I'm customizing a UISlider
. I could set a custom thumb image that is higher than the usual thumb however I could not make the track higher when setting a higher minimum track image but the track height remained the same.
It should be possible as in the iPod/Music App on the iPad the volume slider is also higher as the usual slider as you can see here:
http://blog.cocoia.com/wp-content/uploads/2010/01/Lol-wut.png
You need to subclass the slider and override the trackRectForBounds:
method, like this:
- (CGRect)trackRectForBounds:(CGRect)bounds
{
return bounds;
}
The simplest solution for Swift:
class TBSlider: UISlider {
override func trackRectForBounds(bounds: CGRect) -> CGRect {
return CGRectMake(0, 0, bounds.size.width, 4)
}
}
use next methods setThumbImage, setMinimumTrackImage, setMaximumTrackImage
[self setThumbImage:[UIImage imageNamed:@"switchThumb.png"] forState:UIControlStateNormal];
[self setMinimumTrackImage:[UIImage imageNamed:@"switchBlueBg.png"] forState:UIControlStateNormal];
[self setMaximumTrackImage:[UIImage imageNamed:@"switchOffPlain.png"] forState:UIControlStateNormal];
and create subclass like this
- (id) initWithFrame: (CGRect)rect{
if ((self=[super initWithFrame:CGRectMake(rect.origin.x,rect.origin.y,90,27)])){
[self awakeFromNib];
}
return self;
}
For those that would like to see some working code for changing the track size.
class CustomUISlider : UISlider
{
override func trackRectForBounds(bounds: CGRect) -> CGRect {
//keeps original origin and width, changes height, you get the idea
let customBounds = CGRect(origin: bounds.origin, size: CGSize(width: bounds.size.width, height: 5.0))
super.trackRectForBounds(customBounds)
return customBounds
}
//while we are here, why not change the image here as well? (bonus material)
override func awakeFromNib() {
self.setThumbImage(UIImage(named: "customThumb"), forState: .Normal)
super.awakeFromNib()
}
}
Only thing left is changing the class inside the storyboard:
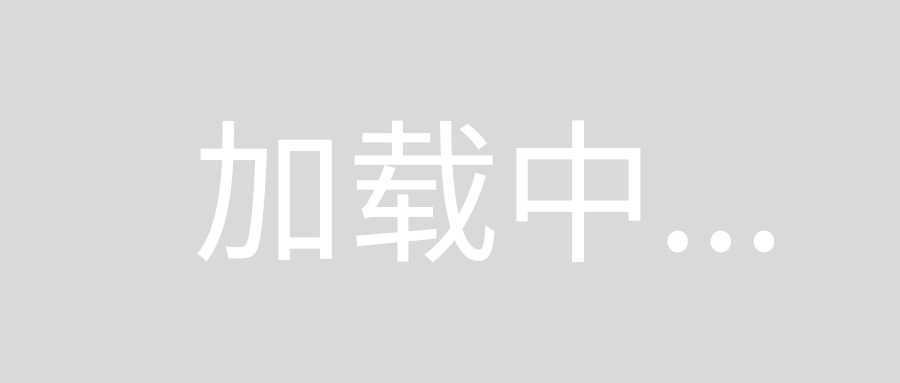
You can keep using your seekbar action and outlet to the object type UISlider, unless you want to add some more custom stuff to your slider.
//use this code
UIImage *volumeLeftTrackImage = [[UIImage imageNamed: @"video_payer_scroll_selection.png"] stretchableImageWithLeftCapWidth: 9 topCapHeight: 0];
UIImage *volumeRightTrackImage= [[UIImage imageNamed: @"video_bar_bg.png"] stretchableImageWithLeftCapWidth: 9 topCapHeight: 0];
[volumeslider setMinimumTrackImage: volumeLeftTrackImage forState: UIControlStateNormal];
[volumeslider setMaximumTrackImage: volumeRightTrackImage forState: UIControlStateNormal];
[volumeslider setThumbImage:[UIImage imageNamed:@"sound_bar_btn.png"] forState:UIControlStateNormal];
[tempview addSubview:volumeslider];