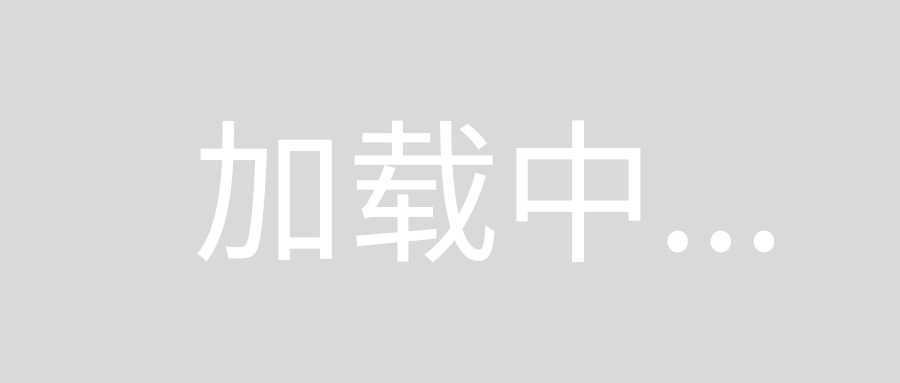
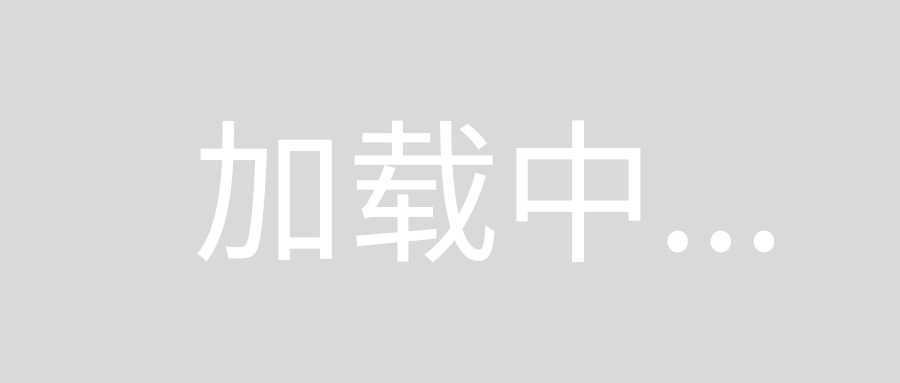
Is there a way to fuse the Jpeg image with the correspondant pixel temperature shown in the stringgrid attached.
After the fusion, mouse arround and read the temperatures from the image.
If there is, please can anyone show me how.
Thank you, AmmadeuX
What I understand is that you have three different questions at hand. I will point them out below, along with an answer. But please, you need to do your best to get these types of projects done yourself, and only ask questions here if you have a specific issue which needs to be resolved. It's always subjective to ask "How to do something".
Questions 2 and 3 are either-or solutions, meaning each one could be a different question and answer all-together, completely separate. But they both still depend on the first question.
Question 1: "How do I identify the color of a pixel which the mouse is pointed over?"
I am assuming you're using a standard TImage
control with its default properties, and is not set to re-size, stretch, or center the image. If you need to do that, there's a different approach.
First, you have to identify which pixel the mouse is pointed over. There are a few ways of doing this, but depending on what control you are using to display the image (I'm assuming a standard TImage
), I would recommend using the Mouse Move event (OnMouseMove
) to capture movement of the mouse over this control. At this point, you need to identify the current mouse position. Thankfully, this event comes with the X and Y position of the mouse pointer.
Then, you need to get the canvas of the image. You can get this with:
TImage.Picture.Bitmap.Canvas
Next, read the Canvas' Pixels[]
property to get the color.
Question 2: "How do I identify where a color falls on a From/To scale?"
Now that you know what color the mouse is pointed over, you need to identify in which position of your scale this color belongs. For this, it's easiest to stick with only 1 color channel. Your example above illustrates a combination and fade of different colors, which is way too tricky for me to try to explain. Please ask this as an additional question, because it requires its own thought which I cannot do (requires extensive math which I'm not good at).
Question 3: "How do I link an image to a table of data?"
Since you have a table of temperature readings, you need to read this table by column/row based on the individual pixel provided in the first question above.
Since you already know the X/Y position of the mouse, use these same variables to identify the column/row of the grid. (I discourage using a grid as your primary base of storing this data, you should use a client-dataset instead, and dynamically load the grid with that data.)
Conclusion:
Here's the code of a sample app I prepared for you. It should help you understand the basics behind what you're doing. You'll have to pick your own image, as I didn't want to pollute this answer's code with raw image data.
NOTE: Once you edit your question to be clear as to how you expect the image to be linked to the data, then I will modify my answer. Otherwise, this is the best I can explain in its current state.
Unit1.pas
unit Unit1;
interface
uses
Winapi.Windows, Winapi.Messages, System.SysUtils, System.Variants, System.Classes, Vcl.Graphics,
Vcl.Controls, Vcl.Forms, Vcl.Dialogs, Vcl.ExtCtrls, Vcl.StdCtrls, Vcl.ComCtrls;
type
TForm1 = class(TForm)
Image1: TImage;
Shape1: TShape;
StatusBar1: TStatusBar;
procedure Image1MouseMove(Sender: TObject; Shift: TShiftState; X,
Y: Integer);
end;
var
Form1: TForm1;
implementation
{$R *.dfm}
procedure TForm1.Image1MouseMove(Sender: TObject; Shift: TShiftState;
X, Y: Integer);
var
Color: TColor;
Canvas: TCanvas;
R, G, B: Byte;
begin
Canvas:= Image1.Picture.Bitmap.Canvas;
Color:= Canvas.Pixels[X, Y];
Shape1.Brush.Color:= Color;
R:= GetRValue(Color);
G:= GetGValue(Color);
B:= GetBValue(Color);
StatusBar1.Panels[0].Text:= 'Pos: '+IntToStr(X)+' x '+IntToStr(Y);
StatusBar1.Panels[1].Text:= 'Clr: '+IntToStr(Color);
StatusBar1.Panels[2].Text:= 'RGB: '+IntToStr(R)+' x '+IntToStr(G)+' x '+IntToStr(B);
end;
end.
Unit1.dfm
object Form1: TForm1
Left = 315
Top = 113
Caption = 'Form1'
ClientHeight = 415
ClientWidth = 548
Color = clBtnFace
Font.Charset = DEFAULT_CHARSET
Font.Color = clWindowText
Font.Height = -11
Font.Name = 'Tahoma'
Font.Style = []
OldCreateOrder = False
DesignSize = (
548
415)
PixelsPerInch = 96
TextHeight = 13
object Image1: TImage
Left = 0
Top = 0
Width = 548
Height = 281
Align = alTop
Anchors = [akLeft, akTop, akRight, akBottom]
OnClick = Image1Click
OnMouseMove = Image1MouseMove
end
object Label1: TLabel
Left = 8
Top = 285
Width = 31
Height = 13
Anchors = [akLeft, akBottom]
Caption = 'Label1'
end
object Shape1: TShape
Left = 8
Top = 312
Width = 532
Height = 57
Anchors = [akLeft, akRight, akBottom]
end
object StatusBar1: TStatusBar
Left = 0
Top = 396
Width = 548
Height = 19
Panels = <
item
Width = 150
end
item
Width = 150
end
item
Width = 50
end>
end
end