I've looked some time for a good explanation about the association between ASP.NET (MVC/WebAPI) as server-side and AngularJS as the client. But I'm still uncertain whether is it the correct way to implement a SPA with both technologies.
My idea is, to use ASP.NET as REST service. But I'm impressed about the Bundle in ASP.NET. To use it I need ASP.NET MVC and its Razor templates. Is that a good practice for building an application with angularjs as client?
I guess I only need MVC for authorization if the user is allowed to go on relevant pages. Or is there a better way with angular to find out the windows user? Because I need the account of the windows login user.
I hope anyone can help me to find out the right way.
The stack you're suggesting is perfectly good to use.
My team has built an application in the past where we had 2 razor pages, the one was basically the external app (by external I mean non-logged in user) which was quite light weight and only handled signup, then the other acted as an internal shell for the rest of the app (once logged in).
- The .NET bundling allowed us to do some cool things like only delivering certain scripts to certain razor pages. EG a non-logged in user didn't need to be served the whole internal app.
- Using this method also allows you to utilize the built in ASP.NET auth framework.
I recently stumbled across a really cool bootstrap project that can help you construct a boilerplate app. My suggestion is just download this, and have a look through the boilerplate app to give you more clarity on its usage. (Then it's up to you if you want to use it or not, but it'll give you more clarity on solution construction)
Thus your final app would have one (or 2) MVC controller(s) that deliver your shell pages, and all other calls would be done via WebAPI endpoints. See rough example below:
..\Controllers\RootController.cs
public class RootController : Controller
{
// we only utilize one server side rendered page to deliver out initial
// payloads; scripts; css; etc.
public ActionResult Index()
{
// using partial view allows you to get rid of the _layout.cshtml page
return PartialView();
}
}
..\Views\Root\Index.cshtml
<html ng-app="MyAngularApp">
<head>
@Styles.Render("~/content/css")
@Scripts.Render("~/bundles/js")
@Scripts.Render("~/bundles/app")
</head>
<body>
<div class="container">
<div ng-view></div>
</div>
</body>
</html>
..\WebAPI\SomethingController.cs
public class SomethingController : ApiController
{
private readonly IRepository _repo;
public SomethingController(IRepository repo)
{
_repo = repo;
}
// GET: api/Somethings
public IQueryable<Something> GetSomethings()
{
return _repo.GetAll();
}
}
Tip:
The easiest way I've found to construct projects like this is to use the Visual Studio's New Project Wizard. Select Web Project -> MVC
, Make sure to Select WebAPI
. This will ensure that App_Start has all the required routes & configs pre-generated.
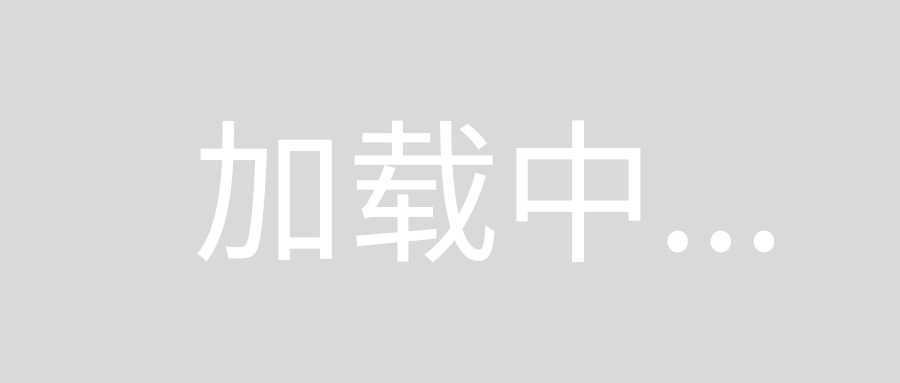
I assume you are using Angular 1.x. I don't see any constraints in using both razor and the template provided by angular, but generally you'd be better off in sticking with angular's template system. Here's some pointers:
- You'll use 2 razor views - _Layout.cshtml and Index.cshtml. These 2 will bootstrap the application.
- You'll need at least an MVC home controller that maps to your Index.cshtml.
- You'll need to configure your MVC routes to all point to your home controler using catch-all. Refer to this link for more details (go to RouteConfig.cs section of the article)
- For bundling javascript you can use MVC's bundling and minification and use the @Scripts.Render in your MVC view. There will be a performance penalty in the initial load since you'll be loading the minified scripts all at the same time. If your app is too large then you can use something like require js. Here's some links for a more detailed explanation:
Bundling and Minifying an AngularJS Application with ASP.NET MVC
Bundling and Minifying an AngularJS Application with ASP.NET MVC
- For authentication you can use Token-Based authentication here's a link for configuring it.