I have a problem with jQuery flot.
PHP output (not JSON):
[[1, 153], [2, 513], [3, 644]] ~~ [[1, 1553], [2, 1903], [3, 2680]]
jQuery call:
$.ajax({
url: 'xxx.php',
success: function (data) {
var dataArray = data.split('~~'),
dataArray1 = dataArray[0],
dataArray2 = dataArray[1],
plot = $.plot($('#xxx'), [{
data: dataArray1,
color: colours[0]
},
{
data: dataArray2,
color: colours[1],
points: {
show: true,
}
},
], {
series: {
bars: {
show: true,
barWidth: .6,
align: 'center'
}
},
grid: {
show: true,
hoverable: true,
clickable: true,
autoHighlight: true,
borderWidth: true,
borderColor: 'rgba(255, 255, 255, 0)'
},
xaxis: {
show: false
}
});
}
});
Taking the data in this way, I'm trying to use jQuery Flot but does not work...
Whereas, I can by separating data:
First label:
[[1, 153], [2, 513], [3, 644]]
Second label:
[[1, 1553], [2, 1903], [3, 2680]]
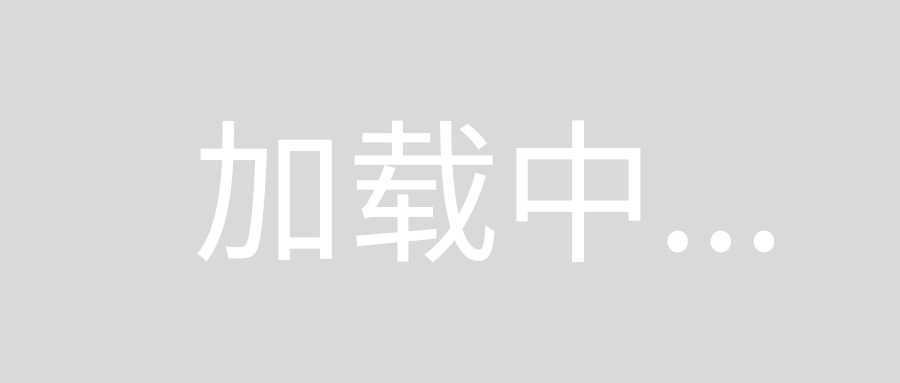
I'll share a Simple example jquery Flot with ajax for basic understanding purpose.
See this page and let change it into ajax : http://www.jqueryflottutorial.com/making-first-jquery-flot-line-chart.html
First, you must successful showing the chart as described without ajax. Don't forget to put height and width in div tag if you don't include css file.:
<div id="flot-placeholder" style="width: 100%; height: 260px"></div>
If ok, then follow this step.
STEP 1 : Put the script inside a function:
<script>
function show_chart(data) {
// this will be moved to php file
//var data = [[1, 130], [2, 40], [3, 80], [4, 160], [5, 159], [6, 370], [7, 330], [8, 350], [9, 370], [10, 400], [11, 330], [12, 350]];
var dataset = [{label: "line1",data: data}];
var options = {
series: {
lines: { show: true },
points: {
radius: 3,
show: true
}
}
};
$(document).ready(function () {
$.plot($("#flot-placeholder"), dataset, options);
});
}
</script>
STEP 2 : Create sample.php.
<?php
require 'config.php';
if($_POST)
{
$id = $_POST['id'];
$arr = array();
$arr = [[1, 130], [2, 40], [3, 80], [4, 160], [5, 159], [6, 370], [7, 330], [8, 350], [9, 370], [10, 400], [11, 330], [12, 350]];
echo json_encode($arr);
}?>
Note : $arr that moved from the first script then become only a sample data. You should make a php class or function that fetch data from database and return as array format as shown in $arr.
STEP 3 : Create simple ajax to get the response and render the chart :
var id = 1;
$.post('/..if any folder../sample.php', {
id : id,
}, function(response){
var data = JSON.parse(response);
show_chart(data); // call function and render the chart in <div id="flot-placeholder"></div>
});
Step Finish.
In some cases, we may need two or more data type. Then just add this to the code :
inside sample.php :
$arr1 = array();
$arr2 = array();
$arr1 = [[1, 130], [2, 40], [3, 80], [4, 160], [5, 159], [6, 370], [7, 330], [8, 350], [9, 370], [10, 400], [11, 330], [12, 350]];
$arr2 = [[1, 130], [2, 40], [3, 80], [4, 160], [5, 159], [6, 370], [7, 330], [8, 350], [9, 370], [10, 400], [11, 330], [12, 350]];
// put more if rquired
echo json_encode($arr1,$arr2); // put more array if required
inside ajax :
var data = JSON.parse(response);
var result1 = data[0]; // response data from $arr1
var result2 = data[1]; // response data from $arr2
Sorry for long description. Hope it will help.
Just for fun :
Some people don't want to show 'sample.php' in the console log. For this purpose we can simply change 'sample' as a folder and create index.php inside it and in the ajax we just direct the url to the folder like this :
$.post('/..if any folder../sample/'), { // this will open index.php