I have a little non-conventional DIV in this design as shown below. I could use a height and do it but I want it to change dynamically:
For instance, if the DIV has more content and the height changes in one of the block on the right, the left DIV auto adjust it's height as well. I wonder if flex could help. Here's how it should change to:
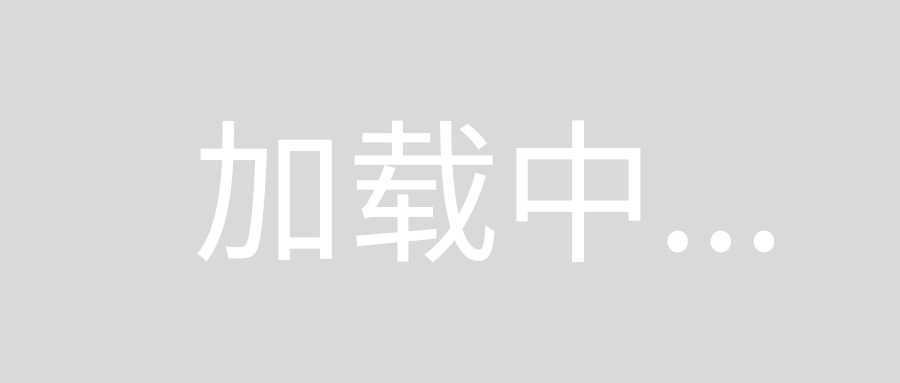
I have this HTML so far:
<div class="container">
<div class="row row-eq-height">
<div class="col-sm-8 col-8">
<div class="black">
<p>Bar Graph or Line Graph</p>
</div>
</div>
<div class="col-sm-4 col-4">
<div class="red">
<p>numbers</p>
</div>
<div class="purple">
<p>numbers</p>
</div>
<div class="green">
<p>numbers</p>
</div>
<div class="blue">
<p>numbers</p>
</div>
</div>
</div>
</div>
and CSS:
p { color: #fff; }
.black { background-color: black; }
.green { background-color: green; }
.red { background-color: red; }
.blue { background-color: blue; }
.purple { background-color: purple; }
JSFiddle Demo
You can use flexbox for this.
Update
And another way:
https://jsfiddle.net/persianturtle/ar0m0p3a/5/
.row-eq-height > [class^=col] {
display: flex;
flex-direction: column;
}
.row-eq-height > [class^=col]:last-of-type div {
margin: 10px;
}
.row-eq-height > [class^=col]:last-of-type div:first-of-type {
margin-top: 0;
}
.row-eq-height > [class^=col]:last-of-type div:last-of-type {
margin-bottom: 0;
}
.row-eq-height > [class^=col]:first-of-type .black {
flex-grow: 1;
}
Update
A better way with more specific classes:
https://jsfiddle.net/persianturtle/ar0m0p3a/3/
.row-eq-height > [class^=col]:first-of-type {
display: flex;
}
.row-eq-height > [class^=col]:first-of-type .black {
flex-grow: 1;
}
.blue p {
margin: 0;
}
Original way:
https://jsfiddle.net/persianturtle/ar0m0p3a/1/
[class^=col] {
display: flex;
flex-direction: column;
}
[class^=col] div {
flex-grow: 1
}
You're using Bootstrap 4, right? Bootstrap 4 implements flexbox by default and you can solve this very easy while using purely classes Bootstrap 4 provides:
<div class="container">
<div class="row">
<div class="col-sm-8 col-8 black">
<p>Bar Graph or Line Graph</p>
</div>
<div class="col-sm-4 col-4 d-flex align-content-around flex-wrap">
<div class="red">
<p>numbers and more and so on and on and on</p>
</div>
<div class="purple">
<p>numbers</p>
</div>
<div class="green">
<p>numbers</p>
</div>
<div class="blue">
<p class="mb-0">numbers</p>
</div>
</div>
</div>
</div>
JS Fiddle link: https://jsfiddle.net/ydjds2ko/
Details:
You don't need the class row-eq-height
(it's not in Bootstrap4
anyway) because equal height is default.
The first div with the class col-sm-8
has the right height, it's just not visible with the black background, because the inner div has it's own height. I just removed the inner div and added the class black
to the col. If you need inner div, give it the (Bootstrap4) class h-100
which adjusts the height to the parent element.
the div with the class col-sm-4 get's a the classes d-flex align-content-around flex-wrap. They're aligning the content divs. Bootstrap doku: https://getbootstrap.com/docs/4.0/utilities/flex/#align-content
- Set the width of the divs inside this container at the widht of the parent with w-100
Because the <p>
adds a margin at the bottom, your blue div at the
ends doesn't close flush with the black div. I added the class
"mb-0", which sets the margin bottom to "0" so you can see it works.
That should work wether the right or left div is the one with the bigger height property.
Edit: added classes for content alignment.
Here's a very simple Bootstrap only approach for this. You don't need any additional CSS.
https://www.codeply.com/go/J3BHCFT7lF
<div class="container">
<div class="row">
<div class="col-8">
<div class="black h-100">
<p>Bar Graph or Line Graph</p>
</div>
</div>
<div class="col-4 d-flex flex-column">
<div class="red mb-2">
<p>numbers</p>
</div>
<div class="purple mb-2">
<p>numbers with more lines of content that wrap to the next line</p>
</div>
<div class="green mb-2">
<p>numbers</p>
</div>
<div class="blue">
<p>numbers</p>
</div>
</div>
</div>
</div>
This uses the Bootstrap 4 utility classes to make the right column fill height.
Related:
bootstrap 4 row height