I am trying to import a Typeahead
component and getting
cannot find a module 'ng2-typeahead'
error.
I have ASP.NET Core application, downloaded this on from here :
https://www.npmjs.com/package/generator-aspnetcore-angular2
and using tutorial from here for Typeahead
:
https://www.npmjs.com/package/ng2-typeahead
What I did:
- Added in package.json:
"ng2-typeahead": "1.0.0",
Added code in system.congfig.js
:
paths: {
// paths serve as alias
'npm:': 'node_modules/'
},
var map = {
....
'ng2-typeahead': 'npm:ng2-typeahead',
};
var packages = {
'ng2-typeahead': { main: 'ng2-typeahead.js', defaultExtension: 'js' }
};
Getting error by importing Typeahead
in app.module.ts
file:
import { Typeahead } from 'ng2-typeahead';
Am i doing something wrong or missing something ? Thanks for any help.
You can try this workaround-
First, Copy ng2-typeahead.ts into your app folder.
In app.module.ts, import from ng2-Typeahead like this-
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
import { AppComponent } from './app.component';
import { Typeahead } from './ng2-Typeahead';
//import { Typeahead } from 'ng2-Typeahead';
@NgModule({
imports: [ BrowserModule, FormsModule ],
declarations: [ AppComponent, Typeahead ],
bootstrap: [ AppComponent ]
})
export class AppModule { }
Use Typeahead in your component like this-
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
template: `<h1>My First Angular App</h1>
<typeahead
[(ngModel)]="selectedFruit"
[list]="fruits"
[searchProperty]="'searchText'" [displayProperty]="'name'"
[maxSuggestions]="2"
(suggestionSelected)="fruitSelected($event)"
placeholder="Begin typing a fruit">
</typeahead>
`
})
export class AppComponent {
fruits: any[] = [
{
id: 1,
name: "1 - Apple",
searchText: "apple"
},
{
id: 2,
name: "2 - Orange",
searchText: "orange"
},
{
id: 3,
name: "3 - Banana",
searchText: "banana"
}
];
selectedFruit: any = this.fruits[0];
public fruitSelected(fruit) {
this.selectedFruit = fruit;
}
}
Output result:
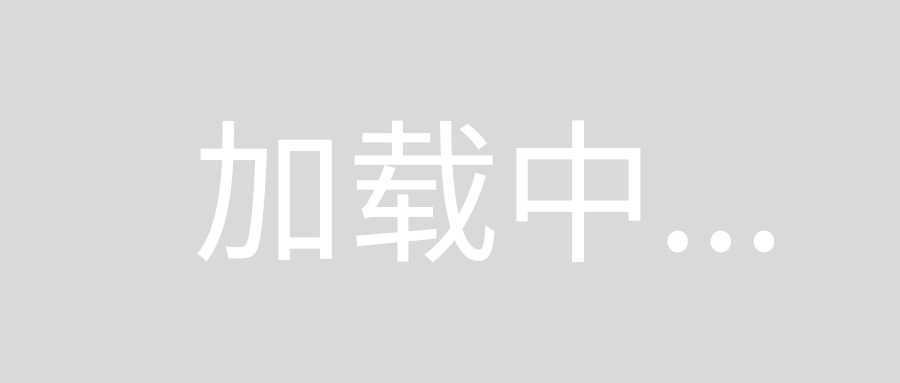
See if this helps.