Can you change the length of one side of a rectangle in pygame?
For example:
img = pygame.image.load('image.gif')
rect = img.get_rect()
In that example I load an image and create a rectangle around it. With that in mind could I, for instance, change the length of the bottom of the rect with out it changing any other sides of the rectangle?
You can use PyGames Rect objects to store rectangular coordinates.
As @Tehsmeely mentioned, there are a number of so-called virtual attributes which can be used to move or align a Rect object in your game:
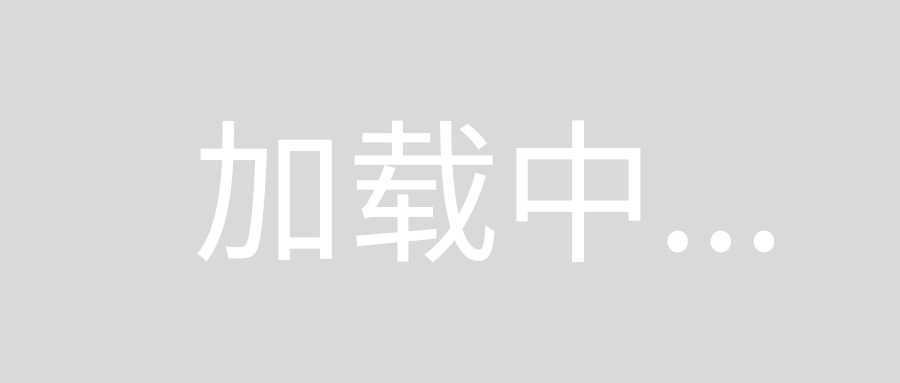
When you want to load an image form a file into your game the pygame.image.load()
function returns a new Surface object which is like your main screen surface. You can blit (i.e. copy) this new surface object onto any other surface you want by using the .blit()
method:
The first argument of .blit()
is the source surface.
The second argument can either be pair of coordinates representing the upper left corner of the source surface
or a Pygame Rect object where the topleft corner of the rectangle will be used as the position for the blit.
The size of the destination rectangle does not affect the blit.
This means you can change the length of the bottom of your rect
:
rect.width= 100
but this will not affect the size of your img
.
I hope this helps :)
Have a look here: http://www.pygame.org/docs/ref/rect.html
A rect is simply a data structure for these things, and you can set any of the below properties of a rect
x,y
top, left, bottom, right
topleft, bottomleft, topright, bottomright
midtop, midleft, midbottom, midright
center, centerx, centery
size, width, height
w,h
just make a new rect in python shell and have a play, you can make Rects themselves
import pygame
rect = pygame.Rect((10,10),(20,20))
print rect.height
>>20
rect.height = 30
print rect.height
>>30
Edit:
This won't effect the image you loaded with regards to how it appears, to draw to a screen you need a surface and a rect, if you want to modify the image you need to change the surface. Alternatively stretching you loaded imaged by drawing to an increased rect WILL work, check out the explanation for Surface.blit, http://www.pygame.org/docs/ref/surface.html#pygame.Surface.blit