I'm having trouble with drawing some lines that are stroked with a color and then filling their insides (they make a polygon) with another one.
UIColor *houseBorderColor = [UIColor colorWithRed:(170/255.0) green:(138/255.0) blue:(99/255.0) alpha:1];
CGContextSetStrokeColorWithColor(context, houseBorderColor.CGColor);
CGContextSetLineWidth(context, 3);
// Draw the polygon
CGContextMoveToPoint(context, 20, viewHeight-19.5);
CGContextAddLineToPoint(context, 200, viewHeight-19.5); // base
CGContextAddLineToPoint(context, 300, viewHeight-119.5); // right border
CGContextAddLineToPoint(context, 120, viewHeight-119.5);
CGContextAddLineToPoint(context, 20, viewHeight-19.5);
// Fill it
CGContextSetRGBFillColor(context, (248/255.0), (222/255.0), (173/255.0), 1);
//CGContextFillPath(context);
// Stroke it
CGContextStrokePath(context);
With the CGContextStrokePath
commented out, I get this result:
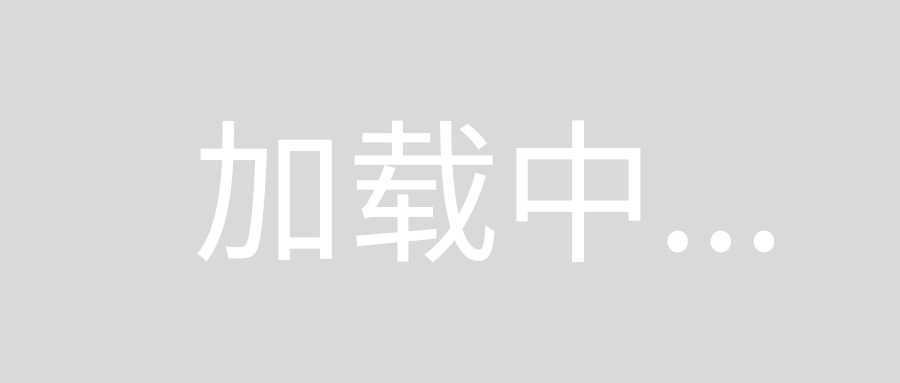
But if I uncomment CGContextStrokePath
and fill out the polygon, the color overflows the strokes:
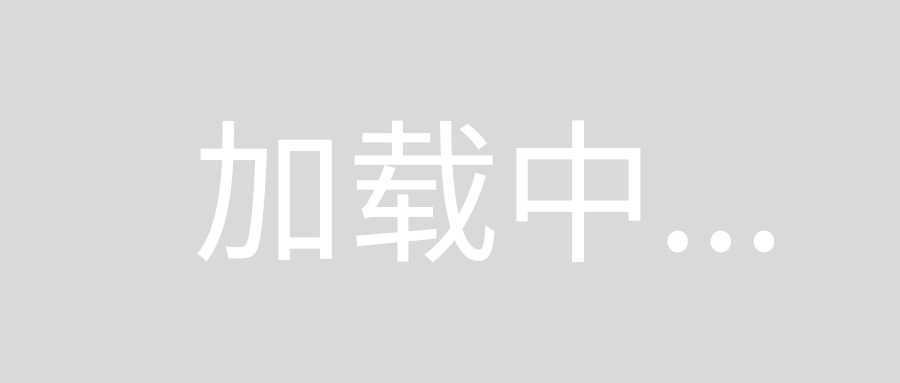
How do you achieve a result like this (without having to redo the whole drawing procedure twice):
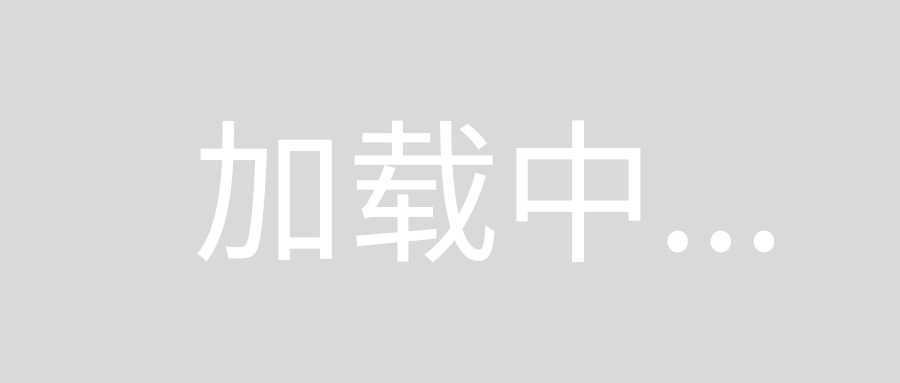
You can use
CGContextDrawPath(context, kCGPathFillStroke);
instead of
CGContextFillPath(context);
CGContextStrokePath(context);
The problem is that both CGContextFillPath()
and CGContextStrokePath(context)
clear the current path, so that only the first operation succeeds, and the second
operation draws nothing. CGContextDrawPath()
combines fill and stroke without
clearing the path in between.
Using UIBezierPath you could do this:
UIBezierPath *path = [[UIBezierPath alloc] init];
[path moveToPoint:CGPointMake(20, viewHeight-19.5)];
[path addLineToPoint:CGPointMake(200, viewHeight-19.5)];
[path addLineToPoint:CGPointMake(300, viewHeight-119.5)];
[path addLineToPoint:CGPointMake(120, viewHeight-119.5)];
[path addLineToPoint:CGPointMake(20, viewHeight-19.5)];
[[UIColor colorWithRed:(248/255.0) green:(222/255.0) blue:(173/255.0) alpha:1.0] setFill];
[path fill];
[[UIColor colorWithRed:(170/255.0) green:(138/255.0) blue:(99/255.0) alpha:1.0] setStroke];
[path stroke];
When you stroke or fill the path in the context, the context removes the path for you (it expects that it's work is done). You must add the path again if you want to fill it after stroking it.
It's probably best to create a CGPathRef path
local variable, build the path, add it, stroke, add it again, fill.
CGMutablePathRef path = CGPathCreateMutable();
CGPathMoveToPoint(path, nil, 20, viewHeight-19.5);
CGPathAddLineToPoint(path nil, 200, viewHeight-19.5); // base
CGPathAddLineToPoint(path nil, 300, viewHeight-119.5); // right border
CGPathAddLineToPoint(path nil, 120, viewHeight-119.5);
CGPathAddLineToPoint(path nil, 20, viewHeight-19.5);
CGContextAddPath(context, path);
CGContextFillPath(context);
// possibly modify the path here if you need to
CGContextAddPath(context, path);
CGContextStrokePath(context);