I have a UIScrollview
to allow for pagination, and a UIWebview
on the detail views inside. I know you are not supposed to do that according to Apples Class Reference, but I don't see another option.
In order to make responses and experience a bit better, I disabled scrolling of the UIWebview
, with:
[[[webView subviews] lastObject] setScrollEnabled:FALSE];
But now I am looking for a solution to make the webview the right size, depending on the content. Is there an easy way to do this, where the width of the webview stays the same, but the height grows (automatically) when that is required to show the full article? This will remove the scrolling problem, as it sit he scrollview who will take care of all the scrolling in this scenario.
Does that make sense?
From the documentation for UIWebView, it states:
Important: You should not embed UIWebView or UITableView objects in
UIScrollView objects. If you do so, unexpected behavior can result
because touch events for the two objects can be mixed up and wrongly
handled.
A more appropriate approach would be to add your UIWebView to a UIViewController with a UIScrollView (or UITableView) underneath. I'd be conscious of the amount of space you'll have left though for the scroll view though, especially if you're dealing with the iPhone.
Hope that's a nudge in the right direction.
Update:
After looking into this (I was sure there would be a way), it seems the best approach I managed to come up with is to add your UIWebView to the header view of a UITableView.
//create webview
_webView = [[UIWebView alloc] initWithFrame:CGRectMake(0.0, 0.0, 320.0, 280.0)];
//load request and scale content to fit
[_webView loadRequest:[NSURLRequest requestWithURL:[NSURL URLWithString:@"http://www.stackoverflow.com/"]]];
[_webView setScalesPageToFit:YES];
//add web view to table header view
[[self tableView] setTableHeaderView:_webview];
This adds a UIWebView to the header of the table view before the cells are displayed underneath. Note that a table header view (and footer views also) are different from section headers (and footers) so you can still use these two if you are using UITableViewStylePlain.
Be sure to attenuate the frame of the webview to allow for any cells underneath to be visible or else the user will not be able to scroll the tableview as any touches will be intercepted by the webview.
This still goes against the UIWebView documentation (stated above) but, in practice, this implementation actually works fine as long as the webview does not cover the entire scrollable region of the view.
Here's a screenshot of the test app I made: 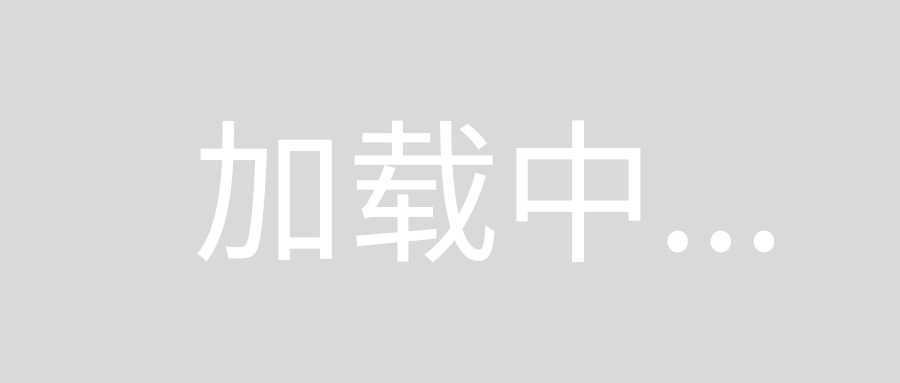
Hope that helps :)
There is a better way to do this.
From iOS 5.0 onwards, apple expose the UIScrollView present inside the UIWebView. So to resize the webview based on the contents, here is what you do.
- Make the parent of UIWebView the delegate to that UIWebView.
Implement the webViewDidFinishLoad: and in that function just do this.
CGSize sz = [webView.scrollView contentSize];
CGRect rect = webView.frame;
rect.size.height = sz.height;
webView.frame = rect;
Even if there are multiple load start and finish, it does not matter since eventually we will have the web view resized to fit the contents.
In case you don't want the user to see the dynamic resizing, you can just set the alpha of webview to 0 and when everything loads and you are done with resizing, you can transition it to alpha 1 with animation.