Well, I just can't increment a date with moment.js. I get a javascript date object in my code, wrap it into moment
function, calculate the amount of hours I need to add to the initial date and after I use .add
method nothing happens. Tried doing something like currentTime.add(2, 'hours')
, that didn't work as well. What am I doing incorrectly?
const currentTime = moment(ioc.get<Main.IArchiveManager>("ArchiveManager").getCurrentDate());
const speed = this.getData().speed;
const distance = this.calcDistanceFromPrevPoint(initialPoint,prevPoint);
const timeToReachPoint = (distance / speed) * 60;
const estimatedTime = currentTime.add(timeToReachPoint, 'hours');
debugger;
return estimatedTime;
this is a screenshot from my devtool so you know what is going on: 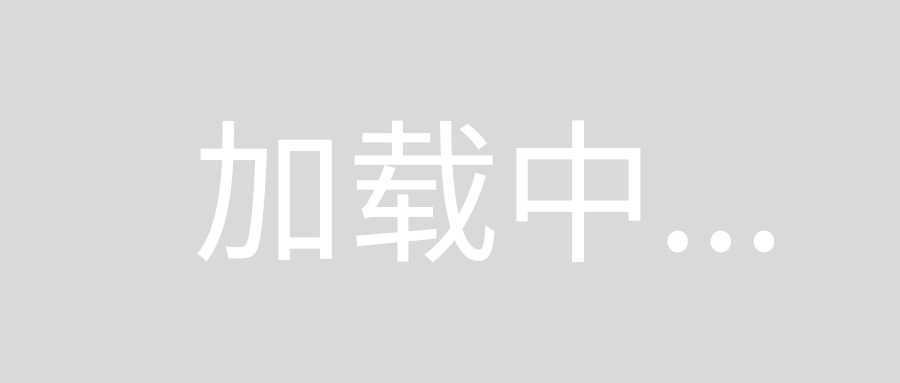
You have to use format()
(or .toString()
or .toISOString()
) to display the value of a moment object.
Note that:
- moment objects are mutable, so calling
add
will change the original object, if you need you can use the clone()
method
- Do not use Internal properties (prefixed with
_
)
Your code is fine, you are just logging moment object the wrong way:
const currentTime = moment();
console.log(currentTime.format())
const speed = 0.1//this.getData().speed;
const distance = 20.56;// this.calcDistanceFromPrevPoint(initialPoint,prevPoint);
const timeToReachPoint = (distance / speed) * 60;
const estimatedTime = currentTime.add(timeToReachPoint, 'hours');
console.log(estimatedTime.format())
<script src="https://cdnjs.cloudflare.com/ajax/libs/moment.js/2.18.1/moment.min.js"></script>
Everything is working as expected. You are logging the value of currentTime
after it got changed. Remember that .add()
changes the value of the object, it does not return a copy, but the object itself (for better chaining). See my example, you'll see that the came console.log, called twice but at different timings displays the values you'd expect.
var time = moment(new Date());
console.log(time);
time.add(2,'h');
console.log(time)
<script src="https://momentjs.com/downloads/moment.min.js"></script>