I want every row in my page to display 3 thumbnails, but its stacked in one row.
How do I manage the looping? Thank you...
<?php
foreach ($rows as $row){
?>
<div class="col-md-3">
<div class="thumbnail">
<img src="user_file/<?php echo $row->foto; ?>">
</div>
</div>
<?php
}
?>
This code generates stacked thumbnails in a row. How can I generate the row for every 3 columns?
This screenshot is what I got from the code:
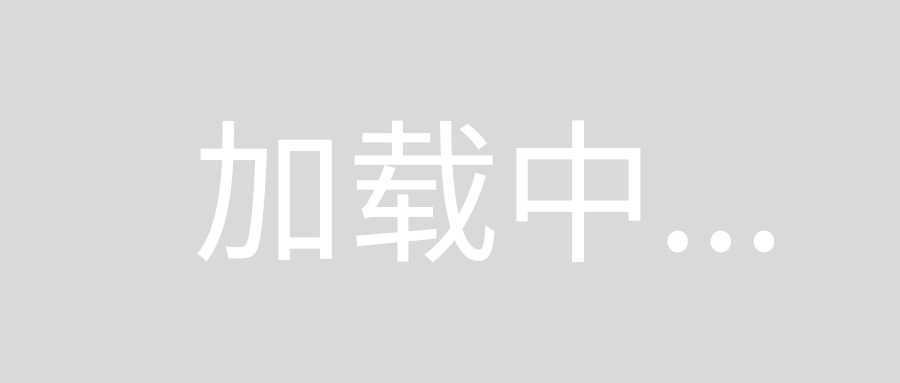
This is what I'm looking to get:
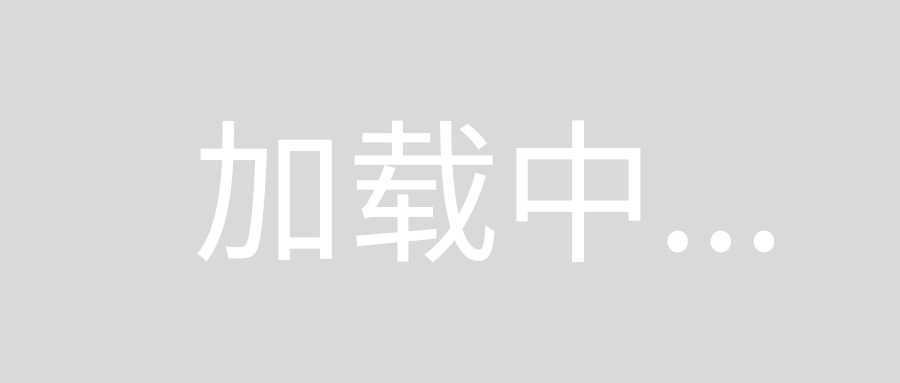
Edit: Originally i posted this quickly from the top of my head. Thanks Wael Assaf for pointing out an improvement, which I have used. Also I have added a couple of changes to the code, now it is versatile and can be used for variable number of columns you can choose by changing the variable $numOfCols
You need to add a div for each row. Then the floating divs you have, will not just wrap around but instead will be in a their own container.
The bootstrap class row
is perfect for this:
<?php
//Columns must be a factor of 12 (1,2,3,4,6,12)
$numOfCols = 4;
$rowCount = 0;
$bootstrapColWidth = 12 / $numOfCols;
?>
<div class="row">
<?php
foreach ($rows as $row){
?>
<div class="col-md-<?php echo $bootstrapColWidth; ?>">
<div class="thumbnail">
<img src="user_file/<?php echo $row->foto; ?>">
</div>
</div>
<?php
$rowCount++;
if($rowCount % $numOfCols == 0) echo '</div><div class="row">';
}
?>
</div>
Uses php modulus operator to echo the open and close of each row at the right points.
Hope this helps.
You can use array_chunk(input array, size of each chunk)
function to chunk your array into pieces.
php.net manual: array_chunk
Chunks an array into arrays with size elements. The last chunk may contain less than size elements.
Here is an example:
<?php
$numberOfColumns = 3;
$bootstrapColWidth = 12 / $numberOfColumns ;
$arrayChunks = array_chunk($items, $numberOfColumns);
foreach($arrayChunks as $items) {
echo '<div class="row">';
foreach($items as $item) {
echo '<div class="col-md-'.$bootstrapColWidth.'">';
// your item
echo '</div>';
}
echo '</div>';
}
?>
First You should define a variable, then right before the loop ends increment it and echo the closing row tag and open another one depending on it.
Useful steps
- define
$i = 0;
- inside the loop make your echos.
- right before the foreach ends increment the
$i++
and make a condition : if $i % 3 == 0
then echo the closing tag of the row then generate a new row.
Code :
<div class='row'>
<?php
foreach($items as $item) {
echo "<div class='col-lg-2'>";
echo "<div class='item'>";
echo 'Anythin';
echo '</div>';
echo '</div>';
$i++;
if ($i % 3 == 0) {echo '</div><div class="row">';}
}
?>
</div>
Tip : You don't really want to foreach the row, its a bad idea, make one row and foreach the items.
No need for all this complexity. Bootstrap works within a 12 col grid system automatically. Just make sure you loop and make col size so that that evenly divides by 12 e.g. col-md-4.
This example will provide 3 per row automatically since 12 / 4 = 3.
<div class="row">
LOOPCODE
{
<div class="col-md-4">
DATA
</div>
}
</div>
This is a better way – to use chunk() function of Collections.
`@foreach($items->chunk(5) as $chunk)
<ul>
@foreach($chunk as $item)
Item {{ $loop->iteration }}
@endforeach
</ul>
@endforeach`