jpegData(compressionQuality: 0.7)
let newImage = UIImage(data: data!)
self.photoArray.append(newImage! as UIImage)
}
self.imageView.animationImages = self.photoArray
self.imageView.animationDuration = 3.0
self.imageView.startAnimating()
}
}
}
Note :- if pod file give "How to fix “SWIFT_VERSION '3.0' is unsupported, supported versions are: 4.0, 4.2, 5.0” error in Xcode 10.2?
" this error then solve it from this link:- https://stackoverflow.com/a/55901964/8537648
video reference: - https://youtu.be/B1DelPi1L0U
sample image:-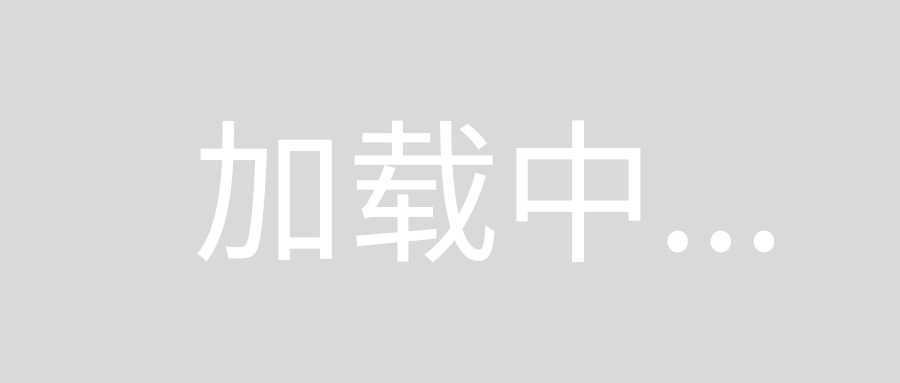
You can use this OpalImagePicker like this (Swift 4):
var imagePicker: OpalImagePickerController!
imagePicker = OpalImagePickerController()
imagePicker.imagePickerDelegate = self
imagePicker.selectionImage = UIImage(named: "aCheckImg")
imagePicker.maximumSelectionsAllowed = 3 // Number of selected images
present(imagePicker, animated: true, completion: nil)
And then implement its delegate:
func imagePickerDidCancel(_ picker: OpalImagePickerController) {
//Cancel action
}
func imagePicker(_ picker: OpalImagePickerController, didFinishPickingImages images: [UIImage]) {
}
How about this way:
Open "photos.app" first, select multiple photos , and copy them ;
In your own app, try to retrieve those copies photos;
I knew that there are some apps did like this, but do not know how can achieve step 2.