I'm using the new Android Studio, I'm looking for a way to use the support library from multiple projects. Basically I have a project that uses the ActionBarSherlock
this projects requires the support library. So I added a reference as like in this question.
Now I have the problem that my main project also uses the support library so I have it includes twice in some way. If I remove the library from one of both projects I'll get errors that some support library related classes are unknown which is clear for me, but if I have a reference in both projects I'll face this error:
Android Dex: [ProjectName] UNEXPECTED TOP-LEVEL EXCEPTION:
Android Dex: [ProjectName] java.lang.IllegalArgumentException: already added: Landroid/support/v4/app/ShareCompat$ShareCompatImplJB;
Android Dex: [ProjectName] at com.android.dx.dex.file.ClassDefsSection.add(ClassDefsSection.java:123)
Android Dex: [ProjectName] at com.android.dx.dex.file.DexFile.add(DexFile.java:163)
Android Dex: [ProjectName] at com.android.dx.command.dexer.Main.processClass(Main.java:490)
Android Dex: [ProjectName] at com.android.dx.command.dexer.Main.processFileBytes(Main.java:459)
...
Any idea how to fix this?
I fixed this by going into File > Project Structure...
and selecting Modules
then click on the module's Dependencies
tab. Then next to the library I changed the scope from Compile
to Provided
.
This means the module can still use it, but it doesn't reach compile, since I assume you are already using that JAR in your main project.
Hope this helps!
I had a similar issue happen to me. Select actionbarsherlock
in the project tab/bar/whatever (on the left, so you can see the project tree). hit F4
to open the module settings.
Make sure that you are not using the same dependencies twice in actionbarsherlock and your project.
Also make sure that you're using the version of the support library that's in the android-studio sdk (android-studio/sdk/extras/android
).
Finally, if you're like me, make sure you didn't accidentally make actionbarsherlock dependent on itself, thus loading the library twice THAT way! >.<
Take a look at these images showing my module preferences:
http://imgur.com/a/JupWp
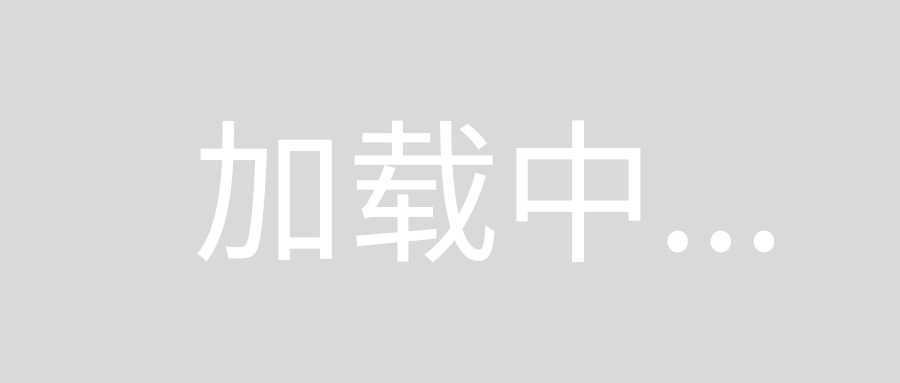
Also, don't forget to make sure libs
isn't hiding any of the same ones!
Did this use to be an Eclipse project? If so try going into Eclipse, clean the project, exit, rebuild in IntelliJ. I believe this is a state-issue, not a configuration issue.
Instead of importing android support library as jar, I changed the libraries (in my case facebook sdk) dependencies in build.gradle to:
dependencies {
compile 'com.android.support:support-v4:13.0.0'
}