I came across this design image online and I am really puzzled on how could I make a UITableCell that has multiple subtitles and allows me to customised them in the way shown by the picture.
My understanding is that one can only use 1 subtitle per cell.
Is there a way to create a UITable cell that looks like that? How would you go on to make those 4 subtitles under the cell title???
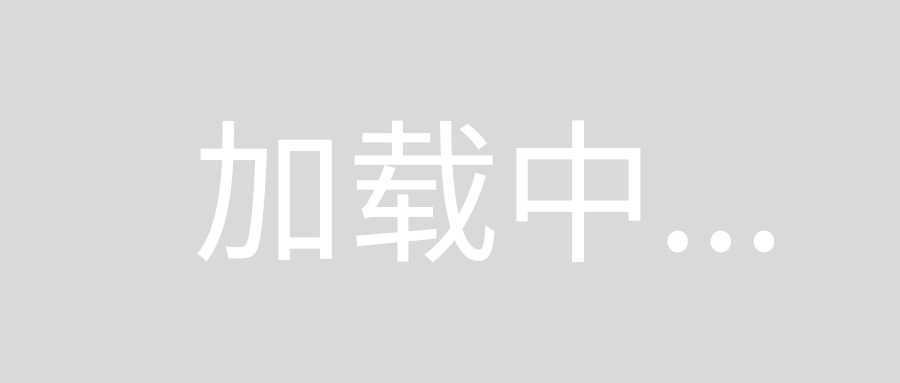
You could do that easily by having a custom layout for the UITableViewCell. This video should help you in doing this:
http://www.youtube.com/watch?v=d_kO-J3DYvc
Basically you will need to design the UI of the cell in storyboard/NIB file and add multiple labels to your table cell there. Sub-class UITableViewCell and link it to the designed UITableViewCell in storyboard/NIB. Addd IBOulets in that class for the labels and link your labels from the UI to these IBOutlets.
From the image provided, it looks like the prototype UITableViewCell
contains one UIImageView
and 5 UILabel
s. Assuming you are using IB or storyboard to create the table view cell, set the 'Table View Style' to Custom, than drag a UIImageView
and 5 UILabel
s onto the prototype cell. For each of the UILabel
s, adjust their position, font and font size as desired. You may also need to adjust the height of the cell.
Just subclass UITableViewCell, and add in multiple UILabel's. Then override the layoutSubviews method to position those labels. Then in the cellForRow, make sure you instantiate your subclassed UITableViewCell. I don't have time to check this, but the subclass would look something like:
@interface CustomCell : UITableViewCell {
UILabel *myCustomLabel1;
}
@end
@implementation CustomCell
- (id)initWithFrame:(CGRect)frame reuseIdentifier:(NSString *)reuseIdentifier {
if (self = [super initWithFrame:frame reuseIdentifier:reuseIdentifier]) {
// Initialization code
myCustomLabel1 = [[UILabel alloc] initWithFrame:CGRectZero];
[self.contentView addSubview:myCustomLabel1];
}
return self;
}
-(void)layoutSubviews
{
[super layoutSubviews];
float margin = 5;
[myCustomLabel1 setFrame:CGRectMake(self.bounds.origin.x+margin, self.bounds.origin.y, self.bounds.size.width - (2*margin), self.bounds.size.height)];
}
@end
Hey I have created a Sample Project regarding Custom Cell check this github link that I have created. I have used storyboard.
here is the screenshot of the sample app
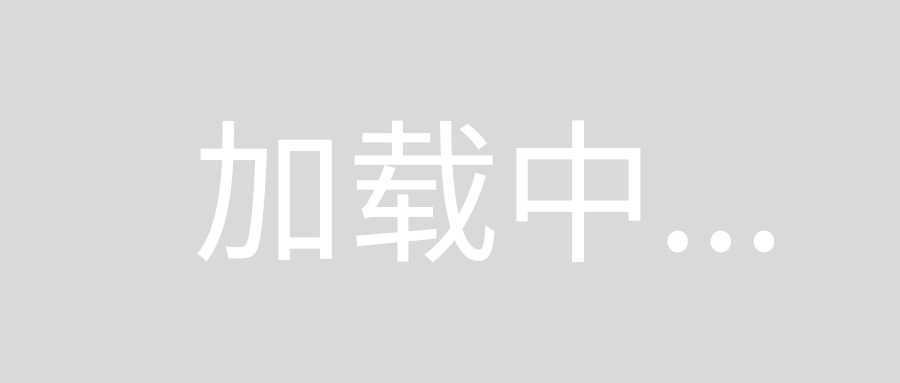