I am working on a program that is supposed to simulate bank accounts. I have my base class called "Investment", my two subclasses called "Stock" and "MutualFund", and I also have a class called "CustomerAccount" that is supposed to be associated with the base class "Investment". 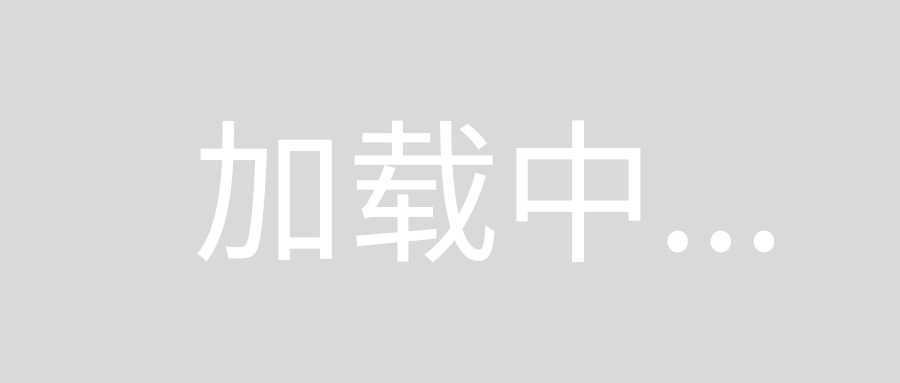
I'm not sure how to associate the CustomerAccount class with the Investment class.
The CustomerAccount class has a "CanHave" relationship with Investment.
In your CustomerAccount class, create a collection of Investments. You could use a list to achieve this:
List<Investment> _investments;
Don't forget to initialize the list in your constructor.
_investments = new List<Investment>();
Edit:
Looping through the list:
foreach Investment invst in _investments
{
if (invst.GetType() == typeof(Stock)) { }
else if (invst.GetType() == typeof(MutualFund)) { }
}
Rather than having an account have AddStock
and AddMutualFund
it should just have AddInvestment
which is then used to add either a stock or an inventment. You then get all Investment
s, rather than getting all stocks or mutual funds.