I want to get posts of an specific post type where those have custom field with type of post type.
I have post type named "tour", and tour post type has custom field named "country" that its type is Post Object.
The query for get all tours that their country is "Turkey":
$located_tours = get_posts(array(
'numberposts' => -1,
'post_type' => 'tour',
'meta_query' => array(
'key' => 'country',
'title' => 'Turkey',
'compare' => 'LIKE'
)
));
But this query return all tours without any filtering on Country
Anyone have any idea?
Thanks
First of all, meta_query
expects nested arrays, so it's not:
'meta_query' => array(
'key' => THE_KEY,
'value' => THE_VALUE,
),
but:
'meta_query' => array(
array(
'key' => THE_KEY,
'value' => THE_VALUE,
),
),
Note that compare
isn't necessary in your case as its default value is =
.
Anyway, you have a conceptual mistake here. If you are using ACF and your country
custom field type is Post Object
, the value for this field stored in the database is not title
but the related post ID
(either your Return Format is Post Object
or Post ID
).
Something like that (in your wp_postmeta
table):
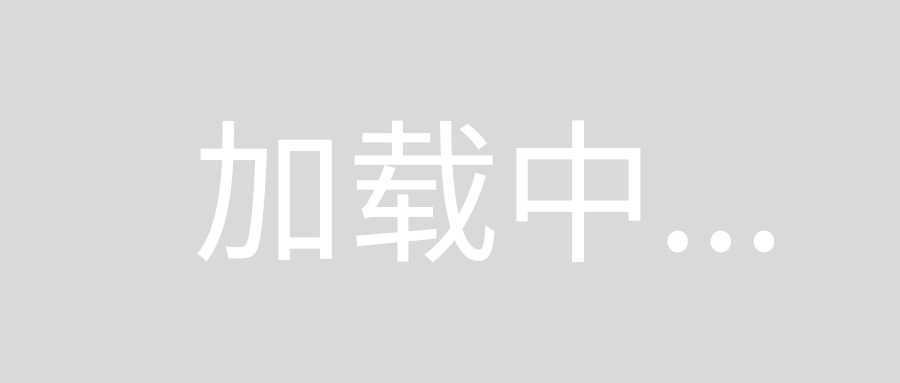
So, you need to query by country's ID
, not country's Title. You could use get_page_by_title
to retrieve your desired country ID:
// Assuming your Country's CPT slug is 'country'
$country = get_page_by_title( 'Turkey', 'OBJECT', 'country' );
$tours_args = array(
'posts_per_page' => -1,
'post_type' => 'tour',
'meta_query' => array(
array(
'key' => 'country',
'value' => $country->ID
)
)
);
$located_tours = get_posts( $tours_args );
Hope this helps!
Try this :
$tour_args = array(
'posts_per_page' => -1,
'post_type' => 'tour',
'meta_query' => array(
array(
'key' => 'country',
'value' => 'Turkey',
'compare' => '=',
),
),
);
$located_tours = new WP_Query($tour_args);