This is beginner question. So please have a patience. I have two view controllers.
First ViewController has two buttons. When the first button is pressed I would like to open TargetViewController with url to Google and when the second button is pressed I would like to open same TargetViewController with url to Yahoo.
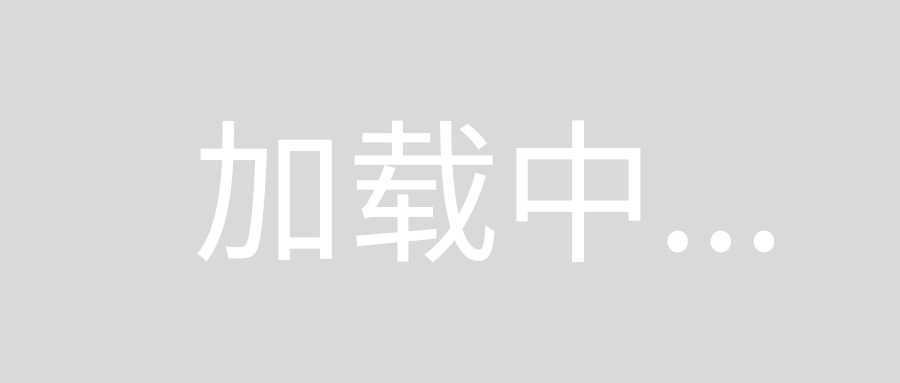
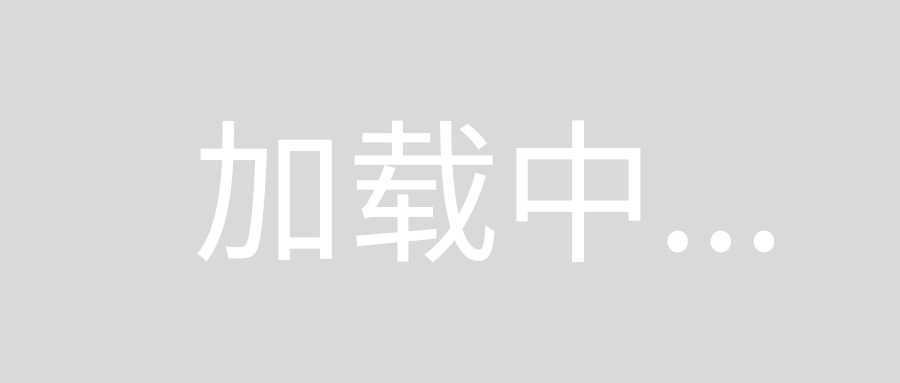
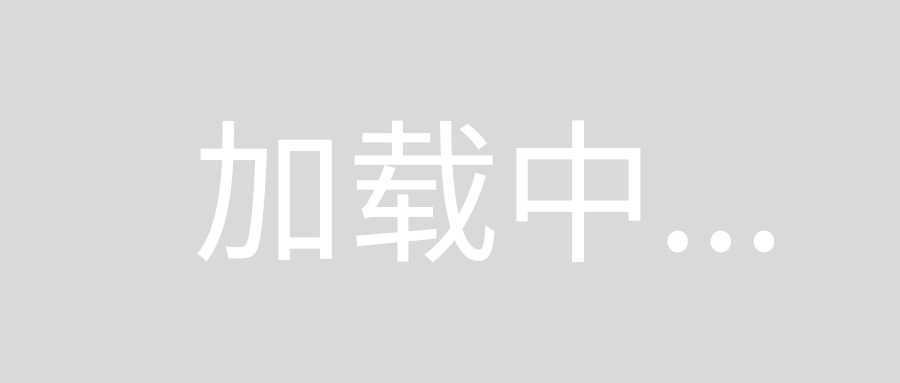
I have create demo project you can download here: https://www.dropbox.com/s/uxjudu1hztyhmk2/Demo.zip?dl=0
If you can help please finish this in simple way with explaining each step you made. It would be a lot of help if you could re upload fished and working example.
I tried previews answers to the same problem/question but they have multiple errors and beginner like me is unable to solved them. Thank you.
You should set segue's identifier in storyboard and implement in viewController:
- (void)prepareForSegue:(UIStoryboardSegue *)segue sender:(id)sender
{
UIViewController* secondController = segue.destinationViewController;
if ([segue.identifier isEqualToString:@"first"])
{
secondController.url = [NSURL URLWithString:@"firstURL"];
}
else
{
secondController.url = [NSURL URLWithString:@"secondURL"];
}
}
I updated your project…
https://www.dropbox.com/sh/csilubh5ztndy0r/AADZ-hygEyNgINyUkod1TRo8a?dl=0
well this is one lengthy answer. But lets see:
First lets name your segues. For that, lets go the storyboard and do click on one of the segue: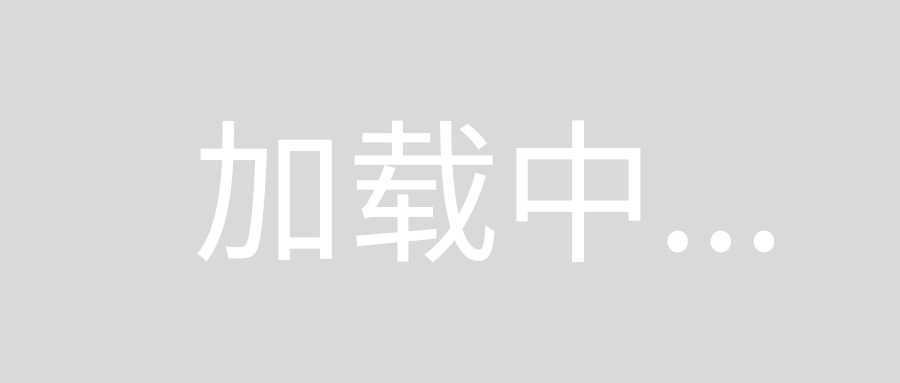
Then you can set the name of the segue on the left panel:
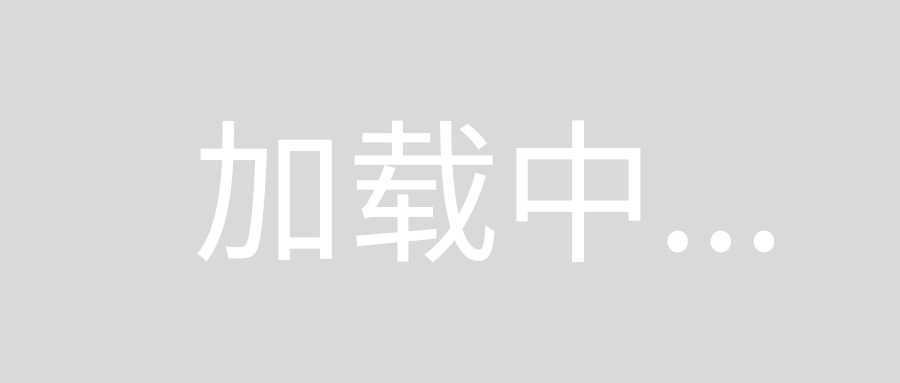
Once this is done, do the same for the second segue.
So now we can identify which segue will be called when you click on the button.
Lets go TargetViewController.h and declare the following property:
@property(strong, nonatomic) NSString * urlString;
Once this is done, lets go to ViewController.m and declare the following method:
- (void)prepareForSegue:(UIStoryboardSegue *)segue sender:(id)sender {
TargetViewController * target = segue.destinationViewController;
if ([segue.identifier isEqualToString:@"GoogleSegueIdentifier"]) {
target.urlString = @"google";
} else if ([segue.identifier isEqualToString:@"YahooSegueIdentifier"]) {
target.urlString = @"yahoo";
}
}
This method will be called, whenever a segue is inisiated on your viewcontroller. So here you can check which segue is called, by referencing the identifier set on your storyboard.
Because you declared this "urlString" variable on TargetViewController, therefore you can set its value here.
After this all you need to do is to add the following in your viewDidLoad @ TargetViewController.m
- (void)viewDidLoad {
[super viewDidLoad];
if([self.urlString isEqualToString:@"google"]){
//open the google URL
} else {
//open yahoo URL
}
}
At the end let me tell you, this is not the best practice what you can follow to get stuff like this done, but this is what you have asked for, so there you go. Hope it helps.
thirdViewController *thirdView = [self.storyboard instantiateViewControllerWithIdentifier:@"third"];
[self.navigationController pushViewController:thirdView animated:YES];
- (IBAction)first_button:(id)sender {
thirdViewController *thirdview = [self.storyboard instantiateViewControllerWithIdentifier:@"third"];
thirdview.url_string=@"first url";
[self.navigationController pushViewController:thirdView animated:YES];
}
- (IBAction)second_button:(id)sender {
thirdViewController *thirdview = [self.storyboard instantiateViewControllerWithIdentifier:@"third"];
thirdview.url_string=@"second url";
[self.navigationController pushViewController:thirdView animated:YES];
}
thirdViewcontroller.h
@property(strong,nonatomic)NSString *url_string;
-(void)viewDidLoad
{
[super viewDidLoad];
NSURL *url = [NSURL URLWithString:url_string];
////////
}
This method have one big plus, there is no need import TargetViewController in First ViewController. Less dependences between classes.
In First ViewController add code:
- (void)prepareForSegue:(UIStoryboardSegue *)segue sender:(id)sender {
if ([sender isKindOfClass:[UIButton class]]) {
NSString *urlString = ((UIButton *)sender).tag == 10 ? @"https://www.google.com" : @"https://www.yahoo.com/";
if ([segue.destinationViewController respondsToSelector:@selector(setUrlString:)]) {
[segue.destinationViewController performSelector:@selector(setUrlString:)
withObject:urlString];
}
}
}
In TargetViewController.h add property:
@property (nonatomic, strong) NSString *UrlString;
After it you could load url in webview, example
Other ways of passing arguments is here.