How do I center all my RecyclerView
items using the FlexboxLayoutManager
?
I need the items to be centered like this:
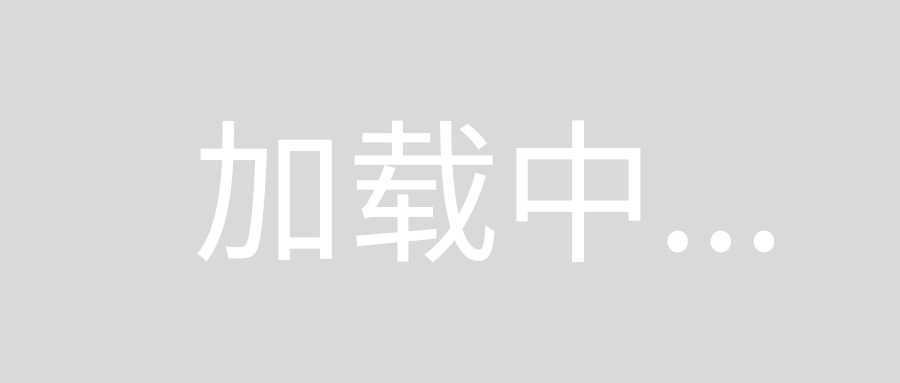
I tried without success:
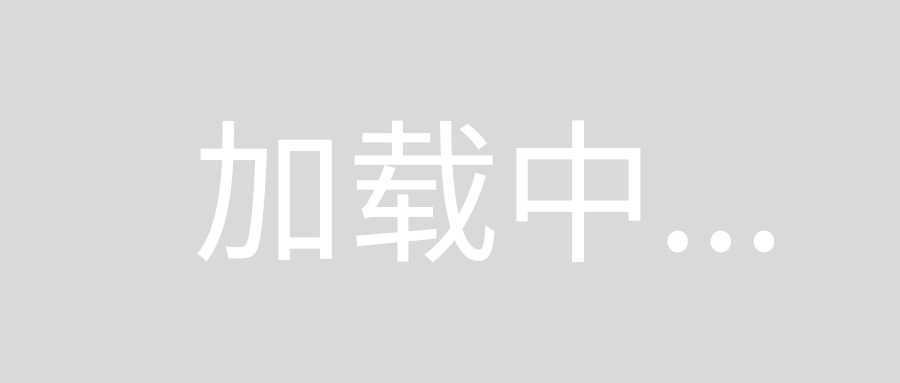
My code where I set the layout manager:
val layoutManager = FlexboxLayoutManager(this)
layoutManager.setFlexWrap(FlexWrap.WRAP)
layoutManager.setFlexDirection(FlexDirection.ROW)
layoutManager.setJustifyContent(JustifyContent.FLEX_START)
layoutManager.setAlignItems(AlignItems.FLEX_START)
val adapter = TagAdapter(tags)
tagRecyclerView.adapter = adapter
tagRecyclerView.layoutManager = layoutManager
(I tried to set layoutManager.setAlignItems(AlignItems.FLEX_START
) to layoutManager.setAlignItems(AlignItems.CENTER)
however it did not work...
Try this
ACTIVITY CODE
public class TestActivity extends AppCompatActivity {
RecyclerView recyclerView;
ArrayList<String> arrayList = new ArrayList<>();
FlexboxAdapter adapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_test);
recyclerView = findViewById(R.id.recyclerView);
initArray();
FlexboxLayoutManager layoutManager = new FlexboxLayoutManager(this);
layoutManager.setFlexDirection(FlexDirection.ROW);
layoutManager.setJustifyContent(JustifyContent.CENTER);
layoutManager.setAlignItems(AlignItems.CENTER);
recyclerView.setLayoutManager(layoutManager);
adapter = new FlexboxAdapter(this, arrayList);
recyclerView.setAdapter(adapter);
}
private void initArray() {
arrayList.add("Nileshfgfdgfdgfdggfgfgfdgvcb");
arrayList.add("Nilesh");
arrayList.add("Nilesh");
arrayList.add("Nilesh");
arrayList.add("Nileshfgfdgfdgfdggfgcvbcvb");
arrayList.add("Nilesh");
arrayList.add("Nilesh");
arrayList.add("Nilesh");
arrayList.add("Nileshfgfdgfdgfdggfgfdgdfgcvb");
arrayList.add("Nilesh");
arrayList.add("Nilesh");
arrayList.add("Nilesh");
arrayList.add("Nilesh");
arrayList.add("Nilesh");
arrayList.add("Nileshfgfdgfdgfdggfgcvb");
arrayList.add("Nilesh");
arrayList.add("Nilesh");
arrayList.add("Nilesh");
arrayList.add("Nilesh");
arrayList.add("Nilesh");
arrayList.add("Nilesh");
arrayList.add("Nileshfgfdgfdgfdggfgdfgdfgcvb");
arrayList.add("Nilesh");
arrayList.add("Nilesh");
arrayList.add("Nilesh");
arrayList.add("Nileshfgfdgfdgfdggfgdfgcvb");
arrayList.add("Nilesh");
arrayList.add("Nilesh");
}
}
ADAPTER CODE
public class FlexboxAdapter extends RecyclerView.Adapter<FlexboxAdapter.ViewHolder> {
Context context;
ArrayList<String> arrayList = new ArrayList<>();
public FlexboxAdapter(Context context, ArrayList<String> arrayList) {
this.context = context;
this.arrayList = arrayList;
}
@Override
public FlexboxAdapter.ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View view = LayoutInflater.from(context).inflate(R.layout.custom_layout, parent, false);
return new ViewHolder(view);
}
@Override
public void onBindViewHolder(FlexboxAdapter.ViewHolder holder, int position) {
holder.title.setText(arrayList.get(position));
}
@Override
public int getItemCount() {
return arrayList.size();
}
public class ViewHolder extends RecyclerView.ViewHolder {
TextView title;
public ViewHolder(View itemView) {
super(itemView);
title = itemView.findViewById(R.id.tvTitle);
}
}
}
CUSTOM LAYOUT
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center">
<TextView
android:id="@+id/tvTitle"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:gravity="center"
android:padding="5dp"
android:text="Nilesh"
android:textColor="#000"
android:textSize="20sp"
android:textStyle="bold" />
</LinearLayout>
ACTIVITY LAYOUT
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".TestActivity">
<android.support.v7.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</LinearLayout>
OUTPUT
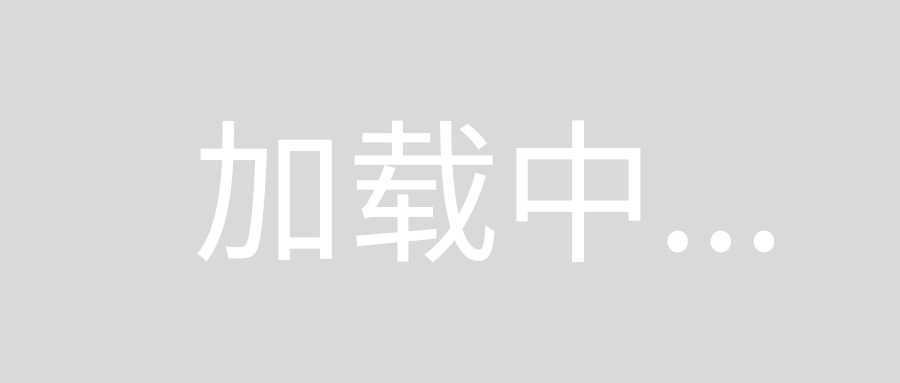
Short answer
You are using layoutManager.setAlignItems(AlignItems.FLEX_START)
. This is causing start alignment.
You should use one of below two alignment that suits your requirement.
layoutManager.setAlignItems(AlignItems.CENTER)
.
or
layoutManager.setAlignItems(AlignItems.SPACE_AROUND)
.
Note:
Because you said this
@MarcEstrada yes, don't work
Keep you item layout width wrap_content
. (all child with parent should have wrap_content
width.) like android:layout_width="wrap_content"
If you use match_parent
width then you will not get centered items.