I have 2 forms, formOrder and formOrderSummary.
On formOrder there are 3 buttons and a listView1.
btnVanilla, btnChocolate and btnNextPage and listview1
On formOrderSummary there is a listView2.
Each time Vanilla or Chocolate is clicked it will add it to the listview.
What I'm trying to do is get the listview1 on formOrder to show in listviewOrder
on formOrderSummary. Currently I got it working if the listview is in the same form but I can't seem to do it if the listview is in a different form.
There is a screenshot below which visualises what I mean
formOrder
Public Class formOrder
Dim frm2 As New formOrderSummary
Public vanillaCount As Integer
Public chocolateCount As Integer
Public mynumber As Double
Private Sub btnVanilla_Click(sender As Object, e As EventArgs) Handles btnVanilla.Click
Me.vanillaCount = Me.vanillaCount + 1
Dim str(3) As String
Dim item As ListViewItem
str(0) = "Vanilla"
str(1) = Me.vanillaCount.ToString()
mynumber = str(1) * 1
str(2) = mynumber.ToString("C")
Dim WholeString As String = str(0)
item = New ListViewItem(str)
For maindish As Integer = 0 To Me.ListView1.Items.Count - 1
If (Me.ListView1.Items(maindish).ToString = "ListViewItem: {" + WholeString + "}") Then
Me.ListView1.Items.RemoveAt(maindish)
Me.ListView1.Items.Add(item)
Return
End If
Next
Me.ListView1.Items.Add(item)
End Sub
Private Sub BtnChocolate_Click(sender As Object, e As EventArgs) Handles b btnChocolate.Click
Me.chocolateCount = Me.chocolateCount + 1
Dim str(3) As String
Dim item As ListViewItem
str(0) = "Chocolate"
str(1) = Me.chocolateCount.ToString()
mynumber = str(1) * 1.5
str(2) = mynumber.ToString("C")
Dim WholeString As String = str(0)
item = New ListViewItem(str)
For maindish As Integer = 0 To Me.ListView1.Items.Count - 1
If (Me.ListView1.Items(maindish).ToString = "ListViewItem: {" + WholeString + "}") Then
Me.ListView1.Items.RemoveAt(maindish)
Me.ListView1.Items.Add(item)
Return
End If
Next
Me.ListView1.Items.Add(item)
End Sub
Private Sub btnConfirm_Click(sender As Object, e As EventArgs) Handles btnConfirm.Click
frm2.Show()
Me.Hide()
End Sub
End Class
FormOrderSummary
Public Class formOrderSummary
Private Sub formOrderSummary_Load(sender As Object, e As EventArgs) Handles MyBase.Load
End Sub
End Class
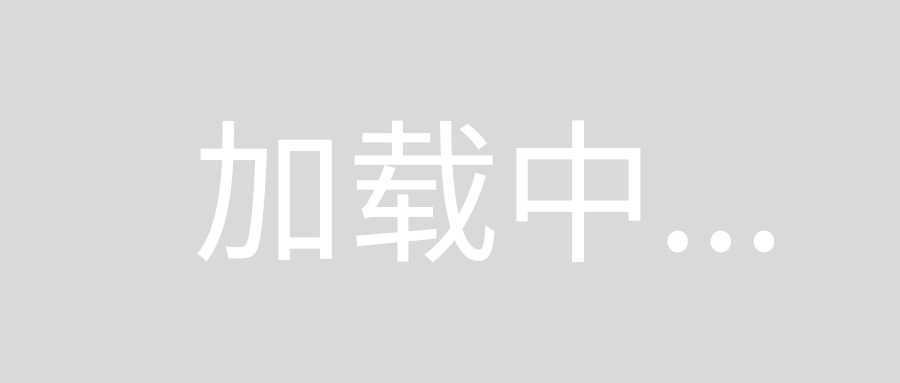
Forms are just classes, it says so at the top of all of them:
Public Class formOrder
so, you can add all the methods or properties you'd like. All you really need to do is create a method (sub) to pass the order to. The bigger issue is how do you pass 2 things (2 cones) in one method? What if the Ice Creame Shoppe offered waffle cones, sprinkles, flavored shells or other extras?
Wouldn't it be great if there was a way to keep the data together and organized? There is:
Public Class IceCream
Public Enum IceCreamFlavors
Vanilla
Chocolate
Strawberry
End Enum
Public Property Flavor As IceCreamFlavors
Public Property Sprinkles As Boolean
Public Property Scoops As Int32
Public Sub New()
Scoops = 1
End Sub
Public ReadOnly Property Cost As Decimal
Get
Return GetCost()
End Get
End Property
' just because I dont like a lot of code in getters
Private Function GetCost() As Decimal
Dim price As Decimal = 0
Select Case Flavor
Case IceCreamFlavors.Chocolate
price = 0.75D
Case IceCreamFlavors.Vanilla
price = 0.65D
Case IceCreamFlavors.Strawberry
price = 55D
' coming soon: Rocky Road!
End Select
Dim temp = (Scoops * price)
If Sprinkles Then
temp += 0.25D
End If
Return temp
End Function
End Class
Ok, that will hold the info for one cone. Notice how it contains everything related to a cone item, even pricing. It is easy to make it more complex such as WaffleCones, Chocolate shell etc without adding lots of loose variables in your form.
We also will need a way to hold several of them. I know what you are thinking: array. Dont go there. A List(of IceCream)
is even easier to use than an array.
Create Order
Private cones As New List(Of IceCream) ' will only hold icecream items
...
Dim item = New IceCream
item.Flavor = IceCream.IceCreamFlavors.Chocolate
item.Sprinkles = False
item.Scoops = 2
cones.Add(item)
The block creates a new Icecream
item, then sets the various attributes, then finally adds it to the list. Notice how I did not have to tell the List how big to be?
item = New IceCream
item.Flavor = IceCream.IceCreamFlavors.Vanilla
item.Sprinkles = True
cones.Add(item)
You access the info much the same way: Dim thisCost = item.Cost
The IceCream
class defaults to 1 scoop, so you only have to set that when it is not one scoop. You could add other defaults.
Pass the Data
Now, on the order summary form create a method to accept the data:
Public Sub SummaryData(order As List(Of IceCream))
' post items to listview
'e.g.: flavor = order(0).Flavor.ToString()
End Sub
If you want to add to it item by item, declare it to take one item:
Public Sub SummaryData(orderItem as IceCream)
(A problem will arise when you want to remove one). To pass the data:
frmSummaryINSTANCE.SummaryData(cones)
Tip: An even easier method is to use use a DataGridView.
dgv.DataSource = order
The DataGridView
will create the columns and display the property data automagically.
You could of course use this without a Class or List by changing the arguments, but it gets wordy and dense as the number and type of arguments increases.
Form Instances
Once you have the data organized properly it becomes pretty easy to do whatever you want. Note: This assumes you are using form instances. That is, rather than:
formOrderSummary.SummaryData(cones)
You do something like this:
Dim frmSumm As New formOrderSummary()
frmSumm.SummaryData(cones)
As noted, forms are classes and the proper way to use them is to create an instance of one (just like in C#). If you are not doing that now, it is something else to learn right away.
You might also want to see: Five Minute Guide to Classes and Lists