You will notice the red "+" / "arrows" glyph in the attached screenshot. It is easy enough to change this "origin" point in Xcode. Is there also a way to do this programmatically or is this entirely an Xcode abstraction?
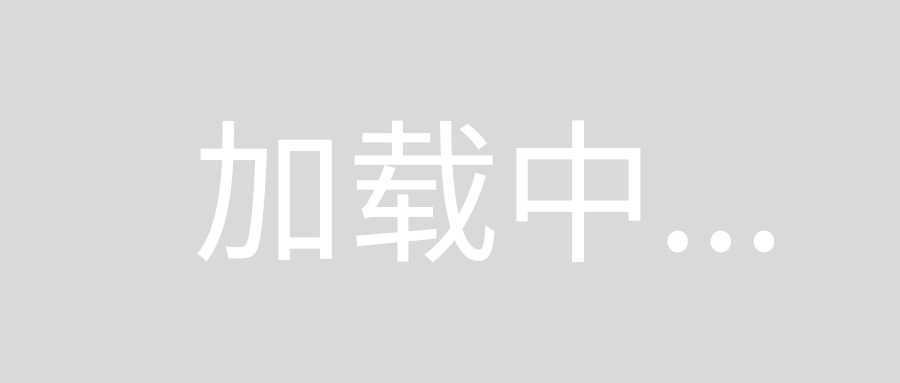
For instance, I want to programmatically create a UILabel and position it by calculating the lower right hand coordinate. In Xcode, I would simply make sure that the red "+" is on the bottom right grid point and define the X, Y, Width and Height parameters with that "origin" in mind.
If you're not using autolayout, you can position a label (or any view) in code by setting its center. So if you know where you want the label's lower right corner to be, you can just subtract half the width and height of the label to compute where its center should be:
CGPoint lowerRight = somePoint;
CGRect frame = label.frame;
label.center = CGPointMake(lowerRight.x - frame.size.width / 2,
lowerRight.y - frame.size.height / 2);
I would recommend just doing that.
But if you want, you can instead go to a lower level. Every view has a Core Animation layer, which is what actually manages the view's on-screen appearance. The layer has an anchorPoint
property, which by default is (0.5, 0.5), representing the center of the layer. You can set the anchorPoint
to (1, 1) for the lower-right corner:
label.layer.anchorPoint = CGPointMake(1, 1);
Now the label's center
actually controls the location of its lower right corner, so you can set it directly:
label.center = somePoint; // actually sets the lower right corner
You'll need to add the QuartzCore
framework to your target and import <QuartzCore/QuartzCore.h>
to modify the anchorPoint
property.
myObject.origin = CGPointMake (0.0,0.0);