可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
I am trying to change the height of the stock UITabBar to 44px, similar to Tweetbot's tab bar height. I've also seen a few other apps do this as well.
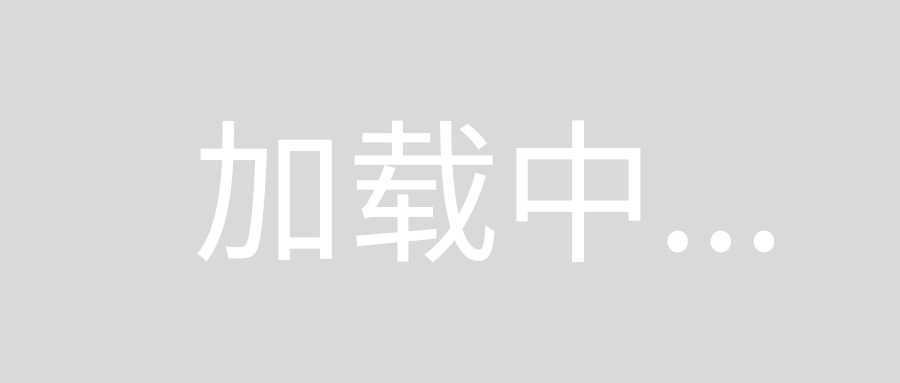
however, when i try to set the height it still remains the same
self.tabBar.frame.height = 40
are we not allowed to change the tab bar height? and if so what is a good alternative? using a toolbar?
回答1:
SomeGuy's answer above worked for me. Here's the Swift translation for anyone who may need it. I made the height close to what it seems most popular apps use.
class TabBar: UITabBar {
override func sizeThatFits(size: CGSize) -> CGSize {
var sizeThatFits = super.sizeThatFits(size)
sizeThatFits.height = 38
return sizeThatFits
}
}
回答2:
It seems everybody says this can't be done easily
In your storyboard give your UITabBar a custom subclass name, then implement the subclass with the following
This tells all views that use the tab bar that it should be a certain height.
@implementation MyTabBar
-(CGSize)sizeThatFits:(CGSize)size
{
CGSize sizeThatFits = [super sizeThatFits:size];
sizeThatFits.height = 100;
return sizeThatFits;
}
@end
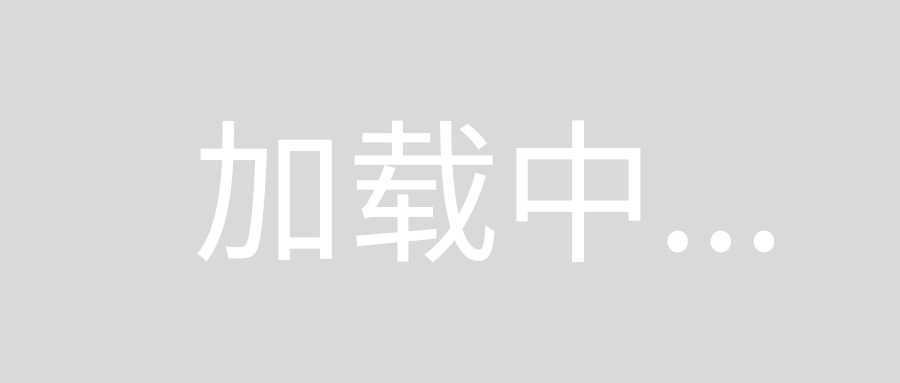
回答3:
For Swift 3 and xcode 8
extension UITabBar {
override open func sizeThatFits(_ size: CGSize) -> CGSize {
var sizeThatFits = super.sizeThatFits(size)
sizeThatFits.height = 80 // adjust your size here
return sizeThatFits
}
}
回答4:
In your UITabBarController
- (void)viewWillLayoutSubviews {
CGRect tabFrame = self.tabBar.frame;
tabFrame.size.height = 80;
tabFrame.origin.y = self.view.frame.size.height - 80;
self.tabBar.frame = tabFrame;
}
回答5:
In swift it is even simpler than all solutions suggested above by using an extensions to UITabBar, no subclassing necessary:
extension UITabBar {
override public func sizeThatFits(size: CGSize) -> CGSize {
super.sizeThatFits(size)
var sizeThatFits = super.sizeThatFits(size)
sizeThatFits.height = <Insert your height here>
return sizeThatFits
}
}
回答6:
If you have auto layout enabled, you will need to override instrinsicContentSize instead
Proper usage of intrinsicContentSize and sizeThatFits: on UIView Subclass with autolayout
class TabBar: UITabBar {
override func intrinsicContentSize() -> CGSize {
var intrinsicSize = super.frame.size
intrinsicSize.height = 120
return intrinsicSize
}
}
回答7:
The developer doesn't own the tabBar, the framework does. It will fight you to make sure that the tabBar stays the same height. If you want to work around this, you can make your own toolbar and add autlayout constraints to its height to force it to stay whatever height you'd like.
回答8:
If you are on iOS 11 then following will help
-(CGSize)sizeThatFits:(CGSize)size
{
CGSize sizeThatFits = [super sizeThatFits:size];
sizeThatFits.height = 60;
if (@available(iOS 11.0, *)) {
UIWindow *window = UIApplication.sharedApplication.keyWindow;
CGFloat bottomPadding = window.safeAreaInsets.bottom;
sizeThatFits.height += bottomPadding;
}
return sizeThatFits;
}
Basically need to cover safe area, else tabbar height on iPhone X appears to be low.