I want to add a save button
in action bar but it shows below the action bar
.
What is the correct to solve ? Any help would be greatly appreciated.
Code
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical" android:layout_width="match_parent"
android:layout_height="match_parent">
<android.support.v7.widget.Toolbar
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@color/black"
android:theme="@style/Theme.AppCompat"
android:minHeight="?attr/actionBarSize">
<LinearLayout
android:layout_width="wrap_content"
android:layout_gravity="right"
android:layout_height="match_parent">
<Button
android:id="@+id/save"
android:text="SAVE"
android:layout_width="wrap_content"
android:background="#000"
android:textColor="#fff"
android:layout_height="wrap_content"
android:textSize="16dp" />
</LinearLayout>
</android.support.v7.widget.Toolbar>
<ImageView
android:layout_marginLeft="50dp"
android:layout_marginTop="20dp"
android:background = "@drawable/img_receipt"
android:src="@drawable/upload"
android:scaleType="fitXY"
android:layout_width="270dp"
android:layout_height="290dp"
android:id="@+id/image" />
<TextView
android:layout_marginLeft="15dp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textAppearance="?android:attr/textAppearanceMedium"
android:text="Add a description"
android:textColor="@color/black"
android:textStyle="bold"
android:layout_marginTop="40dp"
android:id="@+id/textView"
android:layout_below="@+id/image"
/>
<EditText
android:layout_marginLeft="5dp"
android:layout_width="350dp"
android:paddingBottom="75dp"
android:paddingLeft="10dp"
android:layout_height="120dp"
android:hint="Add a description"
android:textColor="@color/black"
android:background="@drawable/roundedcorner_edittext"
android:layout_marginTop="80dp"
android:layout_below="@+id/image"
android:id="@+id/editText" />
</RelativeLayout>
Screen Shot
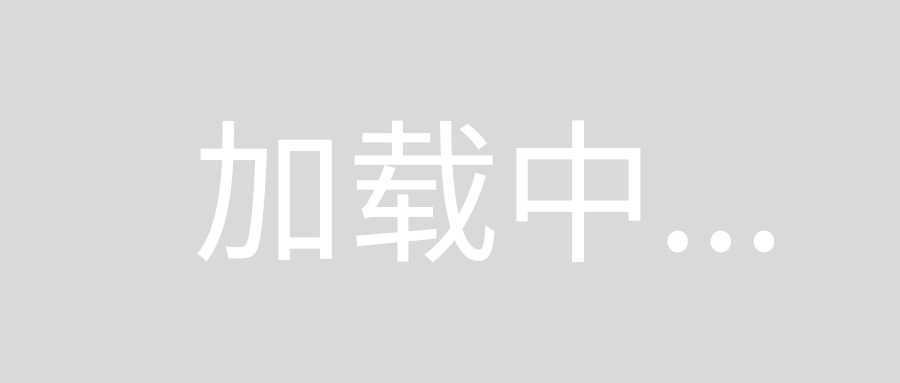
As you can see the save button is placed below action bar. I want it place beside the healthy app title.
You can add button on action bar like that...
Remove toolbar(if you are using only to add save button on action bar)
create the menu_main xml file:
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto">
<item
android:id="@+id/action_save"
android:icon="@drawable/you_resourse_here"
android:title="Save"
app:showAsAction="always"
android:orderInCategory="0"/>
</menu>
Then in your activity, if you created a new file your need to edit onCreateOptionsMenu
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_main, menu);
return true;
}
and you can edit what the actions do in the following method:
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
if (id == R.id.action_save) {
do something when you click on save button
return true;
}
return super.onOptionsItemSelected(item);
}
Hope this will help you.
Finally I get it works
In my Receipt Activity, add these two lines
Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
and add this lines in androidMainFest to avoid the app cras
<activity android:name=".Receipt"
android:theme="@style/Theme.AppCompat.Light.NoActionBar">
</activity>
// Initially, make an XML file inside your layout folder say "toolbar.xml"
<?xml version="1.0" encoding="utf-8"?>
<android.support.v7.widget.Toolbar
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@color/lightblue"
app:theme="@style/ToolbarColoredBackArrow"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:android="http://schemas.android.com/apk/res/android" >
</android.support.v7.widget.Toolbar>
// And inside any layout file where you want to use toolbar jsut do following
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.limatrder.activitis.Bill_details">
<FrameLayout
android:id="@+id/framelayout"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<include layout="@layout/toolbar" />
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center">
<ImageView
android:id="@+id/imgback_billDetail"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_centerInParent="true"
android:padding="15dp"
android:src="@drawable/action_bar_cross" />
<TextView
android:id="@+id/txtviewcart_billdetais"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:layout_marginLeft="20dp"
android:padding="15dp"
android:text="View Cart"
android:textColor="@color/white"
android:textSize="18dp" />
</RelativeLayout>
</FrameLayout>
<!-- here you can write your code for remaining design-->
<LinearLayout
android:orientation="vertical"
android:layout_below="@+id/framelayout"
android:background="@color/lightblue"
android:layout_width="wrap_content"
android:layout_height="wrap_content">
</LinearLayout>
</RelativeLayout>
// and in yor style.xml use
Theme.AppCompat.Light.NoActionBar