Can you please let me know how I can get the total and current number of the carousel slides in bootstrap like the image below?
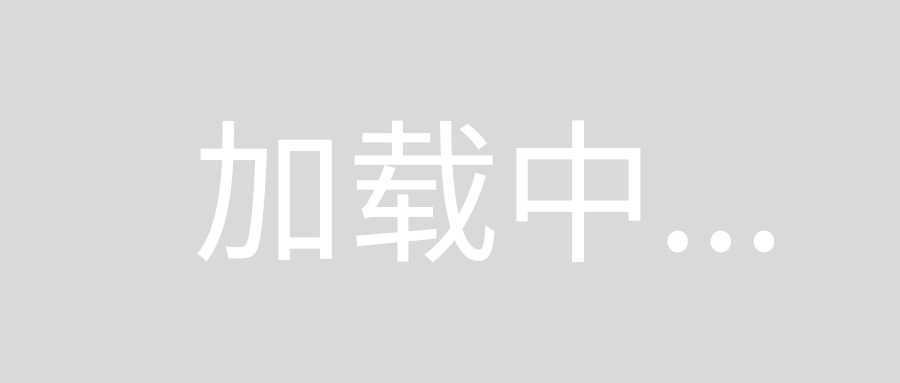
I have an standard Bootstrap carousel and a <div>
with the .num
class to display the total and current number and I used this code to retrieve the numbers but it didn't go through
$('.num').html(){
$('#myCarousel').carousel({number})
}
Thanks
Update:
Please find a sample at this jsfiddle LINK
Each slide
has a .item
class to it, you can get the total number of slides like this
var totalItems = $('.item').length;
Active slide
has a class named as active
, you can get the index of active slide
like this
var currentIndex = $('div.active').index() + 1;
You can update these values by binding the bootstrap carousel slid
event like this
$('#myCarousel').bind('slid', function() {
currentIndex = $('div.active').index() + 1;
$('.num').html(''+currentIndex+'/'+totalItems+'');
});
EXAMPLE
Update of @Khawer Zeshan's code
For Bootstrap 3.0+ use slid.bs.carousel
instead of slid
and on
instead of bind
. So the update code will be like that
var totalItems = $('.item').length;
var currentIndex = $('div.active').index() + 1;
$('#myCarousel').on('slid.bs.carousel', function() {
currentIndex = $('div.active').index() + 1;
$('.num').html(''+currentIndex+'/'+totalItems+'');
});
var totalItemsPop = $('#Mycarousel .item').length;
$('#Mycarousel').on('slide.bs.carousel', function() {
setTimeout(function(){
currentIndexPop = $('#Mycarousel div.active').index() + 1;
$('.num').html('' + currentIndexPop + '/' + totalItemsPop + '');
}, 1000);
});
after slide event div will be active and can not get active index, so keep you code inside set time out function
Bootstrap 3.2+:
carouselData.getItemIndex(carouselData.$element.find('.item.active'))
You can use jquery index() function to get the current index of active element inside list of item. So the code is look like this:
var currentItem = $("#carousel-1 .item.active" );
var currentIndex = $('#carousel-1 .item').index(currentItem) + 1;
The only drawback with the below code will be that it doesn't show up till you go to next slide (triggers after each slide change).
JS/jQuery
$('#carousel-slide').on('slid.bs.carousel', function () {
var carouselData = $(this).data('bs.carousel');
var currentIndex = carouselData.getActiveIndex();
var total = carouselData.$items.length;
var text = (currentIndex + 1) + " of " + total;
$('#carousel-index').text(text);
});
HTML
<div id="carousel-index">
<!-- Number will be added through JS over here -->
</div>