I'm trying to align the layout so the images align in a row.
Here's a image of what it is currently doing
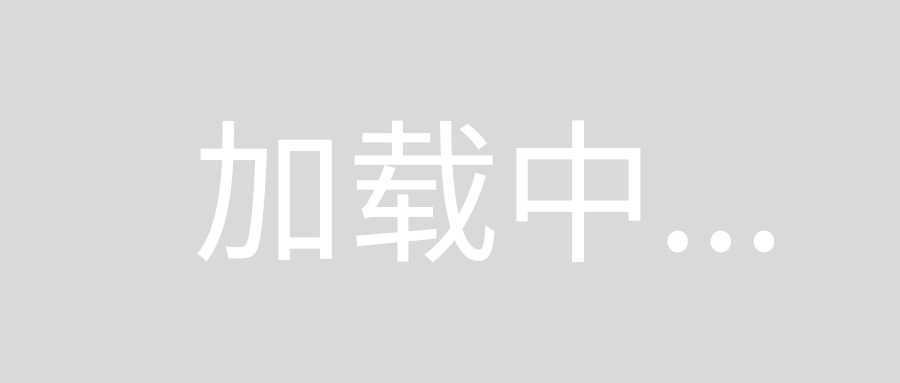
HTML
<div class="p-alignleft"></div>
<div class="p-alignright"></div>
CSS
.p-alignleft {
float: left;
margin-right:40px;
width:450px;
font-size: 1.2em;
line-height: 1.4em;
}
.p-alignright {
float: right;
width:450px;
font-size: 1.2em;
line-height: 1.4em;
}
If I understand rightly, you have a couple of options here. Instead of floating, my preference is to set each div to display: inline-block; That will make the divs line up next to each other, even if one is taller than the other:
div {
display: inline-block;
vertical-align: top;
}
A working example: http://cdpn.io/ojDEl
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<style media="all">
.wrap {width: 800px;}
.wrap div {width: 48%; display: inline-block; vertical-align: top; background: #e7e7e7; margin-bottom: 20px;}
/* temp demo styles */
.wrap div {height: 200px;}
.wrap div.test {height: 300px;}
</style>
</head>
<body>
<div class="wrap">
<div>Person A</div>
<div class="test">Person B</div>
<div>Person C</div>
<div>Person D</div>
</div>
</body>
</html>
By looking at the captured screen, I think you should enclose each person's part inside a div
, and give them classes .p-alignleft
or .p-alignright
. After every two of them, make an empty <div class="clear"></div>
with style .clear {clear:both}
, so the next two persons will align at the same vertical level
HTML:
<div class="p-alignleft">Person A</div>
<div class="p-alignright">Person B</div>
<div class="clear"></div>
<div class="p-alignleft">Person C</div>
<div class="p-alignright">Person D</div>
CSS:
.p-alignleft {float:left}
.p-alignright {float:right}
.clear {clear:both}
- Use a container div as a row element
<div class="row clearfix"><div class="media">...</div></div>
- Float both elements to the left, and set
clear: left
on the odd ones.
- Use a javascript solution to set the height to be the same. then you can leave the clear left, right, or clear all to one side.
something like this, you probably need to tweak it, it's more like pseudo code.:
var maxHeight = 0;
var items = $('.media');
// get the max height of the items
items.each(function() {
var height = parseInt($(this).outerHeight().replace('px', ''), 10);
if (maxHeight < height) {
height = maxHeight;
}
});
// assign the height to all the items
items.height(height + 'px');