i am trying to add a gif in the back of my code up i cant seem it get it working here is my code i am working wiht
import sys
import webbrowser
import random
import time
import os
import subprocess
from PyQt4.QtCore import QSize, QTimer
from PyQt4.QtGui import QApplication, QMainWindow, QPushButton, QWidget, QIcon, QLabel, QPainter, QPixmap, QMessageBox, QAction, QKeySequence, QFont, QFontMetrics, QMovie
from PyQt4 import QtGui
class UIWindow(QWidget):
def __init__(self, parent=None):
super(UIWindow, self).__init__(parent)
self.resize(QSize(600, 750))
self.ToolsBTN = QPushButton('tab', self)
self.ToolsBTN.resize(100,40)
self.ToolsBTN.move(60, 300)
self.CPS = QPushButton('tab1', self)
self.CPS.resize(100,40)
self.CPS.move(130,600)
self.Creator = QPushButton('tab2', self)
self.Creator.resize(100,40)
self.Creator.move(260, 50)
class MainWindow(QMainWindow,):
def __init__(self, parent=None):
super(MainWindow, self).__init__(parent)
self.setGeometry(50, 50, 600, 750)
self.setFixedSize(600, 750)
self.startUIWindow()
def startUIWindow(self):
self.Window = UIWindow(self)
self.setWindowTitle("My Program")
self.show()
if __name__ == '__main__':
app = QApplication(sys.argv)
w = MainWindow()
sys.exit(app.exec_())
so that is all i have right now and i am trying to get my Dance.gif to play in the back but its not working for me if someone could help me out with this that would be amazing!
You must reproduce the movie using QMovie, and every time it changes image you must refresh the background with the current pixmap.
add the next code in __ init __
function:
self.movie = QMovie("{filename}.gif")
self.movie.frameChanged.connect(self.repaint)
self.movie.start()
And we implemented the paintEvent function:
def paintEvent(self, event):
currentFrame = self.movie.currentPixmap()
frameRect = currentFrame.rect()
frameRect.moveCenter(self.rect().center())
if frameRect.intersects(event.rect()):
painter = QPainter(self)
painter.drawPixmap(frameRect.left(), frameRect.top(), currentFrame)
Complete code:
import sys
from PyQt5.QtCore import QSize
from PyQt5.QtGui import QMovie, QPainter, QPixmap
from PyQt5.QtWidgets import QApplication, QMainWindow, QPushButton, QWidget
class UIWindow(QWidget):
def __init__(self, parent=None):
super(UIWindow, self).__init__(parent)
self.resize(QSize(600, 750))
self.ToolsBTN = QPushButton('tab', self)
self.ToolsBTN.resize(100, 40)
self.ToolsBTN.move(60, 300)
self.CPS = QPushButton('tab1', self)
self.CPS.resize(100, 40)
self.CPS.move(130, 600)
self.Creator = QPushButton('tab2', self)
self.Creator.resize(100, 40)
self.Creator.move(260, 50)
class MainWindow(QMainWindow):
def __init__(self, parent=None):
super(MainWindow, self).__init__(parent)
self.setGeometry(50, 50, 600, 750)
self.setFixedSize(600, 750)
self.startUIWindow()
self.movie = QMovie("animated-dancing-image-0028.gif")
self.movie.frameChanged.connect(self.repaint)
self.movie.start()
def startUIWindow(self):
self.Window = UIWindow(self)
self.setWindowTitle("My Program")
self.show()
def paintEvent(self, event):
currentFrame = self.movie.currentPixmap()
frameRect = currentFrame.rect()
frameRect.moveCenter(self.rect().center())
if frameRect.intersects(event.rect()):
painter = QPainter(self)
painter.drawPixmap(frameRect.left(), frameRect.top(), currentFrame)
if __name__ == '__main__':
app = QApplication(sys.argv)
w = MainWindow()
sys.exit(app.exec_())
GIF:
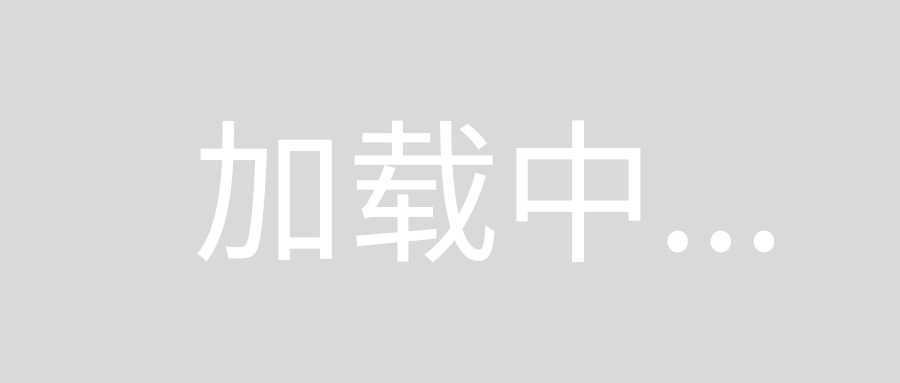
Output:
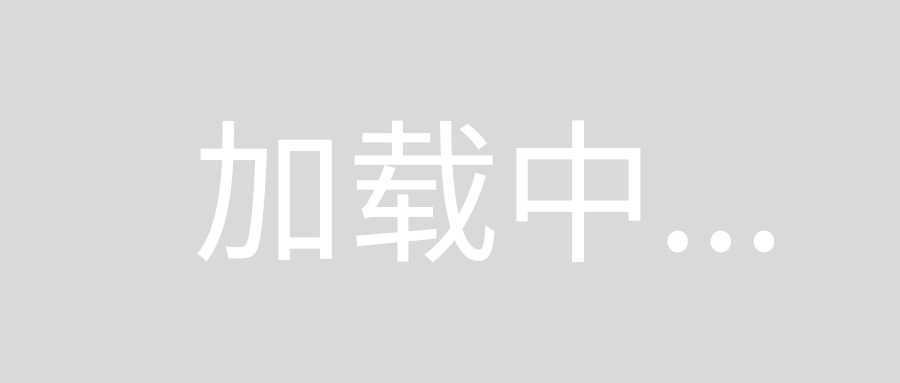
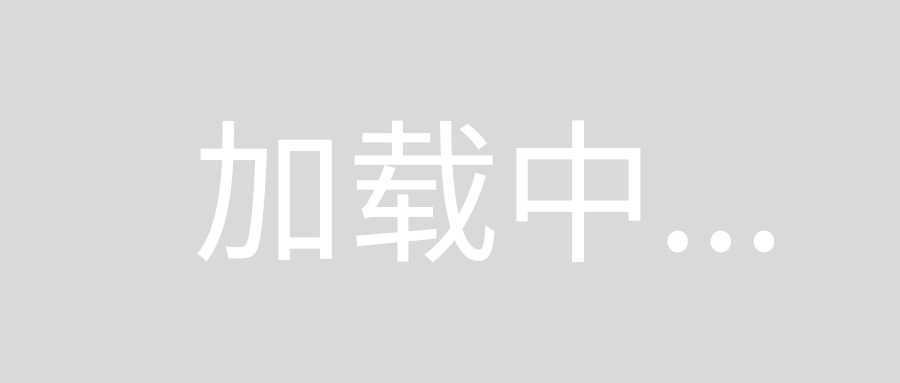
I achieved this using this class...
class backgroundView(QGraphicsView):
def __init__ (self,movie):
super(backgroundView,self).__init__()
self.movie=movie
self.display_pixmap=movie.currentPixmap()
self.setStyleSheet('QGraphicsView {background-color: rgb(0,0,0);}')
def paintEvent(self,event):
self.display_pixmap = self.movie.currentPixmap().scaled(self.my_size)
painter=QPainter()
painter.begin(self.viewport())
painter.fillRect(event.rect(),self.palette().color(QPalette.Window))
x = (self.width() - self.display_pixmap.width())/2
y = (self.height() - self.display_pixmap.height())/2
painter.drawPixmap(x, y, self.display_pixmap)
painter.end()
def resizeEvent(self, event):
self.my_size=event.size()
Then the main window class looks something like this...
class MainWindow(QMainWindow):
def __init__(self):
view=backgroundView(self.movie)
mainLayout=QVBoxLayout()
self.setCentralWidget(view)
view.setLayout(mainLayout)
button=QPushButton('Button')
mainLayout.addWidget(button)
self.movie.frameChanged.connect(view.update)
self.movie.start()
self.show()
This will fill the background with you GIF and also scale it to the size of the window.
I adapted this from a class I have been using for background images that I found here. I don't know why, but it wont repaint unless you set the style sheet of the view.