I have the view below:
@Html.LabelFor(m => m.CompanyPostCode)
@Html.TextBoxFor(m => m.CompanyPostCode)
@Html.LabelFor(m => m.CompanyCity)
@Html.TextBoxFor(m => m.CompanyCity)
@Html.HiddenFor(m => m.CompanyCityID)
All attributes are marked as [Required] in my view model. Then problem is that my CompanyCityID (marked as Required) is hidden and thus no validation is done in the view. If I show this attribute in my view the validation is done.
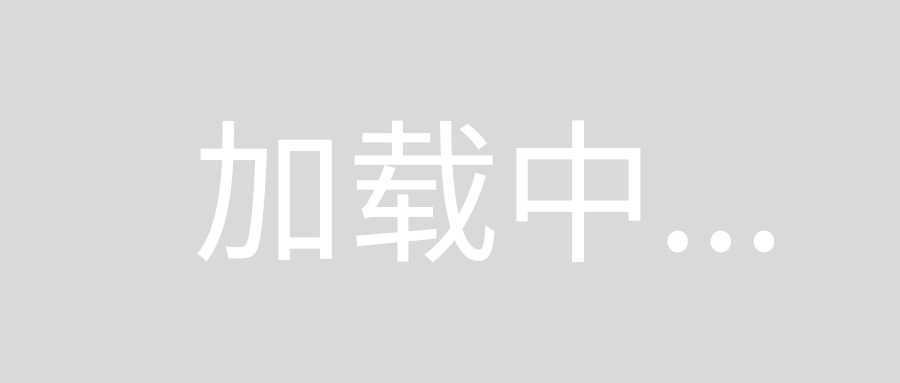
My question: is it possible to perform a validation on a hidden field? A workaround exist?
It may seems a little strange to validate a hidden field. The reason is that this field is filled from jQuery based on special rules. If it is not filled, I know something is not valid on the view.
Thanks.
The possible reason can be that there is ignore: ':hidden'
line in jquery.validate.unobtrusive.js
file.
After 1.9.0 version it is a default behaviour. You can fix that manually by adding
$.validator.setDefaults({ ignore: [] });
As you can see here
Another change should make the setup of forms with hidden elements
easier, these are now ignored by default (option “ignore” has
“:hidden” now as default). In theory, this could break an existing
setup. In the unlikely case that it actually does, you can fix it by
setting the ignore-option to “[]” (square brackets without the
quotes).
You can also just comment out this line in the jquery.validate.js file.
ignore: ":hidden"
If you don't have to use javascript, in your Controller, and in your action of the related view, you can add a model error before validating your model. Example:
[HttpPost]
public ActionResult Fix(YourModel mdl)
{
if (mdl.CompanyCityID==0)
ModelState.AddModelError("", "Your error message!");
if (ModelState.IsValid)
{
//
//Some code
//
return View("YourView", yourlist);
}
return View(mdl);
}
You can always put @Html.HiddenFor(m => m.CompanyCityID)
inside a hidden div and change it to EditorFor()
.
CSS
.hidden {
display: none;
}
View
<div class="hidden">
@Html.EditorFor(m => m.CompanyCityID)
</div