As the title suggests, I want to put some text in the action bar. Firstly, here's a mockup (in Paint!) of what I'm imagining:
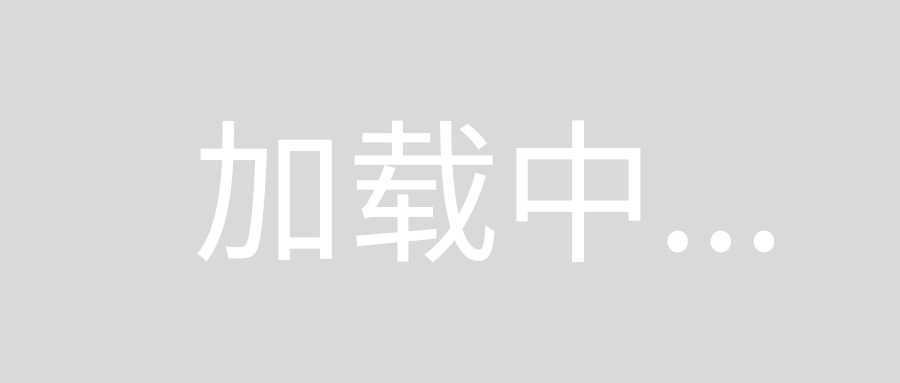
I'm really struggling with this. In my activity I put:
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.main_screen, menu);
return true;
}
And in the menu folder I put main_screen.xml:
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:android="http://schemas.android.com/apk/res/android" >
<item android:id="@+id/user_level"
android:title="Administrator"
android:showAsAction="withText" />
</menu>
So I have 2 questions:
Question 1
Why at the very least the solution above doesn't show the text Administrator
Question 2
Once it's showing the text, how do I make it appear like a label (with the grey background and the rounded corners, etc)?
Thank you for your help.
custom menu item is what you need for your second question.
first you need to make custom layout for your menu item.
cust_menu.xml
<TextView
android:id="@+id/admin"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dp"
android:text="Administrator"
android:textColor="#fff"
android:background="@drawable/my_shape";
android:textStyle="bold" />
for making rounded grey square background you need to make custom shape file and set it to your TextView
background.
my_shape.xml
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="rectangle" >
<corners android:radius="3dp" />
<solid android:color="#e3e3e3" />
</shape>
now your menu item will go like this.
main.xml
<item
android:id="@+id/admin"
android:actionLayout="@layout/cust_menu"
app:showAsAction="always">
</item>
and in java class simply do this.
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
let me know if any doubts.
for more information visit this
EDIT :
you can access your TextView
this way.
@Override
public boolean onCreateOptionsMenu(Menu menu) {
MenuInflater inflater = getSupportMenuInflater();
inflater.inflate(R.menu.main, menu);
View view = menu.findItem(R.id.admin).getActionView();
TextView textView = (TextView) view.findViewById(R.id.textID);
// do your stuff
return super.onCreateOptionsMenu(menu);
}
happy coding.
You can try adding below code in your menu item:
android:showAsAction="always|withText"
android:orderInCategory="50"
50
is just a random number.
You can configure the ActionBar styles and properties by creating ActionBar theme styles.
Read more at:
Custom ActionBar Styles
Question 1
You have to use the app namespace.
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto">
<item android:id="@+id/user_level"
android:title="Administrator"
app:showAsAction="withText" />
</menu>
Question 2
You cannot use menu to do that. What you can do is to use AppBarLayout
.
<android.support.design.widget.CoordinatorLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<android.support.design.widget.AppBarLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:theme="@style/ThemeOverlay.AppCompat.Dark.ActionBar">
<android.support.v7.widget.Toolbar
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize">
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center_vertical|end"
android:paddingEnd="15dp"
android:paddingLeft="15dp"
android:paddingRight="15dp"
android:paddingStart="15dp"/>
</android.support.v7.widget.Toolbar>
</android.support.design.widget.AppBarLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<!--Your normal content-->
</LinearLayout>
</android.support.design.widget.CoordinatorLayout>