I need to write a function (in Javascript) that accepts the endpoints of a line segment and an additional point and returns coordinates for the point relative to the start point. So, based on this picture:
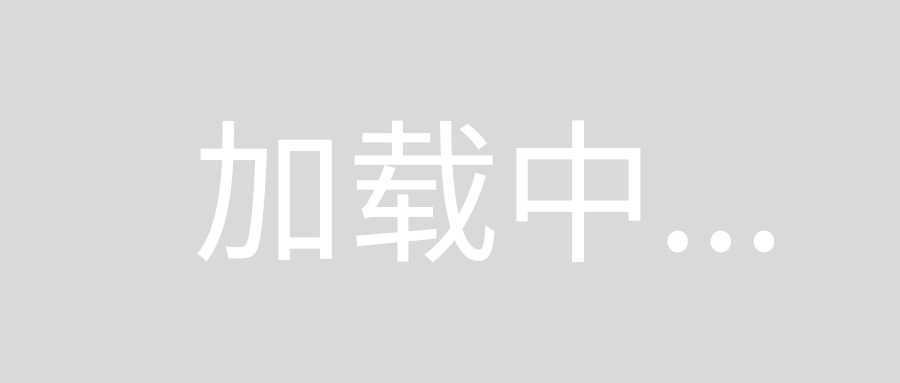
function perpendicular_coords(x1,y1, x2,y2, xp,yp)
returns [xp',yp']
where xp' is the distance from (xp,yp) along a line perpendicular to the line segment, and yp' is the distance from (x1,y1) to the point where that perpendicular line intersects the line segment.
What I've tried so far:
function rotateRad(cx, cy, x, y, radians) {
var cos = Math.cos(radians),
sin = Math.sin(radians),
nx = (cos * (x - cx)) + (sin * (y - cy)) + cx,
ny = (cos * (y - cy)) - (sin * (x - cx)) + cy;
return [nx, ny];
}
[xp', yp'] = rotateRad(x1,y1, xp, yp, Math.atan2(y2-y1,x2-x1));
I didn't write the function; got it from https://stackoverflow.com/a/17411276/1368860
I took a different approach, by combining two functions:
- To get yp' I find the intersection point using the answer from here: Perpendicular on a line from a given point
- To get xp' I calculate the distance between (xp, yp) and the line, using the equation from here: https://en.wikipedia.org/wiki/Distance_from_a_point_to_a_line
Not the most elegant solution, but it seems to work.
Resulting code https://jsfiddle.net/qke0m4mb/
function perpendicular_coords(x1, y1, x2, y2, xp, yp) {
var dx = x2 - x1,
dy = y2 - y1;
//find intersection point
var k = (dy * (xp-x1) - dx * (yp-y1)) / (dy*dy + dx*dx);
var x4 = xp - k * dy;
var y4 = yp + k * dx;
var ypt = Math.sqrt((y4-y1)*(y4-y1)+(x4-x1)*(x4-x1));
var xpt = distance(x1, y1, x2, y2, xp, yp);
return [xpt, ypt];
}
// Distance of point from line
function distance(x1, y1, x2, y2, xp, yp) {
var dx = x2 - x1;
var dy = y2 - y1;
return Math.abs(dy*xp - dx*yp + x2*y1 - y2*x1) / Math.sqrt(dy*dy + dx*dx);
}