For some reason, flex items are not staying inside the container in Safari.
Here's what the layout looks like in Chrome and Firefox:
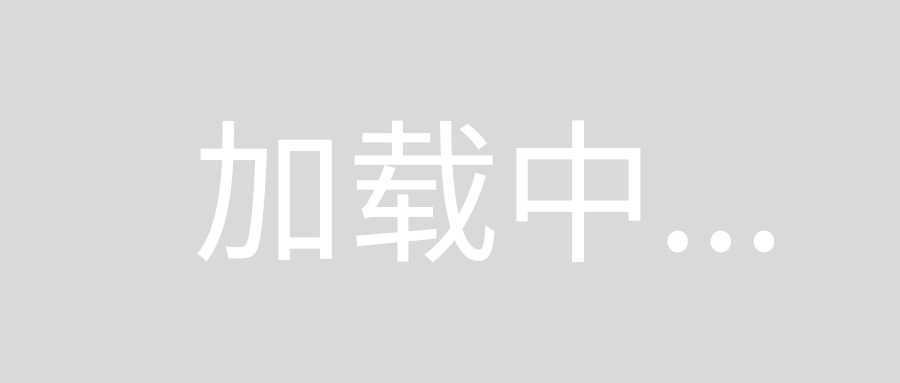
Here's what it looks like in Safari:
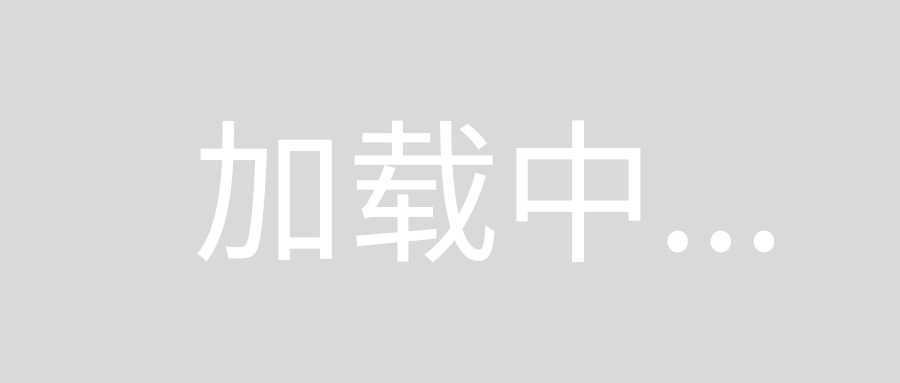
Here's my code:
#div1 {
background: black;
width: 250px;
height: 600px;
display: flex;
flex-direction: column;
padding-bottom: 5px
}
#div2 {
background: pink;
color: #FFF;
width: 240px;
height: 200px
}
#div3 {
background: blue;
color: #FFF;
width: 240px;
height: 100%;
position: relative
}
<div id="div1">
<div id="div2">test</div>
<div id="div3">test2</div>
</div>
The Problem
You have a container with height: 600px
.
This container has two children:
- One child has
height: 200px
.
- The other child has
height: 100%
.
Since percentage heights are based on the height of the parent, you are setting the height of the second child to equal the full height of the container.
10.5 Content height: the height
property
percentage
Specifies a percentage height. The percentage is calculated with respect to the height of the generated box's containing block.
As a result, an overflow occurs:
(200px + 600px) > 600px
Except that in a flex container, an initial setting is flex-shrink: 1
. This means that flex items can shrink in order to fit inside the container. Chrome and Firefox apply this setting correctly, allowing the element with height: 100%
to shrink to fit. Safari, apparently, has a different interpretation.
Solutions
You could use calc()
to solve the problem:
#div3 {
height: calc(100% - 200px);
}
#div1 {
background: black;
width: 250px;
height: 600px;
display: flex;
flex-direction: column;
padding-bottom: 5px
}
#div2 {
background: pink;
color: #FFF;
width: 240px;
height: 200px
}
#div3 {
background: blue;
color: #FFF;
width: 240px;
height: calc(100% - 200px);
}
<div id="div1">
<div id="div2">test</div>
<div id="div3">test2</div>
</div>
However, since you're already working in a column-direction flex container, you can use flex
to make the second child consume remaining space:
#div3 {
flex: 1;
}
This means: Any space not used up by other siblings will be consumed by this child.
#div1 {
background: black;
width: 250px;
height: 600px;
display: flex;
flex-direction: column;
padding-bottom: 5px
}
#div2 {
background: pink;
color: #FFF;
width: 240px;
height: 200px
}
#div3 {
background: blue;
color: #FFF;
width: 240px;
flex: 1;
}
<div id="div1">
<div id="div2">test</div>
<div id="div3">test2</div>
</div>