可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
I have to following code for selecting layout on button click.
View.OnClickListener handler = new View.OnClickListener(){
public void onClick(View v) {
switch (v.getId()) {
case R.id.DownloadView:
// doStuff
setContentView(R.layout.main);
break;
case R.id.AppView:
// doStuff
setContentView(R.layout.app);
break;
}
}
};
findViewById(R.id.DownloadView).setOnClickListener(handler);
findViewById(R.id.AppView).setOnClickListener(handler);
When I click the "AppView" button, the layout changes, but when I click the "DownloadView "button, nothing happens.
This link says that I have to start a new activity.
But I don't know how to use the code there of intent to start new activity, will a new file be added?
EDIT:
I have my code on the new activity:
package com.example.engagiasync;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.TextView;
public class AppView extends Activity implements OnClickListener{
@Override
public void onCreate(Bundle savedInstanceState){
setContentView(R.layout.app);
TextView tv = (TextView) this.findViewById(R.id.thetext);
tv.setText("App View yo!?\n");
}
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
}
}
but it does not work, it force closes, the logcat says: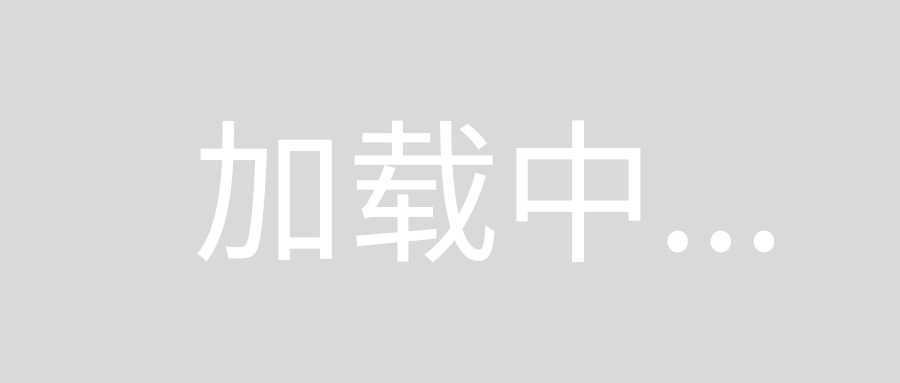
回答1:
Button btnDownload = (Button) findViewById(R.id.DownloadView);
Button btnApp = (Button) findViewById(R.id.AppView);
btnDownload.setOnClickListener(handler);
btnApp.setOnClickListener(handler);
View.OnClickListener handler = new View.OnClickListener(){
public void onClick(View v) {
if(v==btnDownload){
// doStuff
Intent intentMain = new Intent(CurrentActivity.this ,
SecondActivity.class);
CurrentActivity.this.startActivity(intentMain);
Log.i("Content "," Main layout ");
}
if(v==btnApp){
// doStuff
Intent intentApp = new Intent(CurrentActivity.this,
ThirdActivity.class);
CurrentActivity.this.startActivity(intentApp);
Log.i("Content "," App layout ");
}
}
};
Note : and then you should declare all your activities in the manifest .xml file like this :
<activity android:name=".SecondActivity" ></activity>
<activity android:name=".ThirdActivity" ></activity>
EDIT : update this part of Code :) :
@Override
public void onCreate(Bundle savedInstanceState){
super.onCreate(savedInstanceState);// Add THIS LINE
setContentView(R.layout.app);
TextView tv = (TextView) this.findViewById(R.id.thetext);
tv.setText("App View yo!?\n");
}
NB : check this (Broken link) Tutorial About How To Switch Between Activities.
回答2:
I would add an android:onClick
to the layout and then change the layout in the activity.
So in the layout
<ImageView
(Other things like source etc.)
android:onClick="changelayout"
/>
Then in the activity add the following:
public void changelayout(View view){
setContentView(R.layout.second_layout);
}
回答3:
I think what you're trying to do should be done with multiple Activities. If you're learning Android, understanding Activities is something you're going to have to tackle. Trying to write a whole app with just one Activity will end up being a lot more difficult. Read this article to get yourself started, then you should end up with something more like this:
View.OnClickListener handler = new View.OnClickListener(){
public void onClick(View v) {
switch (v.getId()) {
case R.id.DownloadView:
// doStuff
startActivity(new Intent(ThisActivity.this, DownloadActivity.class));
break;
case R.id.AppView:
// doStuff
startActivity(new Intent(ThisActivity.this, AppActivity.class));
break;
}
}
};
findViewById(R.id.DownloadView).setOnClickListener(handler);
findViewById(R.id.AppView).setOnClickListener(handler);
回答4:
I know I'm coming to this late, but what the heck.
I've got almost the exact same code as Kris, using just one Activity but with 2 different layouts/views, and I want to switch between the layouts at will.
As a test, I added 2 menu options, each one switches the view:
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()) {
case R.id.item1:
setContentView(R.layout.main);
return true;
case R.id.item2:
setContentView(R.layout.alternate);
return true;
default:
return super.onOptionsItemSelected(item);
}
}
Note, I've got one Activity class. This works perfectly. So I have no idea why people are suggesting using different Activities / Intents. Maybe someone can explain why my code works and Kris's didn't.
回答5:
You wanted to change the layout at runtime on button click. But that is not possible and as it has been rightly stated above, you need to restart the activity. You will come across a similar problem when u plan on changing the theme based on user's selection but it will not reflect in runtime. You will have to restart the activity.
回答6:
The logcat shows the error, you should call super.onCreate(savedInstanceState)
:
@Override
public void onCreate(Bundle savedInstanceState){
super.onCreate(savedInstanceState);
//... your code
}
回答7:
First I would suggest putting a Log in each case of your switch to be sure that your code is being called.
Then I would check that the layouts are actually different.
回答8:
It is very simple, just do this:
t4.setOnClickListener(new OnClickListener(){
@Override
public void onClick(View v) {
launchQuiz2(); // TODO Auto-generated method stub
}
private void launchQuiz2() {
Intent i = new Intent(MainActivity.this, Quiz2.class);
startActivity(i);
// TODO Auto-generated method stub
}
});