code:
<?php
if(isset($_POST['save']))
{
$comment1 = $_POST['comment2'].",".date('Y-m-d');
$comment2 = $_POST['comment2'];
$id = $_POST['id'];
$query = "update enquires2 set comment1 = '$comment1', comment2 = '$comment2', s_date = '$s_datee' where id='$id'";
$result = mysqli_query($link,$query);
if($result==true)
{
echo "successfull";
}
else
{
echo "error!";
}
}
?>
<form method="post" name="myform">
<table>
<tr>
<th>comment1</th>
<th>comment2</th>
<th>Action</th>
</tr>
<?php
$sql = "select * from enquires2 ";
$result = mysqli_query($link,$sql);
while ($row = mysqli_fetch_array($result))
{
?>
<tr>
<td>
<input type='hidden' name='id' value='<?php echo $row['id']; ?>'>
</td>
<td>
<?php echo $row['comment1']; ?>
</td>
<td>
<input type='text' name='comment2' id='comment2' value=""/>
</td>
<td>
<input type ='submit' name='save' id='save' value='Save' />
</td>
</tr>
<?php
}
?>
</table>
</form>
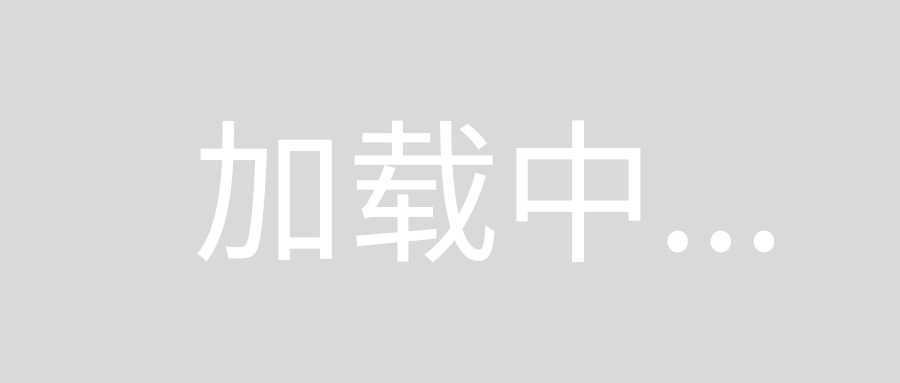
In this code I want to update table enquires2 with unique id. In following image you see that table row having save button this is only one row similarly it have multiple row which having save button in each row. Now I want that when I click on save button of particular row only that row data will be update. How can I fix this problem ? Please help.
Thank You
You could use AJAX and jQuery to do this and send the data to a separate PHP file and assigning the $row['ID']
to a data-value attribute of the button,
$("#save-btn").click(function(){
id = $(this).attr(data-value);
***** rest of values here
$.ajax({
method: "GET",
data: {id: id, rest of: data here},
url: phpfile.php,
success: function(){
console.log("Success");
}
})
});
While in the PHP file you would take get the id like,
$_GET['id']
, and same with the other values since we are using the GET method and then put them in the update query.
First of all, for security reason you need to change this query to a prepared statement see PHP MySQLI Prevent SQL Injection:
$id = $_POST['id'];
$query = "update enquires2 set comment1 = '$comment1', comment2 = $comment2', s_date = '$s_datee' where id='$id'";
$result = mysqli_query($link,$query);
This line is bad anyway, you are missing a opening quote for $comment2.
$query = "update enquires2 set comment1 = '$comment1', comment2 = $comment2', s_date = '$s_datee' where id='$id'";
Are you sure $link is an actual mysqli link?
As for the html part, you need to mkae one form for each record. See the link posted HTML: Is it possible to have a FORM tag in each TABLE ROW in a XHTML valid way?
alternatively you could do something bad like only adding the $id to evry field for every row (similar to:)
<input type ='submit' name='save[<?=$id;?>]' id='save' value='Save' />
and in the php code check witch key is set.
if(isset($_POST['save']) && is_array($_POST['save'])){
$id=key($_POST['save']);
}
You will need to replicate the bad thing for your comments as well but as a proof of concept you can run this snippet on phpfiddle.org
<?php
print_r($_POST);
if(isset($_POST['save']) && is_array($_POST['save'])){
echo key($_POST['save']);
}
?>
<html>
<form method='post'>
<input type='submit' name='save[1]' value='1' />
<input type='submit' name='save[2]' value='2' />
</form>
</html>
Wish i could provide you a really full answer but there's alot of work to be done on your code for it to be 'proper coding'. Again this becaome a matter of opinion beside the fact that your code is vunerable to sql injection and is NOT accepable.
Don't use your code at all for security vulnerability. Read more about sql injection Here. After all, For each row () create a form with a hidden input storing id of row .
I revised my code to make it work,create a nested table inside your td, so that tag will be accepted,
also see this link for a working reference,
HTML: Is it possible to have a FORM tag in each TABLE ROW in a XHTML valid way?
<?php
if(isset($_POST['save']))
{
$comment1 = $_POST['comment2'].",".date('Y-m-d');
$comment2 = $_POST['comment2'];
$id = $_POST['id'];
$query = "update enquires2 set comment1 = '$comment1', comment2 = '$comment2', s_date = '$s_datee' where id='$id'";
$result = mysqli_query($link,$query);
if($result==true)
{
echo "successfull";
}
else
{
echo "error!";
}
}
?>
<table>
<tr>
<th>comment1</th>
<th>comment2</th>
<th>Action</th>
</tr>
<?php
$sql = "select * from enquires2 ";
$result = mysqli_query($link,$sql);
while ($row = mysqli_fetch_array($result))
{
?>
<tr><td><table>
<form method="post" name="myform">
<tr>
<td>
<input type='hidden' name='id' value='<?php echo $row['id']; ?>'>
</td>
<td>
<?php echo $row['comment1']; ?>
</td>
<td>
<input type='text' name='comment2' id='comment2' value=""/>
</td>
<td>
<input type ='submit' name='save' id='save' value='Save' />
</td>
</tr>
</form>
</table>
</td>
</tr>
<?php
}
?>
</table>