Sometimes when I run python scripts in terminal a python instance opens up
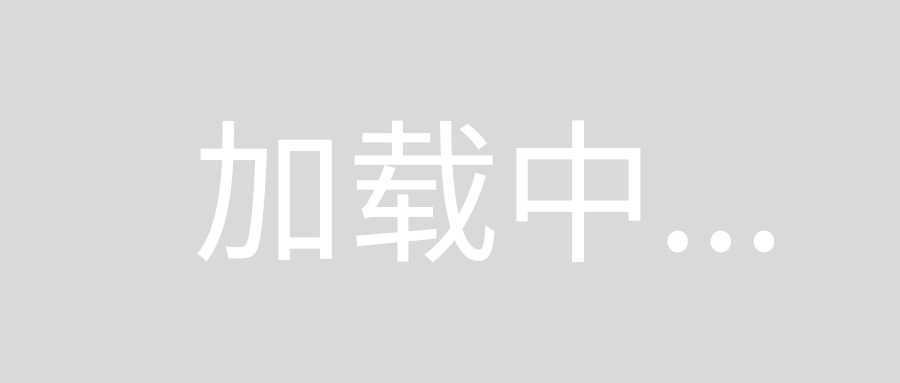
I've seen matplotlib and ghost cause this. The only way to close it seems to be to close the python terminal.
What exactly is this instance and how do I have it close automatically after my script executes?
On OS X the Dock will display an icon for Python whenever you do something that interacts with the GUI. The most common reason for this is using matplotlib
with a GUI backend (e.g. PyQt). When using an interactive backend (e.g. PyQt) you will normally see a rocket icon after you call plt.show()
which will remain on the Dock until after the window is closed and process that interacted with the GUI exits.
When you are working interactively in the Python shell, the icon stays there not because the Terminal is still open (or script is still executing) but because the Python process is still active. This process is probably maintaining a GUI event loop in the background.
There are a few solutions:
- In
matplotlib
you can use plt.show(block=False)
to detach the event loop, meaning the icon will disappear once the window is closed.
- Set a non-interactive backend, e.g.
matplotlib.use("Agg")
to avoid creating the windows at all. Of course, you can't see anything.
- Modify the Python.app
.plist
to suppress icons.
For the latter, the instructions here (Dr. Drang) are as follows. Note this is for the system Python, for other locations you'll need to find the .plist
elsewhere:
cd /System/Library/Frameworks/Python.framework/Versions/2.7/Resources/Python.app/Contents/
# NB the following file is binary, use a compatible editor or convert with
sudo plutil -convert xml1 Info.plist # Convert to XML
sudo chmod 666 Info.plist # Make editable
Then add the following before the final </dict>
<key>LSUIElement</key>
<true/>
Return to binary and fix permissions:
sudo chmod 644 Info.plist
sudo plutil -convert binary1 Info.plist
PyCharm doesn't consider the script to be complete until any popups it spawns are closed. This is evident when you run matplotlib and a plot pops up. If PyCharm closed the window upon completion of the script, the window would immediately close before you could even view your chart.
There are two ways to finish the script. Either close the popups, or click the stop button in PyCharm.