So I've checked out the other post which didn't help on here, I'm trying to get my frame with it's message to randomly appear on an area on the screen but when I run it, it says x and y cannot be resolved to a variable, here's the code:
public class MyFrame extends JFrame{
MyFrame(int width, int height, int x, int y){
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setTitle("R and Ts Main Frame");
setSize(width, height);
Random random = new Random();
x = random.nextInt();
y = random.nextInt();
setLocation(x, y);
JLabel label = new JLabel("Random Message");
label.setFont(new Font("Impact", Font.BOLD|Font.PLAIN, height/3));
label.setForeground(Color.BLUE);
getContentPane().add(label);
}
}
and this is my main:
public class OurMain {
public static void main(String[] args) {
Dimension sSize = Toolkit.getDefaultToolkit().getScreenSize();
int w = sSize.width;
JFrame f = new MyFrame(w/3, 100, x, y); //my errors are underlined here under the x and y
f.setVisible(true);
}
}
You should just add some attributes and replace some things:
MyFrame:
public class MyFrame extends JFrame {
int x;
int y;
MyFrame(int width, int height, int x, int y) {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setTitle("R and Ts Main Frame");
setSize(width, height);
this.x = x;
this.y = y;
setLocation(x, y);
JLabel label = new JLabel("Random Message");
label.setFont(new Font("Impact", Font.BOLD | Font.PLAIN, height / 3));
label.setForeground(Color.BLUE);
getContentPane().add(label);
}
}
OurMain:
public class OurMain {
public static void main(String[] args) {
Dimension sSize = Toolkit.getDefaultToolkit().getScreenSize();
int w = sSize.width;
Random random = new Random();
int x = random.nextInt();
int y = random.nextInt();
JFrame f = new MyFrame(w / 3, 100, x, y); //my errors are underlined here under the x and y
f.setVisible(true);
}
}
EDIT:
However, random.nextInt();
is not a good idea, because your screen width&heigth has a little less then 2^32 pixels... so I would limit it to e.g.:
public static void main(String[] args) {
Dimension sSize = Toolkit.getDefaultToolkit().getScreenSize();
int w = sSize.width;
int h = sSize.height;
int x = (int)((Math.random()* w) - w/3);
int y = (int)((Math.random()* h) - 100);
System.out.println(x + " " + y);
JFrame f = new MyFrame(w / 3, 100, x, y);
f.setVisible(true);
}
EDIT of EDIT:
public class OurMain {
public static void main(String[] args) {
Dimension sSize = Toolkit.getDefaultToolkit().getScreenSize();
int w = sSize.width;
int h = sSize.height;
Random rand1 = new Random();
int randomWidth = rand1.nextInt(w);
int randomHeight = rand1.nextInt(h);
Random rand2 = new Random(); // I would still use rand1
int randomX = rand2.nextInt(w - randomWidth + 1);
int randomY = rand2.nextInt(h - randomHeight + 1);
JFrame f = new MyFrame(randomWidth, randomHeight, randomX, randomY);
f.setVisible(true);
}
}
This will solve your problem. You did not declare/create x
and y
before using them, but you don't need them, so just use them locally.
public class OurMain {
public static void main(String[] args) {
Dimension sSize = Toolkit.getDefaultToolkit().getScreenSize();
int w = sSize.width;
Random random = new Random();
int x = random.nextInt(sSize.width);
int y = random.nextInt(sSize.height);
JFrame f = new MyFrame(w/3, 100, x, y);
}
}
public class MyFrame extends JFrame {
private static final long serialVersionUID = 1L;
public MyFrame(int width, int height, int x, int y) {
super();
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setTitle("R and Ts Main Frame");
setSize(width, height);
setLocation(x, y);
JLabel label = new JLabel("Random Message");
label.setFont(new Font("Impact", Font.BOLD | Font.PLAIN, height / 3));
label.setForeground(Color.BLUE);
getContentPane().add(label);
setVisible(true);
}
}
Result (it is loaded in a different location each time):
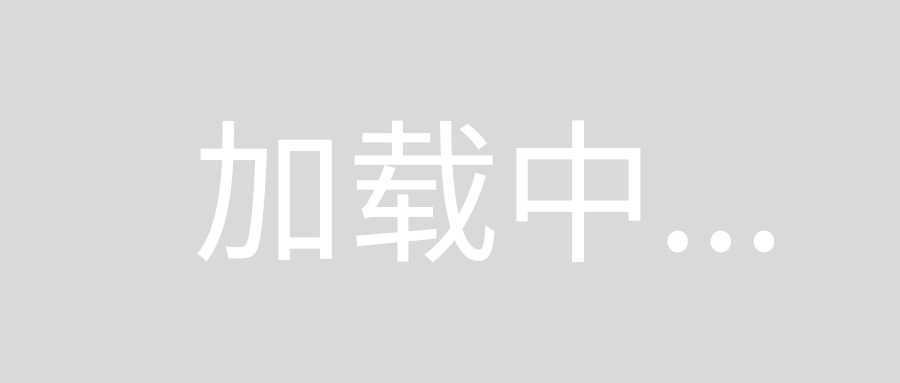