I know this is a simple question, but I thought I'd give it a try.
So say I have a TextBox named "toReverse". If I wanted to reverse all the text in that TextBox, how would I go about doing that?
Also, I can't use VB.NET in it, even though it has a one-line way of doing it.
char[] chararray = this.toReverse.Text.ToCharArray();
Array.Reverse(chararray);
string reverseTxt = "";
for (int i = 0; i <= chararray.Length - 1; i++)
{
reverseTxt += chararray.GetValue(i);
}
this.toReverse.Text = reverseTxt;
Hope this helps
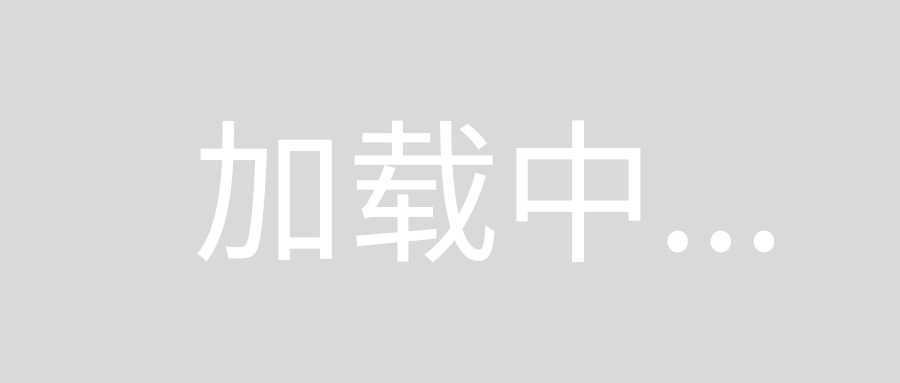
using System.Linq;
new String(str.Reverse().ToArray());
You can pass it to Array of char and reverse it :)
char[] arr = s.ToCharArray();
Array.Reverse(arr);
var yourString = new string(arr);
There are several ways you could go about doing this if you don't want to use the built-in facilities. For example, you could create an array, reverse the array (with an explicit loop rather than Array.Reverse
), and then create a new string from the array:
char[] txt = myString.ToArray();
int i = 0;
int j = txt.Length - 1;
while (i < j)
{
char t = txt[i];
txt[i] = txt[j];
txt[j] = t;
++i;
--j;
}
string reversed = new string(txt);
Another way is to use a stack. Push the individual characters on the stack, then pop them off to populate a StringBuilder
:
Stack<char> charStack = new Stack<char>();
foreach (var c in myString)
{
charStack.Push(c);
}
StringBuilder sb = new StringBuilder(myString.Length);
while (charStack.Count > 0)
{
sb.Append(charStack.Pop());
}
string reversed = sb.ToString();
Another way would be to walk through the string backwards, populating a StringBuilder
:
StringBuilder sb = new StringBuilder(myString.Length);
for (int i = myString.Length-1; i >= 0; --i)
{
sb.Append(myString[i]);
}
string reversed = sb.ToString();
And of course there are many variations to the above.