View v = rootView.findViewById(R.id.layout1);
if (v != null) {
v.buildDrawingCache();
Bitmap bitmap = v.getDrawingCache();
canvas.drawBitmap(bitmap, dummyMatrix, null);
v.destroyDrawingCache();
}
I have this code. But I need to screenshot all my ListView items, but if my listview's have more items than visible on the screen, this code don't capture when the items bigger than the visible rect.
How to capture my ListView correctly?
NEW WORKING CODE CREATED BY ME
public static Bitmap getWholeListViewItemsToBitmap() {
ListView listview = MyActivity.mFocusedListView;
ListAdapter adapter = listview.getAdapter();
int itemscount = adapter.getCount();
int allitemsheight = 0;
List<Bitmap> bmps = new ArrayList<Bitmap>();
for (int i = 0; i < itemscount; i++) {
View childView = adapter.getView(i, null, listview);
childView.measure(MeasureSpec.makeMeasureSpec(listview.getWidth(), MeasureSpec.EXACTLY),
MeasureSpec.makeMeasureSpec(0, MeasureSpec.UNSPECIFIED));
childView.layout(0, 0, childView.getMeasuredWidth(), childView.getMeasuredHeight());
childView.setDrawingCacheEnabled(true);
childView.buildDrawingCache();
bmps.add(childView.getDrawingCache());
allitemsheight+=childView.getMeasuredHeight();
}
Bitmap bigbitmap = Bitmap.createBitmap(listview.getMeasuredWidth(), allitemsheight, Bitmap.Config.ARGB_8888);
Canvas bigcanvas = new Canvas(bigbitmap);
Paint paint = new Paint();
int iHeight = 0;
for (int i = 0; i < bmps.size(); i++) {
Bitmap bmp = bmps.get(i);
bigcanvas.drawBitmap(bmp, 0, iHeight, paint);
iHeight+=bmp.getHeight();
bmp.recycle();
bmp=null;
}
return bigbitmap;
}
working code:
public static Bitmap getWholeListViewItemsToBitmap() {
ListView listview = MyActivity.mFocusedListView;
ListAdapter adapter = listview.getAdapter();
int itemscount = adapter.getCount();
int allitemsheight = 0;
List<Bitmap> bmps = new ArrayList<Bitmap>();
for (int i = 0; i < itemscount; i++) {
View childView = adapter.getView(i, null, listview);
childView.measure(MeasureSpec.makeMeasureSpec(listview.getWidth(), MeasureSpec.EXACTLY),
MeasureSpec.makeMeasureSpec(0, MeasureSpec.UNSPECIFIED));
childView.layout(0, 0, childView.getMeasuredWidth(), childView.getMeasuredHeight());
childView.setDrawingCacheEnabled(true);
childView.buildDrawingCache();
bmps.add(childView.getDrawingCache());
allitemsheight+=childView.getMeasuredHeight();
}
Bitmap bigbitmap = Bitmap.createBitmap(listview.getMeasuredWidth(), allitemsheight, Bitmap.Config.ARGB_8888);
Canvas bigcanvas = new Canvas(bigbitmap);
Paint paint = new Paint();
int iHeight = 0;
for (int i = 0; i < bmps.size(); i++) {
Bitmap bmp = bmps.get(i);
bigcanvas.drawBitmap(bmp, 0, iHeight, paint);
iHeight+=bmp.getHeight();
bmp.recycle();
bmp=null;
}
return bigbitmap;
}
While it is impossible to make a screenshot of not-yet-rendered content (like off-screen items of the ListView), you can make a multiple screenshots, scroll content between each shot, then join images. Here is a tool which can automate this for you: https://github.com/PGSSoft/scrollscreenshot
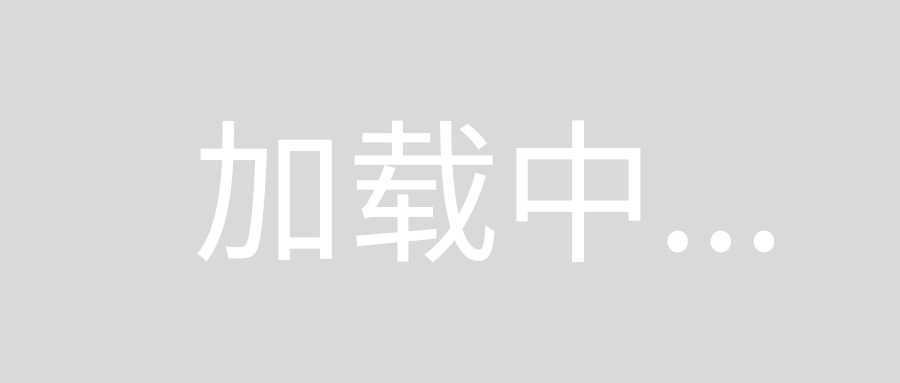
Disclaimer: I'm author of this tool, it was published by my employer. Feature requests are welcome.
Use this function to get bitmap of your list view
public Bitmap getBitmapFromView(View view) {
Bitmap returnedBitmap = Bitmap.createBitmap(view.getMeasuredWidth(),
view.getMeasuredHeight() , Bitmap.Config.ARGB_8888);
Canvas canvas = new Canvas(returnedBitmap);
Drawable bgDrawable = view.getBackground();
if (bgDrawable != null)
bgDrawable.draw(canvas);
else
canvas.drawColor(Color.WHITE);
view.draw(canvas);
return returnedBitmap;
}
use this as
Bitmap b = getBitmapFromView(your listview object here);
and use this bitmap as you want
hope help..
if your generated bitmap is having black color background . this is because you view unable to fetch color from xml file. so set a color of your view
View v = adapter.getView(i, null, listview);
v.measure(View.MeasureSpec.makeMeasureSpec(listview.getWidth(), View.MeasureSpec.EXACTLY),
View.MeasureSpec.makeMeasureSpec(0, View.MeasureSpec.UNSPECIFIED));
v.layout(0, 0, v.getMeasuredWidth(), v.getMeasuredHeight());
v.setDrawingCacheEnabled(true);
v.buildDrawingCache(true);
v.setBackgroundColor(Color.parseColor("#F08080"));
bmps.add(v.getDrawingCache(true));
allitemsheight += v.getMeasuredHeight();