I have a bootstrap website where the Hamburger
is added when the screen is less than 992px. The HamBurger code is like so
<button class="navbar-toggler navbar-toggler-right" type="button" data-toggle="collapse" data-target="#navbarSupportedContent" aria-controls="navbarSupportedContent" aria-expanded="false" aria-label="Toggle navigation"> <span class="navbar-toggler-icon"></span> </button>
Is there any possibility to change the color of the HamBurger button?
The navbar-toggler-icon
(hamburger) in Bootstrap 4 uses an SVG background-image
. There are 2 "versions" of the toggler icon image. One for a light navbar, and one for a dark navbar...
- Use
navbar-dark
for a light/white toggler on darker backgrounds
- Use
navbar-light
for a dark/gray toggler on lighter backgrounds
// this is a black icon with 50% opacity
.navbar-light .navbar-toggler-icon {
background-image: url("data:image/svg+xml;..");
}
// this is a white icon with 50% opacity
.navbar-dark .navbar-toggler-icon {
background-image: url("data:image/svg+xml;..");
}
Therefore, if you want to change the color of the toggler image to something else, you can customize the icon. For example, here I set the RGB value to pink (255,102,203). Notice the stroke='rgba(255,102,203, 0.5)'
value in the SVG data:
.custom-toggler .navbar-toggler-icon {
background-image: url("data:image/svg+xml;charset=utf8,%3Csvg viewBox='0 0 32 32' xmlns='http://www.w3.org/2000/svg'%3E%3Cpath stroke='rgba(255,102,203, 0.5)' stroke-width='2' stroke-linecap='round' stroke-miterlimit='10' d='M4 8h24M4 16h24M4 24h24'/%3E%3C/svg%3E");
}
.custom-toggler.navbar-toggler {
border-color: rgb(255,102,203);
}
Demo http://www.codeply.com/go/4FdZGlPMNV
OFC, another option to just use an icon from another library ie: Font Awesome, etc..
Update Bootstrap 4.0.0:
As of Bootstrap 4 Beta, navbar-inverse
is now navbar-dark
to use on navbars with darker background colors to produce lighter link and toggler colors.
How to change Bootstrap 4 Navbar colors
Use a font-awesome icon as the default icon of your navbar.
<span class="navbar-toggler-icon">
<i class="fas fa-bars" style="color:#fff; font-size:28px;"></i>
</span>
Or try this on old font-awesome versions:
<span class="navbar-toggler-icon">
<i class="fa fa-navicon" style="color:#fff; font-size:28px;"></i>
</span>
You can create the toggler button with css only in a very easy way, there is no need to use any fonts in SVG or ... foramt.
Your Button:
<button
class="navbar-toggler collapsed"
data-target="#navbarsExampleDefault"
data-toggle="collapse">
<span class="line"></span>
<span class="line"></span>
<span class="line"></span>
</button>
Your Button Style:
.navbar-toggler{
width: 47px;
height: 34px;
background-color: #7eb444;
}
Your horizontal line Style:
.navbar-toggler .line{
width: 100%;
float: left;
height: 2px;
background-color: #fff;
margin-bottom: 5px;
}
Demo
.navbar-toggler{
width: 47px;
height: 34px;
background-color: #7eb444;
border:none;
}
.navbar-toggler .line{
width: 100%;
float: left;
height: 2px;
background-color: #fff;
margin-bottom: 5px;
}
<button class="navbar-toggler" data-target="#navbarsExampleDefault" data-toggle="collapse" aria-expanded="true" >
<span class="line"></span>
<span class="line"></span>
<span class="line" style="margin-bottom: 0;"></span>
</button>
EDIT : my bad! With my answer, the icon won't behave as a toggler
Actually, it will be shown even when not collapsed...
Still searching...
This would work :
<button class="btn btn-primary" type="button" data-toggle="collapse"
data-target="#navbarSupportedContent" aria-controls="navbarSupportedContent"
aria-expanded="false" aria-label="Toggle navigation">
<span>
<i class="fas fa-bars"></i>
</span>
</button>
The trick proposed by my answer is to replace the navbar-toggler
with a classical button class btn
and then, as answered earlier, use an icon font.
Note, that if you keep <button class="navbar-toggler">
, the button will have a "strange" shape.
As stated in this post on github, bootstrap uses some "css trickery", so users don't have to rely on fonts.
So, just don't use the "navbar-toggler"
class on your button if you want to use an icon font.
Cheers.
just insert class navbar-dark
or navbar-light
in the nav element:
<nav class="navbar navbar-dark navbar-expand-md">
<button class="navbar-toggler">
<span class="navbar-toggler-icon"></span>
</button>
</nav>
If you work with sass version of bootstrap in _variables.scss
you can find $navbar-inverse-toggler-bg
or $navbar-light-toggler-bg
where you can change the color and style of your toggle button.
In html you have to use navbar-inverse
or navbar-light
depending on which version you want to use.
I just developed a considerably easier solution. (Yes, I know this is an old question but someone researching this same issue may find this useful.)
I was using an SVG called hamburger.svg. I looked at it with a text editor and couldn't find anything that was setting a colour for the three lines - I'm guessing it defaults to black because that's certainly the behaviour I get - so I simply added a "stroke" parameter to the definition of the SVG. That didn't QUITE work - the borders of the three lines were my chosen colour (white) but the rest of the line was still black so I added a "fill" parameter as well. And that did the trick!
Here is the code for the original hamburger.svg in its entirety:
<?xml version="1.0" ?><!DOCTYPE svg PUBLIC '-//W3C//DTD SVG 1.1//EN' 'http://www.w3.org/Graphics/SVG/1.1/DTD/svg11.dtd'><svg height="32px" id="Layer_1" style="enable-background:new 0 0 32 32;" version="1.1" viewBox="0 0 32 32" width="32px" xml:space="preserve" xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink"><path d="M4,10h24c1.104,0,2-0.896,2-2s-0.896-2-2-2H4C2.896,6,2,6.896,2,8S2.896,10,4,10z M28,14H4c-1.104,0-2,0.896-2,2 s0.896,2,2,2h24c1.104,0,2-0.896,2-2S29.104,14,28,14z M28,22H4c-1.104,0-2,0.896-2,2s0.896,2,2,2h24c1.104,0,2-0.896,2-2 S29.104,22,28,22z"/></svg>
And here is the code for the new SVG after I edited it and saved it as hamburger_white.svg:
<?xml version="1.0" ?><!DOCTYPE svg PUBLIC '-//W3C//DTD SVG 1.1//EN' 'http://www.w3.org/Graphics/SVG/1.1/DTD/svg11.dtd'><svg height="32px" id="Layer_1" style="enable-background:new 0 0 32 32;" version="1.1" viewBox="0 0 32 32" width="32px" xml:space="preserve" xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink"><path d="M4,10h24c1.104,0,2-0.896,2-2s-0.896-2-2-2H4C2.896,6,2,6.896,2,8S2.896,10,4,10z M28,14H4c-1.104,0-2,0.896-2,2 s0.896,2,2,2h24c1.104,0,2-0.896,2-2S29.104,14,28,14z M28,22H4c-1.104,0-2,0.896-2,2s0.896,2,2,2h24c1.104,0,2-0.896,2-2 S29.104,22,28,22z" stroke="white" fill="white"/></svg>
As you can see if you scroll way over to the right, all I did was add:
stroke="white" fill="white"
to the very end of the path. The other thing I had to do was change the file name of the hamburger in the HTML. No messing with the CSS at all and no need to track down another icon.
Easy-peasey! You can imitate this to make your hamburger any colour you like.
As alternative you always can try a simpler workaround, using another icon, for example:
<button type="button" style="background:none;border:none">
<span class="fa fa-reorder"></span>
</button>
ref: https://www.w3schools.com/icons/fontawesome_icons_webapp.asp
<button type="button" style="background:none;border:none">
<span class="glyphicon glyphicon-align-justify"></span>
</button>
ref: https://www.w3schools.com/icons/bootstrap_icons_glyphicons.asp
So you gain total control over their color and size:
button span {
/*overwriting*/
color: white;
font-size: 25px;
}
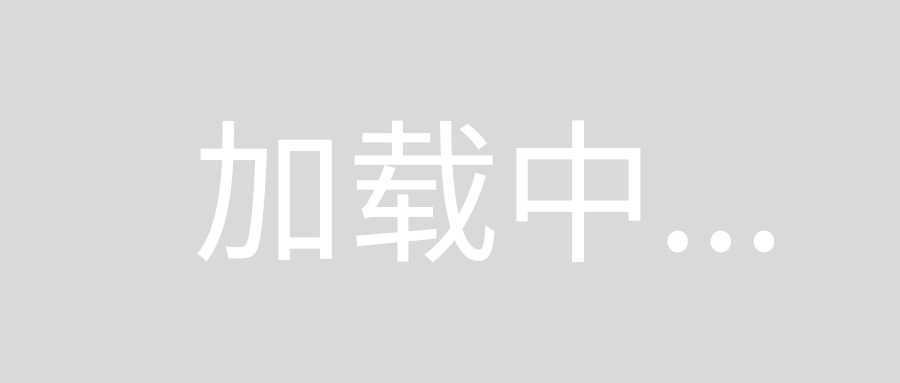
(the button's style applied is just for a quick test):
Check the best solution for custom hamburger nav.
@import "https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css";
.bg-iconnav {
background: #f0323d;
/* Old browsers */
background: -moz-linear-gradient(top, #f0323d 0%, #e6366c 100%);
/* FF3.6-15 */
background: -webkit-linear-gradient(top, #f0323d 0%, #e6366c 100%);
/* Chrome10-25,Safari5.1-6 */
background: linear-gradient(to bottom, #f0323d 0%, #e6366c 100%);
/* W3C, IE10+, FF16+, Chrome26+, Opera12+, Safari7+ */
filter: progid:DXImageTransform.Microsoft.gradient( startColorstr='#f0323d', endColorstr='#e6366c', GradientType=0);
/* IE6-9 */
border-radius: 0;
padding: 10px;
}
.navbar-toggler-icon {
background-image: url("data:image/svg+xml;charset=utf8,%3Csvg viewBox='0 0 32 32' xmlns='http://www.w3.org/2000/svg'%3E%3Cpath stroke='rgba(255,255,255, 1)' stroke-width='2' stroke-linecap='round' stroke-miterlimit='10' d='M4 8h24M4 16h24M4 24h24'/%3E%3C/svg%3E");
}
<button class="navbar-toggler bg-iconnav" type="button">
<span class="navbar-toggler-icon"></span>
</button>
demo image