I'm looking at this fiddle http://jsfiddle.net/yezw6c51/1/ which does what I need to do with one exception.
When you load it has one Input field named 'phone_number'
When you click on Add phone number
you can add multiple rows/input fields as such :
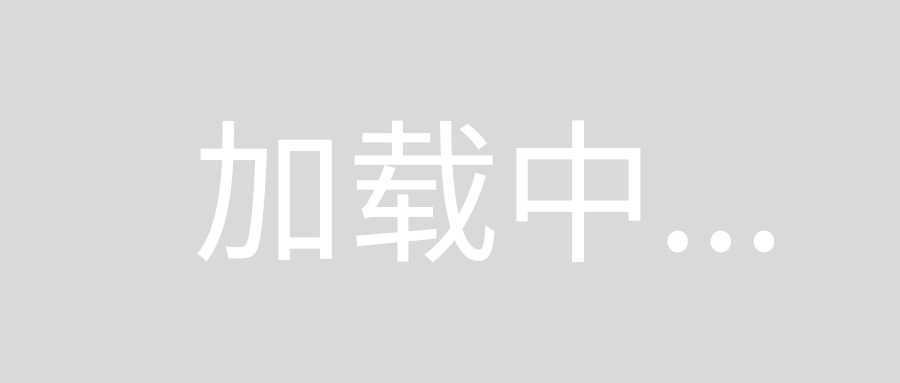
Each new input field that is added has had it's field name incremented. eg : phone_number1, phone_number2 etc. This works fine.
But I'd like the text before each input field to also have the incremented value adding as well, so you end up with :
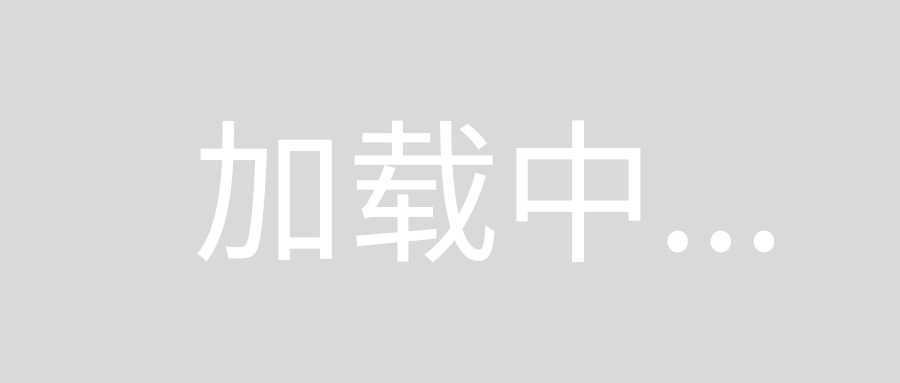
So the text description is incremented with the same value as the phone_number field.
ie: Phone Number 2 = phone_number2 etc
How do I do that ?
Thanks
Wrap your text with span
<span>Phone number :</span>
Then your can change only the text inside span
$(this).parent().find('span').text('Phone number ' + phone_number_form_index);
fiddle: http://jsfiddle.net/yezw6c51/16/
instead of giving each input different names, you can use name="phoneNumber[]" and loop through the post keys in backend.
Demo: http://jsfiddle.net/artuc/yezw6c51/23/
HTML:
<form name="test">
<div class="formRow">
<label>Phone Number <span>1</span>:</label>
<input type="text" name="phoneNumber[]" />
</div>
<a href="javascript:void(0);" class="btnAdd">Add [+]</a>
</form>
JS:
$(document).ready(function(){
$('.btnAdd').click(function(){
base = $('.formRow:first');
newRow = base.clone().insertAfter($('.formRow:last'));
newRow.find('input').val('');
newRow.find('label>span').text(newRow.index('.formRow')+1);
newRow.append('<input type="button" class="btnRemove" value="Remove"/>');
});
$(document).on('click', '.btnRemove', function(){
$(this).parent().remove();
//update index
$('.formRow').each(function(){
target = $(this).find('label>span');
target.text($(this).index('.formRow')+1);
});
});
});
You can Use a Span After "Phone Number" in your HTML.
And make these changes in your script
$(document).ready(function(){
var phone_number_form_index=1;
$("#add_phone_number").click(function(){
phone_number_form_index++;
$(this).parent().before($("#phone_number_form").clone().attr("id","phone_number_form" + phone_number_form_index));
$("#phone_number_form" + phone_number_form_index).css("display","inline");
$("#phone_number_form" + phone_number_form_index).find("span").html(phone_number_form_index);
$("#phone_number_form" + phone_number_form_index + " :input").each(function(){
$(this).attr("name",$(this).attr("name") + phone_number_form_index);
$(this).attr("id",$(this).attr("id") + phone_number_form_index);
});
$("#remove_phone_number" + phone_number_form_index).click(function(){
$(this).closest("div").remove();
});
});
});
check out here
http://jsfiddle.net/yezw6c51/24/