Im new to ASP.NET MVC and want to do a simple page that retrieves some data using Entity and displays it in a paging datagrid.
Can anyone point me in the right direction or to a tutorial etc.
Its just a proof of concept for retrieving a list of stuff and displaying it.
For that you can use ASP.NET MVC jqGrid.
Below I have mentioned sample code for how to achieve that.
Sample Image
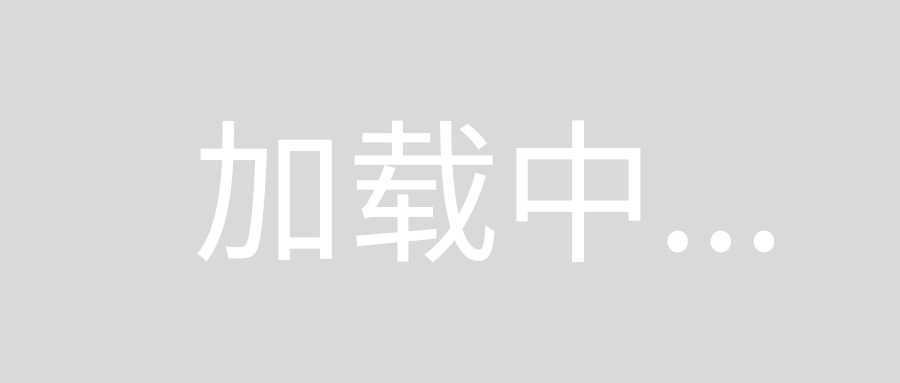
Action Method
public ActionResult JsonSalesCollection(DateTime startDate, DateTime endDate,
string sidx, string sord, int page, int rows)
{
SalesLogic logicLayer = new SalesLogic();
List<Sale> context;
// If we aren't filtering by date, return this month's contributions
if (startDate == DateTime.MinValue || endDate == DateTime.MinValue)
{
context = logicLayer.GetSales();
}
else // Filter by specified date range
{
context = logicLayer.GetSalesByDateRange(startDate, endDate);
}
// Calculate page index, total pages, etc. for jqGrid to us for paging
int pageIndex = Convert.ToInt32(page) - 1;
int pageSize = rows;
int totalRecords = context.Count();
int totalPages = (int)Math.Ceiling((float)totalRecords / (float)pageSize);
// Order the results based on the order passed into the method
string orderBy = string.Format("{0} {1}", sidx, sord);
var sales = context.AsQueryable()
.OrderBy(orderBy) // Uses System.Linq.Dynamic library for sorting
.Skip(pageIndex * pageSize)
.Take(pageSize);
// Format the data for the jqGrid
var jsonData = new
{
total = totalPages,
page = page,
records = totalRecords,
rows = (
from s in sales
select new
{
i = s.Id,
cell = new string[] {
s.Id.ToString(),
s.Quantity.ToString(),
s.Product,
s.Customer,
s.Date.ToShortDateString(),
s.Amount.ToString("c")
}
}).ToArray()
};
// Return the result in json
return Json(jsonData);
}
Jquery Set up
<script type="text/javascript">
var gridDataUrl = '/Home/JsonSalesCollection';
// use date.js to calculate the values for this month
var startDate = Date.parse('today').moveToFirstDayOfMonth();
var endDate = Date.parse('today');
jQuery("#list").jqGrid({
url: gridDataUrl + '?startDate=' + startDate.toJSONString() + '&endDate=' + endDate.toJSONString(),
datatype: "json",
mtype: 'GET',
colNames: ['Sale Id', 'Quantity', 'Product', 'Customer', 'Date', 'Amount'],
colModel: [
{ name: 'Id', index: 'Id', width: 50, align: 'left' },
{ name: 'Quantity', index: 'Quantity', width: 100, align: 'left' },
{ name: 'Product', index: 'Product', width: 100, align: 'left' },
{ name: 'Customer', index: 'Customer', width: 100, align: 'left' },
{ name: 'Date', index: 'Date', width: 100, align: 'left' },
{ name: 'Amount', index: 'Amount', width: 100, align: 'right'}],
rowNum: 20,
rowList: [10, 20, 30],
imgpath: gridimgpath,
height: 'auto',
width: '700',
pager: jQuery('#pager'),
sortname: 'Id',
viewrecords: true,
sortorder: "desc",
caption: "Sales"
});
</script>
You can get more details from GridView in ASP.NET MVC Here
OR
Check This Get the Most out of WebGrid in ASP.NET MVC (compatible with MVC 4)
I hope this will help to you.