So this is the PDF that my function calculates:
fx = 0.3 if (0<=x<1)
0.1 if (1<=x<2)
0.25 if (2<=x<3)
0.15 if (3<=x<4)
0.2 if (4<=x<5)
0 otherwise
And this is my coding for it:
fx = function(x)
{
if ((0<=x) & (x<1)) 0.3
else if ((1<=x) & (x<2)) 0.1
else if ((2<=x) & (x<3)) 0.25
else if ((3<=x) & (x<4)) 0.15
else if ((4<=x) & (x<5)) 0.2
else 0
}
Now how would I go about plotting y=fx?
I've tried:
x <- runif(n,0,5)
y <- fx(x)
plot(x, y, type='1', xlim=c(0,5), ylim=c(0,5))
But I get an error that 'x' and 'y' have differing lengths?
Your problems comes down to the fact your function isn't vectorized properly (it doesn't deal with a vector well).
If you use the accepted solution from your previous question about exactly the same problem then you won't have any issues
eg
# a solution that will work and be properly vectorized
fx <- function(x) c(0, 0.3,0.1,0.25,0.15,0.20, 0)[findInterval(x, c(-Inf, 0:5, Inf))]
x <- runif(n,0,5)
plot(x, fx(x))
If you want to plot a step function (which is what this pdf is), you can use stepfun
eg
fx <- stepfun(x = 0:5, y = c(0,0.3,0.1,0.25,0.15,0.20,0))
plot(fx, ylim = c(0,0.4),xlim = c(0,5), main = 'f(x)')
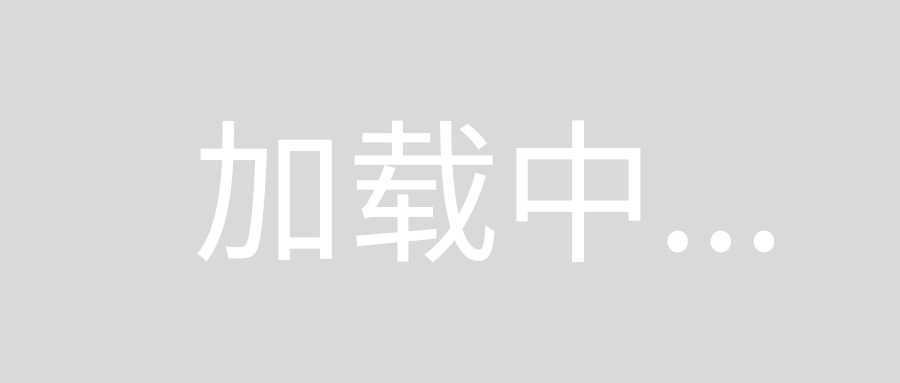
If you don't want the points added then
plot(fx, ylim = c(0,0.4),xlim = c(0,5), main = 'f(x)', do.points=FALSE)
If you want to vectorize a step function, then use Vectorize
vfx <- Vectorize(fx)
Your PDF is not vectorized. Try this:
fx <- function(x) {
ifelse((0<=x) & (x<1), 0.3,
ifelse((1<=x) & (x<2), 0.1,
ifelse((2<=x) & (x<3), 0.25,
ifelse((3<=x) & (x<4), 0.15,
ifelse((4<=x) & (x<5), 0.2,
0)))))
}
x <- seq(0, 6, length.out=n)
plot(x, fx(x))